Walturn’s Best Practices for Backend Development
Aug 26, 2024
Engineering
Best Practices
Walturn
Summary
Walturn follows best practices in backend development, utilizing advanced tools and methodologies for optimal performance, security, and reliability. This article explores our approach to framework choice, code practices, infrastructure recommendations for startups and enterprises, database management, the integration of secure software engineering, and AI use cases in backend development.
Key insights:
NestJS Framework: Walturn uses NestJS for backend systems due to its modular architecture, microservice support, and TypeScript integration, promoting scalability and maintainability.
TypeScript Usage: TypeScript's static typing and enhanced IDE support improve development efficiency and error detection, making it Walturn's primary language for backend development.
SQL Best Practices: SQL is used for database management, with a focus on best practices and implementing security measures to prevent SQL injections.
Python for Scripting: Python is preferred for its readability and extensive library support, enabling efficient automation and scripting tasks in backend development.
RESTful API Design: Walturn adheres to REST principles, ensuring intuitive, consistent, and maintainable APIs, crucial for scalability and ease of integration.
Dependency Injection: Leveraging NestJS's dependency injection system, Walturn enhances modularity and testability by decoupling components and dependencies.
Automated Testing: Automated testing with Jest and supertest ensures high test coverage, faster feedback loops, and reduced testing costs, contributing to reliable software releases.
Infrastructure Recommendations: Walturn recommends platforms like Render, Heroku, Railway, Supabase, and Google Cloud for startups, while recommending AWS for enterprise infrastructure due to its scalability, security, and global reach.
Error Handling Practices: Walturn emphasizes the importance of clear and consistent error codes, appropriate HTTP status codes, and robust logging mechanisms to ensure stability and quick issue resolution.
CI/CD Integration: Walturn utilizes CI/CD pipelines through GitHub Actions, focusing on automated testing, secure deployments, and continuous improvement to deliver high-quality software rapidly.
API Versioning: We follow best practices for API versioning to avoid breaking changes and ensure smooth transitions, with a focus on extensibility, consumer awareness, and backward compatibility.
Security and Privacy: We incorporate security and privacy into the SDLC, emphasizing the use of environment variables for key storage, anti-SQL injection measures, and the principle of least privilege.
Monitoring and APM Tools: Walturn leverages Better Stack for monitoring and Sentry for application performance management, ensuring real-time insights, anomaly detection, and proactive issue resolution.
Documentation Standards: We maintain comprehensive, up-to-date documentation using tools like Swagger and Google Docs, ensuring clear project understanding and facilitating onboarding.
Use of AI: Integrating AI into backend development for tasks like automated bug detection, efficient database management, and intelligent error handling can enhance productivity and innovation.
Introduction
Backend engineering is responsible for managing data, business logic, and server-side operations of any application. As applications grow in complexity and scale, following the best industry practices becomes essential to maintain performance, security, and reliability.
