What is Dependency Injection and Why Use It?
Engineering
Object Oriented Programming
Dependency Injection
Summary
Dependency Injection (DI) is a design pattern that decouples object creation from its dependencies, encouraging more modular, maintainable, and testable code. DI improves system flexibility by allowing dependencies to be injected externally rather than instantiated within a class. With various forms like Constructor, Setter, and Interface Injection, DI ensures easier unit testing and adherence to design principles like SOLID.
Key insights:
Loose Coupling: DI minimizes direct dependency between classes, enhancing modularity.
Flexibility: Makes it easier to swap out components without altering core code.
Testability: Dependencies can be mocked for isolated unit tests.
SOLID Principles: DI supports Single Responsibility and Open/Closed principles.
Multiple Injection Types: Constructor, Setter, and Interface Injection offer flexibility for different use cases.
Introduction
In modern software engineering, writing maintainable and testable code is a top priority. One of the key design patterns that helps achieve this is Dependency Injection (DI). At its core, DI is a method for managing how different parts of a system communicate and depend on each other.
By adopting Dependency Injection, developers can create systems that are easier to extend, modify, and test. This article explores DI from a computer science perspective, discussing its types, benefits, best practices, and common mistakes, as well as examples in Dart to help readers understand why DI is considered a strong tool for building high-quality production-level code.
Dependency Injection: What It Is, Importance, and History
Dependency Injection (DI) is a design pattern used to achieve loose coupling (classes should have minimal knowledge of other classes and depend only on their behavior rather than their specific implementation) between classes or components in a system. The core idea of DI lies in separating the creation of an object’s dependencies from the object itself, allowing the system to be more modular and flexible. Before diving deeper into DI, it is important to understand the concept of dependency.
1. What are Dependencies?
A dependency is any object or component that another object relies on to perform its task. For a better understanding, let us take a look at an example. Consider a scenario where we have a PaymentProcessor class.
This class (shown below) relies on external services such as PaymentGateway to handle transactions and NotificationService to send receipts or confirmations, which are its dependencies. Without Dependency Injection, the PaymentProcessor would create these dependencies itself, leading to tight coupling.
In this example, the PaymentProcessor directly depends on the specific implementations of PaymentGateway and NotificationService.
Now, consider the following scenario: what if we want to switch to a new payment gateway or notification service? In this case, the PaymentProcessor class must be modified which violates the Open/Closed Principle - a key software design concept that states that classes should be open for extension but closed for modification (meaning you can extend a class’s behavior without altering its existing code). Moreover, testing this class becomes challenging because it always creates real instances of its dependencies, making it difficult to mock or replace them during testing.
The tight coupling in the code above reduces the flexibility and maintainability of the code. Dependency Injection offers a solution to these problems by decoupling the class from its dependencies, allowing for easier testing and adherence to SOLID design principles.
2. Introducing Dependency Injection
With Dependency Injection, instead of having a class instantiate its dependencies, those dependencies are provided (or injected) to the class externally. This is typically achieved by passing the required dependencies via the constructor or through setters. This design pattern decoupled the class from specific implementations, making the system more modular and easier to maintain.
Here is the same class provided above, but with constructor injection which helps us to decouple the class from its dependencies and improve maintainability:
In this version, the PaymentProcessor class no longer creates the PaymentGateway or NotificationService. Instead, it receives them as parameters, decoupling the class from specific implementation. Now, the class is flexible and can work with any PaymentGateway or NotificationService provided to it. This allows for easy swapping of implementations as shown below:
Furthermore, this approach simplifies testing, as mock objects or alternative implementations can be injected without modifying the PaymentProcessor itself - following the Open/Closed Principle.
3. Importance of Dependency Injection
Dependency Injection provides several advantages. These include:
Flexibility and Extensibility: By decoupling a class from its dependencies, DI allows you to change or extend the system behavior easily. For example, if the system needs to switch to a new payment gateway, you can simply inject the new PaymentGateway implementation, without modifying the core business logic of PaymentProcessor.
Testability: A major advantage of DI is its impact on testability. Since dependencies are injected, it is easy to substitute real dependencies with mock versions during unit tests. This enables you to test each class in isolation, ensuring that you verify the behavior of the class without relying on the actual implementation of its dependencies. In the example provided above, you could easily mock the PaymentGateway and NotificationService to test how PaymentProcessor behaves under different conditions, without making real API calls. Here is an example of how one might mock a class during testing:
SOLID Principles: SOLID, introduced by Robert C. Martin (Uncle Bob), is a set of five design principles in object-oriented programming aimed at making software designs more understandable, flexible, and maintainable. The acronym stands for Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion principles. DI helps enforce the Single Responsibility Principle by ensuring that a class only focuses on its tasks and does not manage the lifecycle of its dependencies. It also supports the Open/Closed Principle as discussed previously.