At Walturn, we prioritize best practices and use a suite of advanced tools and methodologies to ensure our backend systems are robust and scalable. This comprehensive article explores the best practices that we implement, along with the tools we recommend to optimize workflow to deliver high-quality products.
Framework of Choice
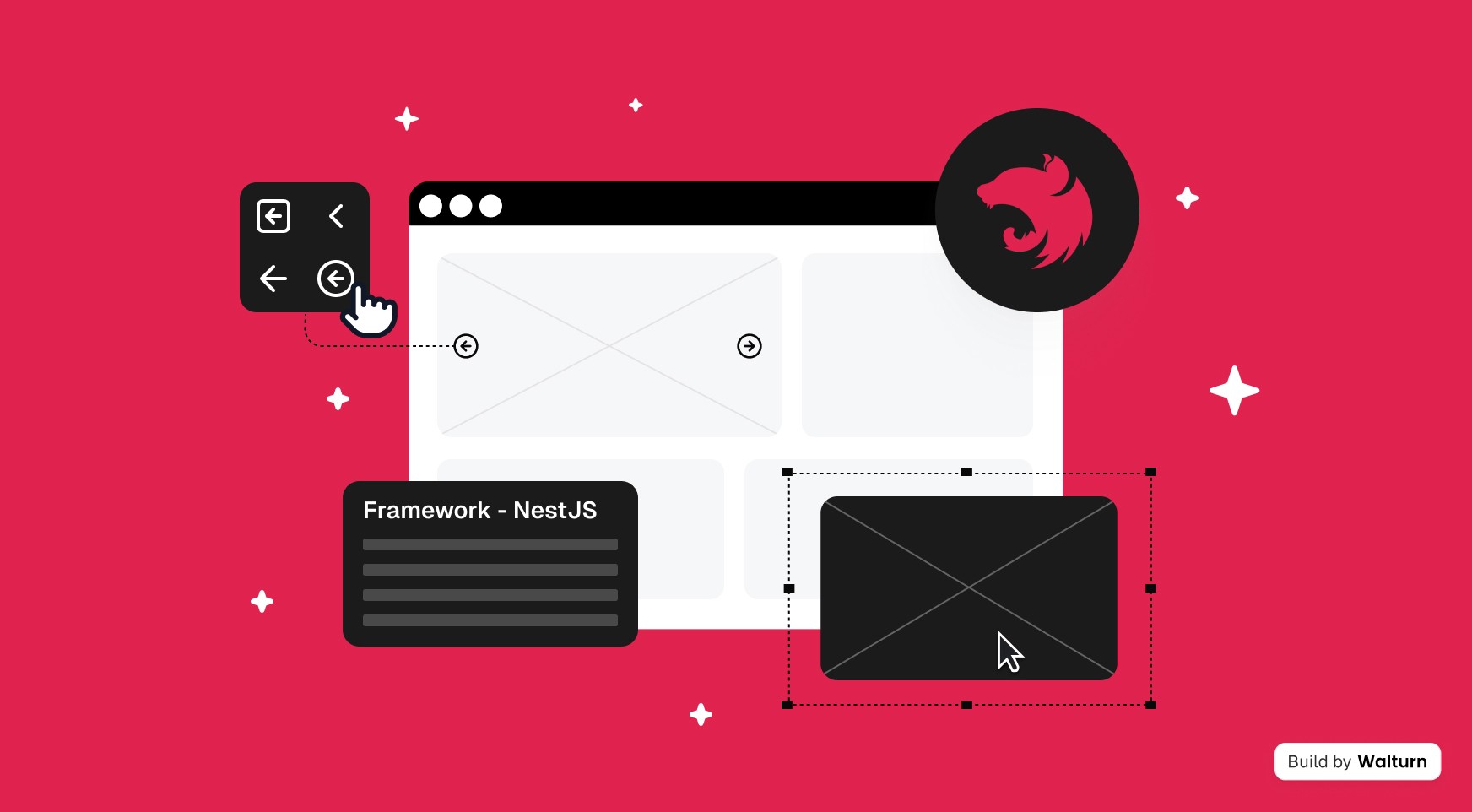
At Walturn, we use NestJS to build our backend systems. NestJS is a framework for building server-side applications and is highly extensible and versatile, offering a modular architecture that allows us to organize our applications into easily manageable modules.
The decision to choose NestJS as our primary framework was heavily influenced by the following:
1. Scalability and Maintainability
NestJS promotes a modular architecture, allowing developers to break down applications into smaller and more manageable modules. This approach improves both the scalability and maintainability of the codebase by enabling developers to isolate separate functionalities - achieving strong Separation of Concerns.
2. Microservice Support
NestJS offers native support for microservices that allows for the development of independent services that can be developed, deployed and maintained separately. By leveraging this, our team can create smaller, manageable components that communicate through well-defined interfaces.
3. TypeScript
NestJS is built with TypeScript which offers benefits such as type safety, code organization, and early error detection.
4. Ecosystem
NestJS has a strong ecosystem with a wide range of plugins, libraries, and third-party packages that extend its capabilities. This allows us to use ready-made solutions for common problems which aids in the acceleration of the development process.
5. Dependency Injection
NestJS’ dependency injection system further enhanced modularity by decoupling components and their dependencies. This makes the codebase more manageable and improves testability by allowing developers to inject mock dependencies during testing.
6. Web Application Development
NestJS provides comprehensive support for building various types of web applications including REST APIs, GraphQL APIs, queues, and real-time and event-driven applications. This allows developers to quickly create applications that can handle a wide range of use cases.
At Walturn, we aim to build high-quality products by following best industry practices. These include:
1. Clean and Modular Code
We follow a modular architecture in our backend system which allows for better scalability, easier maintenance, and enhanced reusability across projects. This approach helps in organizing code logically and supports Separation of Concerns (SoC) which is crucial for long-term project sustainability.
2. RESTful API Design
Our APIs follow the constraints set by the REST architecture style and adhere to the best practices of clear and consistent endpoint naming, proper HTTP status codes, and lightweight JSON formatting. This ensures that our APIs are intuitive and easy to maintain.
3. Utilize Dependency Injection
As discussed previously, NestJS allows us to implement a robust dependency injection system. This approach enhances testability and ensures components remain decoupled.
4. Implement Middleware and Guards
Leverage middleware for tasks such as logging and guards for enforcing authentication and authorization policies. These features help process requests efficiently and securely before they reach the business logic layers.
5. Utilize the Ecosystem
Take advantage of the NestJS ecosystem by integrating existing plugins and libraries. This saves development time, ensures consistency, and allows developers to focus on more critical tasks.
Preferred Languages
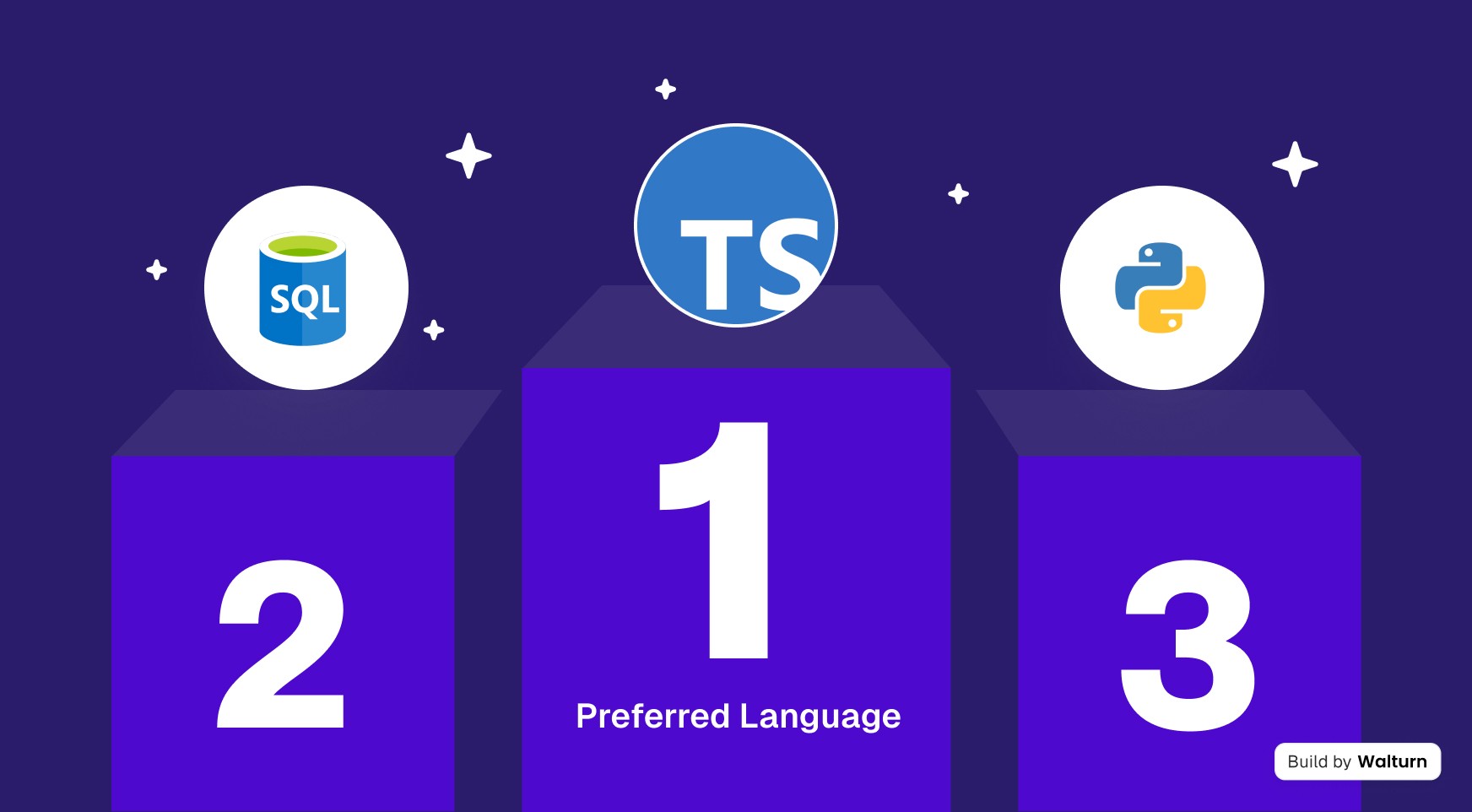
For our backend systems, our primary languages of choice are TypeScript, SQL, and Python. The StackOverflow 2024 Survey, of 45,566 respondents, shows that all three of these languages ranked in the top five most popular technologies among professional developers - representing their wide adoption in the tech industry.
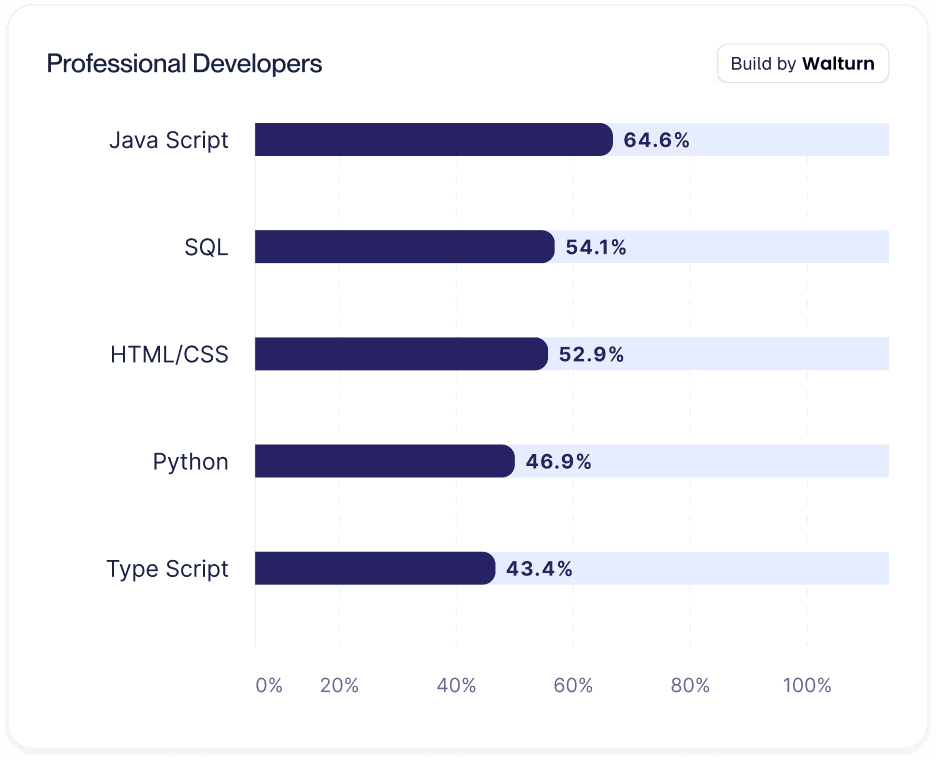
In this section, we will delve deeper into each of these languages and the potential benefits they bring to us as our choice of languages.
1. TypeScript
TypeScript is an extension of JavaScript to facilitate the development of large-scale JavaScript applications. TypeScript introduces a module system, classes, interface, and a rich gradual type system to JavaScript, aiming to enhance the developer experience. The benefits of using TypeScript include:
Static Typing: TypeScript provides optional static typing which helps catch errors early during the development process.