4. Historical Context of Dependency Injection
The concept of Dependency Injection is a specific application of a broader design principle known as Inversion of Control (IoC). In traditional programming or naive applications, classes control their dependencies by creating them directly. With IoC, this control is inverted: an external entity provides the dependencies to the class. DI is one form of IoC, where the external source is responsible for injecting the dependencies.
Today, DI is a common practice across various programming languages and frameworks, including mobile development frameworks like Flutter in Dart. Modern development environments often provide built-in support for DI through specialized libraries or frameworks (e.g., GetIt for Dart), which facilitate the implementation of dependency injection.
Types of Dependency Injections
There are several ways to implement Dependency Injection, each with its advantages and use cases. The most common forms are Constructor Injection, Setter Injection, and Interface Injection. These methods differ in how dependencies are supplied to the class.
1. Constructor Injection
In Constructor Injection, as explained in its name, dependencies are provided to the class via its constructor at the time of instantiation. This ensures that the required dependencies are always available as the object cannot be created without them. Constructor Injection makes it easy to follow best practices such as immutability, as dependencies are set once and cannot be changed afterward. Here is a simplified example in Dart:
In this example, the PaymentProcessor requires a PaymentGateway to function. By injecting the PaymentGateway through the constructor, we ensure the class has the necessary dependency to work correctly. This pattern is particularly useful when a class cannot operate without specific dependencies, making it clear at the time of object creation.
2. Setter Injection
Setter Injection allows dependencies to be provided after the object has been instantiated, using setter methods. This approach is more flexible than constructor injection since it enables dependencies to be modified or replaced at runtime.
However, it also introduces some risks. Since a class may be created without it being immediately available, it can lead to potential runtime errors if those dependencies are not set properly before they are used.
Here is an example of a Setter Injection in Dart:
Here, the PaymentProcessor can operate without the NotificationService initially. The notification service is injected using a setter method, and the class can function as long as the service is set before it is needed. This method is beneficial when the dependency is optional or when it can change over the lifecycle of the object.
3. Interface Injection
Interface Injection is commonly used in complex systems and is recommended. In this approach, the class expects its dependencies to be provided through an interface.
An interface in object-oriented programming defines a contract that a class must follow. It specifies a set of methods that must be implemented by any class that implements the interface but does not define how those methods are executed.
This technique allows for a high level of abstraction and decoupling. The class using the interface can then rely on the expected behavior without needing to know the details of how it is implemented.
Here is an example in Dart to help you better understand the concept:
In this example, the LogService interface defines a contract that any class implementing it must follow, specifically the log() method. There are two different implementations of this interface: FileLogService, which logs messages to a file, and DatabaseLogService, which logs messages to a database. The PaymentProcessor class does not need to know whether it is using a file or database logger - it only knows that it can call the log() method of LogService.
Additionally, as seen in the example above, Interface Injection works in combination with either Constructor or Setter Injection. The idea of having the class depend on an interface rather than a specific class is the core concept of Interface Injection - we would still need to somehow inject the interface into the class.
Each type of Dependency Injection has its ideal use case, and developers must choose the right one depending on the needs of the application. Constructor Injection is usually preferred for its simplicity and strong guarantees, while Setter and Interface Injection can be used when more flexibility is required.
Best Practices for Implementing Dependency Injection
When implementing Dependency Injection, following established best practices is important to ensure that your system remains maintainable, scalable, and flexible. By carefully managing dependencies, developers can avoid common mistakes while maximizing the benefits of DI. In this section, we explore key principles that should be followed when using DI.
1. Depend on Abstractions, Not Implementations
A core principle of Dependency Injection is promoting loose coupling between system components. By injecting dependencies through interfaces or abstract classes, components can operate independently of the concrete classes used to perform specific tasks.
For example, if PaymentProcessor relies on the LogService interface rather than a specific logging class, you can switch between logging to a file, database, or external service without needing to modify the PaymentProcessor class. This promotes system flexibility and allows for easier changes down the line.
2. Use Constructor Injection for Essential Dependencies
Constructor Injection should be the preferred method when dealing with required dependencies. By required dependencies, we refer to those that the class cannot function without. Constructor Injection involves passing all the required dependencies through the class constructor when the object is created, ensuring that the class always has everything it needs to operate correctly.
For example, consider a PaymentProcessor class that relies on a PaymentGateway to handle transactions. By injecting the PaymentGateway via the constructor, the PaymentProcessor cannot be created without a valid gateway, which guarantees that the necessary functionality is always available. This avoids the risk of runtime errors caused by missing dependencies.