Enhanced IDE Support: The type information in TypeScript allows for better tooling and IDE features like auto-completion, navigation, and refactoring.
Gradual Typing: TypeScript supports gradual typing which allows developers to apply types to parts of their program incrementally. This is particularly helpful to adopt TypeScript in existing JavaScript codebases that need to be updated.
Compatibility with JavaScript: As a strict superset of JavaScript, TypeScript is compatible with all existing JavaScript libraries and frameworks. This also means that TypeScript can run on any application or operating system that supports JavaScript.
Modularity and Maintainability: TypeScript’s module system and the introduction of classes and interfaces help organize code more effectively, resulting in higher maintainability and scalability.
These benefits make TypeScript an excellent choice for large-scale projects. At Walturn, we follow the best practices when working with TypeScript according to its guidelines. These include:
Primitive Types: Use number, string, boolean, and symbol instead of Number, String, Boolean, and Symbol. These primitive types ensure proper type checking and avoid issues associated with boxed objects.
Object Type: Use object instead of Object. This type was introduced in TypeScript 2.2 and provides a more accurate representation of non-primitive types which aims to improve type safety.
Generics: Avoid generic types that do not use their type parameters to avoid errors that can occur from used parameters.
Avoid any: Minimize the use of any unless migrating from a JavaScript project. This type disables type checking, which should be avoided to maintain robust type safety. Instead, prioritize using unknown as it requires an explicit type assertion before usage.
Void for Callbacks: Use void for callbacks whose return value will be ignored. This prevents accidentally using the return value of the callback in an unchecked way.
Non-Optional Parameters: Avoid optional parameters in callbacks unless necessary. These can lead to unintended behavior if not handled correctly.
Sort Overloads: Place more general overloads after more specific ones. TypeScript resolves function calls by choosing the first matching overload. By putting specific overloads first, the functional call prioritizes these.
2. SQL
SQL (Structured Query Language) is a programming language used for managing and manipulating relational databases. It allows users to store, retrieve, update, and delete data within a database. SQL operates through various easy-to-understand commands such as INSERT, SELECT, UPDATE, and DELETE to interact with the data stored in a tabular form.
The benefits of using SQL include:
Ease of Use: SQL uses common English keywords in its syntax, which makes it easy to use.
Integration: SQL integrates well with programming languages such as Java, Python, and C#. This allows developers to embed SQL queries within their applications.
Procedures: Similar to functions, developers can define procedures, which are collections of SQL statements. This allows for code reuse.
Flexibility: SQL can perform complex queries involving matrix manipulations and optimization, making it suitable for a wide range of applications.
Standardization: The American National Standards Institute (ANSI) and the International Organization for Standardization (ISO) have adopted SQL standards since 1986. This ensures SQL’s compatibility across different database systems.
At Walturn, we follow the best industry practices for writing SQL. These include:
Alignment: Ensuring proper alignment of code elements makes the code easier to read, especially in complex queries that may include correlated and nested queries.
Avoid select *: Instead of accessing the entire table using select *, specify the columns that need to be retrieved. This helps identify exactly what data is being retrieved and avoid unnecessary data in the memory.
Aliasing: Use clear and meaningful aliases for tables and fields. This enhances code clarity and reduces complexity.
Comments: Use comments to describe complex queries for easier understanding and knowledge sharing.
Clarity: Always clearly specify which fields of the table you are working with. For example, in GROUP BY statements, explicitly mention the field name instead of index-based ordering.
Create Views: Create views for specific user roles. This helps secure the application by restricting what fields are accessible to each user.
Security: Place proper security controls to avoid unauthorized access. For example, instead of placing user input directly into the query, validate their input to ensure no malicious query is present (e.g., SQL Injections).
3. Python
At Walturn, we utilize Python to write scripts that automate various tasks. Python’s readability, flexibility, and extensive library support make it a popular choice for developing efficient scripts.
Python is generally considered a beginner-friendly language due to its easy syntax and extensive set of libraries. This is backed up by Stack Overflow’s survey, which unveils 66.4% of users that learning to code start with Python. This also makes Python an ideal choice for developing scripts that may be utilized by various team members who do not possess extensive programming knowledge. Benefits of using Python include:
Readability: Python’s syntax is readable and straightforward, making it easier for developers to understand and modify code.
Compatibility: Python is compatible with most operating systems and architectures such as Windows, Linux, macOS, and more. This ensures that Python scripts can be run in diverse environments.
Extensive Standard Library: Python comes pre-equipped with a comprehensive standard library that includes modules for various tasks. This speeds up development as code does not need to be written from scratch.
Popularity: Python is used widely by a large community as discussed previously. This means that developers have access to numerous resources, tutorials, and third-party libraries when using Python.
Infrastructure for Startups
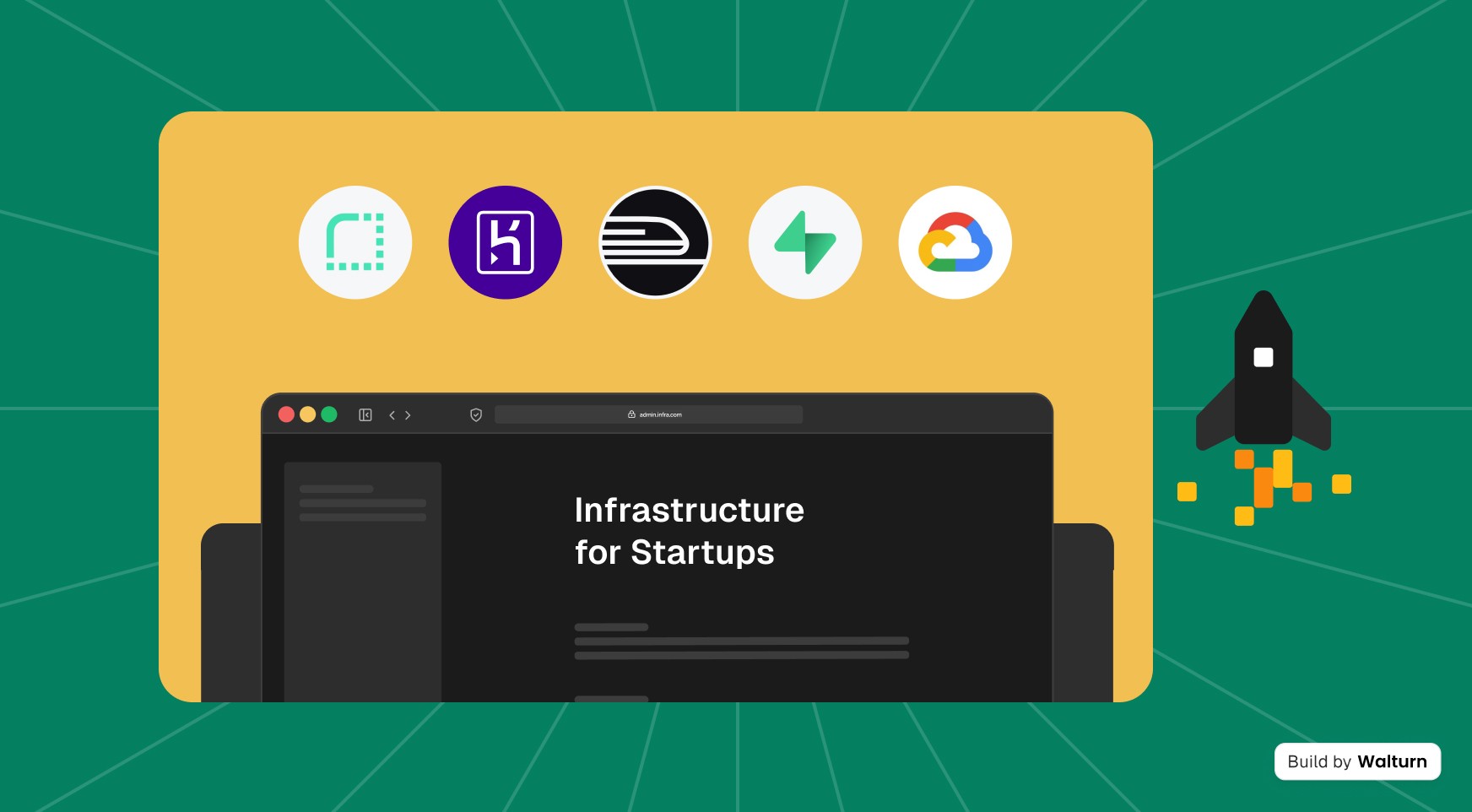
At Walturn, we recommend infrastructure options such as Render, Heroku, or Railway for startups. These platforms provide easy-to-use, scalable, and cost-effective solutions. Services like Supabase and Google Cloud also offer flexible infrastructure options that can be tailored to the specific needs of startups. In this section, we will talk about each of these platforms and their benefits to help clients navigate through the choice of infrastructure.
1. Render
Render is an excellent cloud application hosting service that we recommend for startups. It supports a variety of service types including web services, static sites, and background workers which makes it a good choice for different startup needs. It offers competitive pricing plans starting at $19 per user/month for small teams and early-stage startups. Additional benefits and key features include:
Compliance: Render provides full GDPR and SOC 2 Type II compliance, ensuring your data is secure and meetings essential regulatory requirements.
Automatic Deploys: Render automatically updates your applications on every push with zero downtime. This continuous integration and deployment capability ensures that applications remain up-to-date without manual intervention - allowing your team to focus on development.
Native Language Runtimes: Render offers managed environments for multiple programming languages. Additionally, you can deploy custom applications using Docker which provides the flexibility to run virtually any stack in a managed, scalable environment.
Load-Based Autoscaling: Automatically adjusts the number of instances based on traffic which ensures optimal resource usage and cost-efficiency.
DDoS Protection: Includes built-in protection against denial-of-service attacks. This security feature shields your applications from malicious traffic without requiring additional setup, safeguarding your startup's digital assets.
System Monitoring: Provides comprehensive system monitoring with uptime checks, health monitoring, and instant rollbacks to help maintain high availability and a quick response to issues.
Managed PostgreSQL: Render provides a fully managed PostgreSQL database with built-in support for automated backups, point-in-time recovery, and encryption.
Redis: Render offers managed Redis instances with support for persistent storage which means data is written to the disk every second. This provides high availability and ensures fast access to cached data.
2. Heroku
Like Render, Heroku is another cloud platform that allows developers to build, run, and operate applications. It simplifies the process of application deployment and management which makes it a good choice for startups looking to scale quickly without worrying about infrastructure management. Its benefits and key features include:
Compliance: Heroku provides compliance with industry standards like HIPAA and PCI for sensitive applications.
Quick Deployment: Heroku supports Git-based deployments, enabling continuous integration and delivery workflows. Developers can push code changes directly to Heroku, which automatically builds, runs, and scales the application within smart containers called dynos.