3. Leverage DI Frameworks for Object Creation
In complex systems, manually managing dependency injection can be difficult, especially when dealing with numerous classes and dependencies. To handle this efficiently, it is often recommended to use a Dependency Injection framework which is responsible for managing the creation, configuration, and life cycle of objects.
For example, in Dart, we can use the GetIt library to act as a service locator and DI container, managing the injection of services and dependencies across the application:
4. Minimize the Use of DI in Simple Use Cases
While dependency injection is a valuable pattern, it should not be applied in every scenario. For simple classes or objects that do not have complex dependencies or lifecycles, it is often better to instantiate them directly.
Overusing DI can lead to unnecessary complexity and make the system harder to understand and maintain. DI should be reserved for objects whose dependencies may change over time or that are shared across different parts of the system.
5. Beware of Circular Dependencies
Circular dependencies occur when two or more classes depend on each other. This is a problematic pattern because it can lead to runtime errors and difficulty resolving dependencies. To avoid circular dependencies, the relationships between components should be carefully designed.
For example, if class A depends on class B, and class B depends on class A, both classes cannot be instantiated without the other, causing a deadlock.
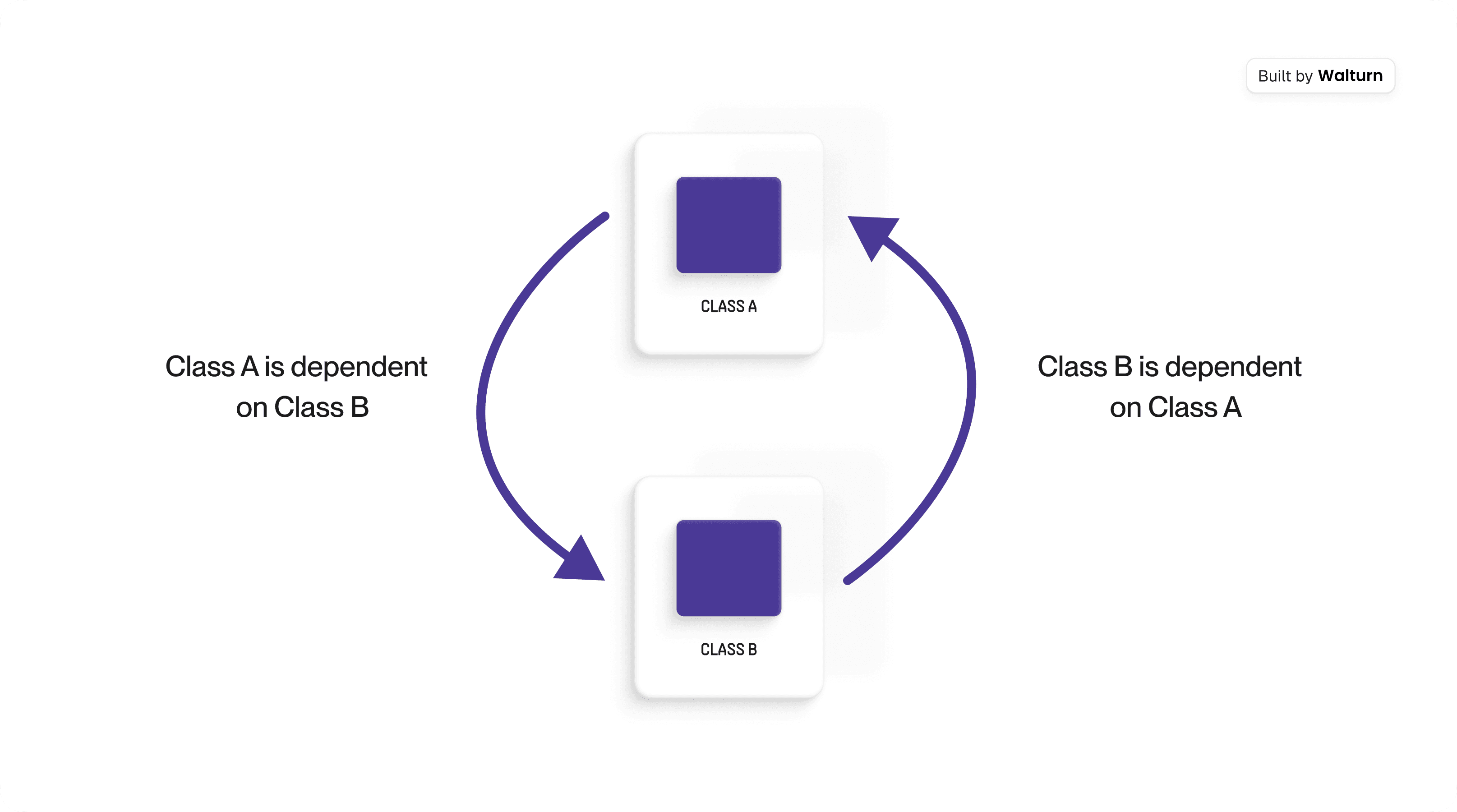
To prevent this, you can break the dependency cycle by introducing an intermediary class or refactoring the system to reduce dependencies between the components. Another option is to introduce more abstraction, such as having both classes rely on an interface that does not create a direct loop.