Language Support: Heroku supports a wide range of programming languages, allowing startups to work with their preferred technologies.
Integrated Development Tools: Heroku's continuous delivery pipeline, Heroku Flow, integrates with GitHub to automate application staging, testing, and deployment. Heroku Pipelines provide a visual representation of the development workflow, from staging to production, making it easier to manage multiple environments.
Managed Services: Heroku also offers managed services like Heroku Postgres and Heroku Redis to reduce the operational burden on startups.
Flexible Pricing: Heroku’s pricing model is designed to be pay-as-you-go. This flexibility is beneficial for startups which allows for cheap proof-of-concepts (e.g., $5 per month for Eco dynos) and allows for easy scaling as their application progresses. There are no termination fees.
System Monitoring: Provides visual monitoring of your application with metrics like response time, throughput, memory usage, and more.
3. Railway
Railway is also a highly suitable platform for startups due to its powerful features. With pricing as low as $5, startups can build initial product releases and evaluate their compatibility with Railway for moving to more robust plans. The platform offers all the essential tools to build, deploy, and scale applications without worrying about infrastructure management. Its benefits and key features include:
Compliance: Railway is SOC 2 Type I certified and offers HIPAA compliance for startups handling sensitive healthcare data. Additionally, they expect to be SOC 2 Type II certified by the end of 2024.
Health Checks and Restarts: Railway includes built-in health checks and automatic restart capabilities to ensure services are always running smoothly.
Automated Deployments: Railway enables fast application deployments. This includes handling containerization, networking, and secrets.
Scaling: Railway adjusts the application’s resources in real-time to match workload demands to ensure it can handle spikes in traffic without interruption.
Monitoring: Railway offers real-time logging and metrics for all services, databases, and plugins. This includes detailed logs, configurable alerts, and more.
Automated CI/CD: Integrates with GitHub for CI/CD. This allows every code push to trigger automated deployment.
Integrated Services: Allows developers to integrate various databases including PostgreSQL and Redis within the platform.
4. Supabase
Supabase offers open-source Backend as a Service (BaaS) as an alternative to Firebase. It is a comprehensive platform that includes backend tools such as databases, user authentication, serverless storage, and more to help developers build applications quickly and efficiently. Supabase offers various pricing plans, including a free plan that is suitable for demonstration purposes. Its benefits and key features include:
Compliance: Supabase is SOC 2 Type II certified and HIPAA compliant which ensures that data handling meets industry practices for security and privacy.
Database: Supabase offers a fully portable and scalable Postgres database. It supports advanced features like Row Level Security (RLS) and comes with automatic backups, branching, and real-time replication.
Authentication: Offers built-in authentication with support for multiple OAuth providers such as Google and custom authentication options.
Serverless Storage: Offers serverless storage for handling and serving large files such as images and videos. It includes a built-in Content Delivery Network (CDN) for optimized delivery and supports features like image transformations.
AI Integration: Supports the storage and management of vector embeddings, making it easier to integrate Machine Learning (ML) models into your application. Additionally, it supports popular ML frameworks like OpenAI and Hugging Face.
Edge Functions: Allows for the deployment and monitoring of serverless functions that are distributed around the globe, closer to users to reduce latency. These functions are automatically managed and scale automatically as per service traffic.
5. Google Cloud
Google Cloud is a cloud computing platform designed to help businesses of all sizes build, deploy, and scale applications. It offers an extensive tool of services that work together to create a seamless ecosystem backed by Google. The benefits and key features of Google Cloud include:
Scalable: Google Cloud offers a variety of services that can be scaled according to growing business needs.
Pricing: Google Cloud’s pay-as-you-go pricing model allows startups to only pay for the resources they use. Additionally, Google provides $300 in free credits for new customers and access to products at no cost up to certain usage limits. This can be helpful for startups looking to enter the market with initial product versions.
Security: Offers advanced security features including data encryption and identity management.
Global Network: Google has established an extensive global network. This helps Google Cloud provide low-latency access to applications and data which enhances user experience worldwide.
AI and ML Integration: Google Cloud offers a wide array of AI and ML services such as Vertex AI to allow startups to develop and deploy applications with state-of-the-art technologies.
Developer-Friendly Tools: Offers a rich ecosystem of development tools that supports various programming languages and frameworks. These tools, along with integrated CI/CD pipelines, facilitate rapid development and deployment.
Google Cloud offers a very extensive set of features for specific industries. As we cannot cover all of these features, we recommend taking a look at their services for better understanding. Its extensive offerings make Google Cloud an excellent and robust choice for startups across various industries.
Walturn recommends these five platforms for startups due to their flexibility, cost-effectiveness, and rich feature set. Reach out to us to get started.
Infrastructure for Enterprises
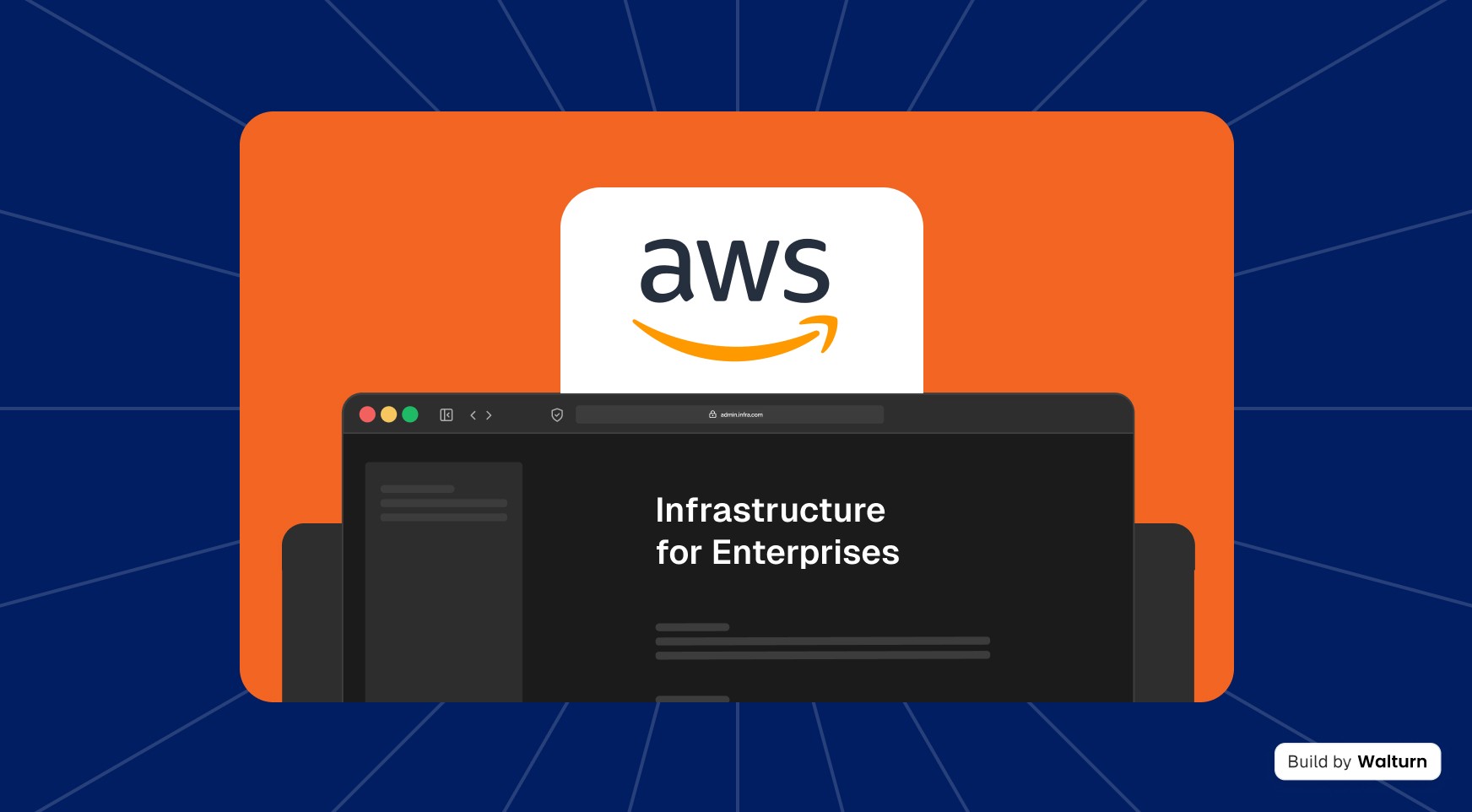
Walturn recommends Amazon Web Services (AWS) as the infrastructure of choice for enterprises due to its comprehensive platform. AWS offers a wide range of services and solutions that cater specifically to the needs of enterprises to ensure they can achieve optimal efficiency.
1. What is AWS?
Amazon Web Services (AWS) is a comprehensive cloud computing platform that provides a set of global cloud-based products including compute power, storage options, networking, and databases. These services are designed to help businesses scale and grow.
It allows businesses to deploy and manage applications on a global scale with minimal overhead. They provide the confidence to owners that they are utilizing the most secure and reliable cloud infrastructure available for large-scale deployments.
2. Pricing
AWS pricing is based on a pay-as-you-go model which offers flexibility for enterprises. This allows businesses to scale their infrastructure according to evolving needs without incurring unnecessary costs. This also eliminates the need for long-term contracts or complex licensing agreements.
Moreover, AWS offers a Free Tier which allows new users to explore and test AWS at no cost for up to 12 months before formally committing to a more comprehensive plan.
3. Key Features and Benefits
Scalability and Flexibility: AWS provides on-demand resources that can be scaled up or down as the business evolves. This is useful for enterprises that experience variable workloads and need to ensure their infrastructure is efficiently able to handle the workload.
Security and Compliance: AWS offers extensive support for compliance and standards around the globe to cater to diverse businesses in varying industries. These include GDPR, FERPA, HIPAA, SOC 2, CCPA, and more. For a complete list of supported certifications, take a look at their official compliance program.
Global Reach: AWS’ global infrastructure extends to multiple geographic regions and availability zones. This ensures that enterprises can deploy applications closer to their customers to improve latency, user experience, and disaster recovery capabilities.
Continuous Improvement: AWS continuously adds to its already extensive set of features. This ensures that enterprises have access to the latest technologies without needing to invest in third-party applications.
Artificial Intelligence: AWS provides dedicated tools to test and deploy AI and ML technologies into applications.
AWS is the recommended choice for enterprises by Walturn due to its comprehensive, scalable, and secure cloud infrastructure. Its extensive feature set, flexible pricing model, and global reach make it an ideal choice for businesses looking to grow and cater to users around the world.