6. Take Advantage of Dependency Injection for Unit Testing
One of the advantages of DI is its ability to simplify unit testing. When classes receive their dependencies through DI, it becomes easy to replace those dependencies with mock objects in testing environments. This allows developers to test classes in isolation, without invoking real services or external dependencies.
Consider a PaymentProcessor that depends on a PaymentGateway:
In production, you would use a RealPaymentGateway that interacts with an actual payment service. However, for unit testing, you can inject a MockPaymentGateway to simulate various scenarios:
This approach enhances test reliability and efficiency by isolating the logic being tested from real-world data or APIs. You can simulate successful transactions, failed payments, and other scenarios without relying on external services. By using dependency injection in this manner, you can write comprehensive unit tests that cover various conditions, improving the quality of your tests and leading to more modular, maintainable code.
7. Consider Dependency Lifecycles
When using DI, especially with libraries like GetIt, it is important to understand and manage the lifecycle of your objects. A common mistake is failing to distinguish between objects that should be singletons (one instance shared throughout the application) and objects that should be transients (a new instance created fresh every time they are needed). Mismanaging object life cycles can result in unexpected behavior, such as memory leaks or performance bottlenecks.
For example, registering a service as a singleton when it needs to be unique per request or operation can lead to an unwanted shared state. On the other hand, creating a new instance of a service every time it is needed when a singleton would be more appropriate, can cause unnecessary memory consumption and slower performance. To avoid this, carefully consider whether each service should be singleton or transient.
In Dart, using GetIt, you can easily control this:
8. Leverage Lazy Initialization of Dependencies
In some cases, dependencies may be expensive to create or may not be needed immediately when a class is instantiated. Lazily initialization is a technique where the creation of a dependency is deferred until it is required. This can optimize the performance of your application by avoiding unnecessary object creation.
In Dart, GetIt supports lazy initialization with the registerLazySingleton() method, which creates the singleton instance only when it is accessed for the first time:
Lazy initialization can be useful for improving the startup time of your application and reducing memory consumption by deferring the creation of resources until they are truly necessary.
9. Monitor and Manage Performance
In complex applications, it is important to keep an eye on performance when using DI. Injecting too many dependencies into a class can make it harder to maintain and slower. To mitigate this, ensure that dependencies are injected only where needed. You should also review the performance of your application to identify bottlenecks.
Conclusion
In conclusion, Dependency Injection (DI) is a useful design pattern that can greatly enhance the flexibility, scalability, and testability of applications as they grow in complexity. Throughout this article, we have explored the foundations of DI, different methods of implementation, and best practices. By following best practices and avoiding common mistakes, developers can effectively use DI to build well-designed applications.
Authors
Improve Your Code Quality with Walturn’s Expertise
At Walturn, we specialize in writing high-quality, scalable, and maintainable code tailored to your project’s needs. Our solutions are designed to ensure code reusability and flexibility, allowing your software to grow and adapt seamlessly. Partner with us to build efficient systems that stand the test of time.
References
Adiassa, Ethiel. “Always Depend on Abstractions, a Dart Example.” DEV Community, 19 Jan. 2024, dev.to/ethiel97/always-depend-on-abstractions-a-dart-example-1ik0
Chris, Kolade. “Open-Closed Principle – SOLID Architecture Concept Explained.” freeCodeCamp.org, 22 Feb. 2023, www.freecodecamp.org/news/open-closed-principle-solid-architecture-concept-explained.
CodeAesthetic. “Dependency Injection, the Best Pattern.” YouTube, 4 Aug. 2023, www.youtube.com/watch?v=J1f5b4vcxCQ.
Fowler, Martin. “Inversion of Control Containers and the Dependency Injection Pattern.” martinfowler.com, martinfowler.com/articles/injection.html.
Techdynasty. “Dependency Injection in Flutter - Techdynasty - Medium.” Medium, 12 Oct. 2023, techdynasty.medium.com/dependency-injection-in-flutter-0f308870d1a5.
What Is an Interface? (the JavaTM Tutorials >Learning the Java Language > Object-Oriented Programming Concepts). docs.oracle.com/javase/tutorial/java/concepts/interface.html.