Database of Choice
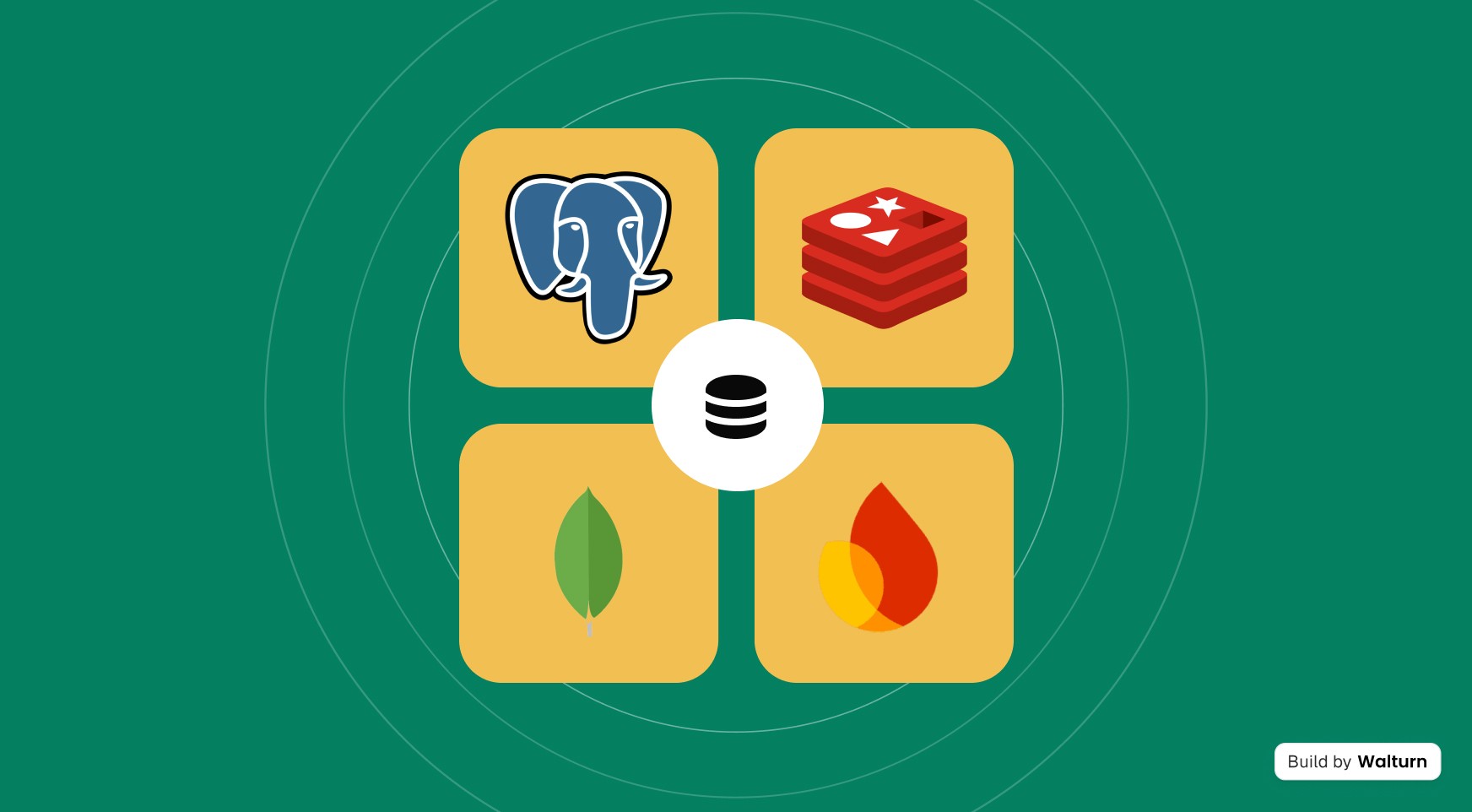
At Walturn, our databases of choice are PostgreSQL, Redis, MongoDB, and Firebase. All four of these database choices are excellent due to their robust management and efficiency. In this section, we will delve deeper into each offering to help organizations understand which one meets their needs best.
1. PostgreSQL
PostgreSQL is a powerful and robust relational database management system (RDBMS). It stores data in a standard tabular structure and utilizes SQL for queries, enabling developers to write highly efficient queries.
One of the significant advantages of PostgreSQL is its ACID compliance, which ensures reliable transaction processing and makes it ideal for handling sensitive data. Additionally, PostgreSQL supports numerous extensions, offering developers a more versatile and streamlined experience.
PostgreSQL is a great choice for applications requiring complex queries and transactions
2. Redis
Redis is an excellent tool for in-memory data storage. In-memory storage is optimal for data that needs to be read or written quickly or frequently, as memory provides faster processing compared to disk storage.
Redis is ideal for caching and session management. It uses key-value pairs to enhance performance further. However, it's important to note that Redis is not suitable as a traditional database for large amounts of data, as this would be an extremely inefficient use of memory.
3. MongoDB
MongoDB is a NoSQL document store that uses a JSON-like document model (BSON) for data storage. It offers schema flexibility, allowing for dynamic schema design and enabling developers to work with schema-less data structures. This makes MongoDB ideal for applications requiring flexible, schema-less data storage.
The database is horizontally scalable with sharding, allowing it to handle large amounts of data and distribute it across multiple servers. MongoDB supports indexing for fast query performance and features a powerful aggregation framework for data processing and transformation. It is particularly efficient at handling hierarchical data structures. However, it is not as strong in handling complex transactions as relational databases.
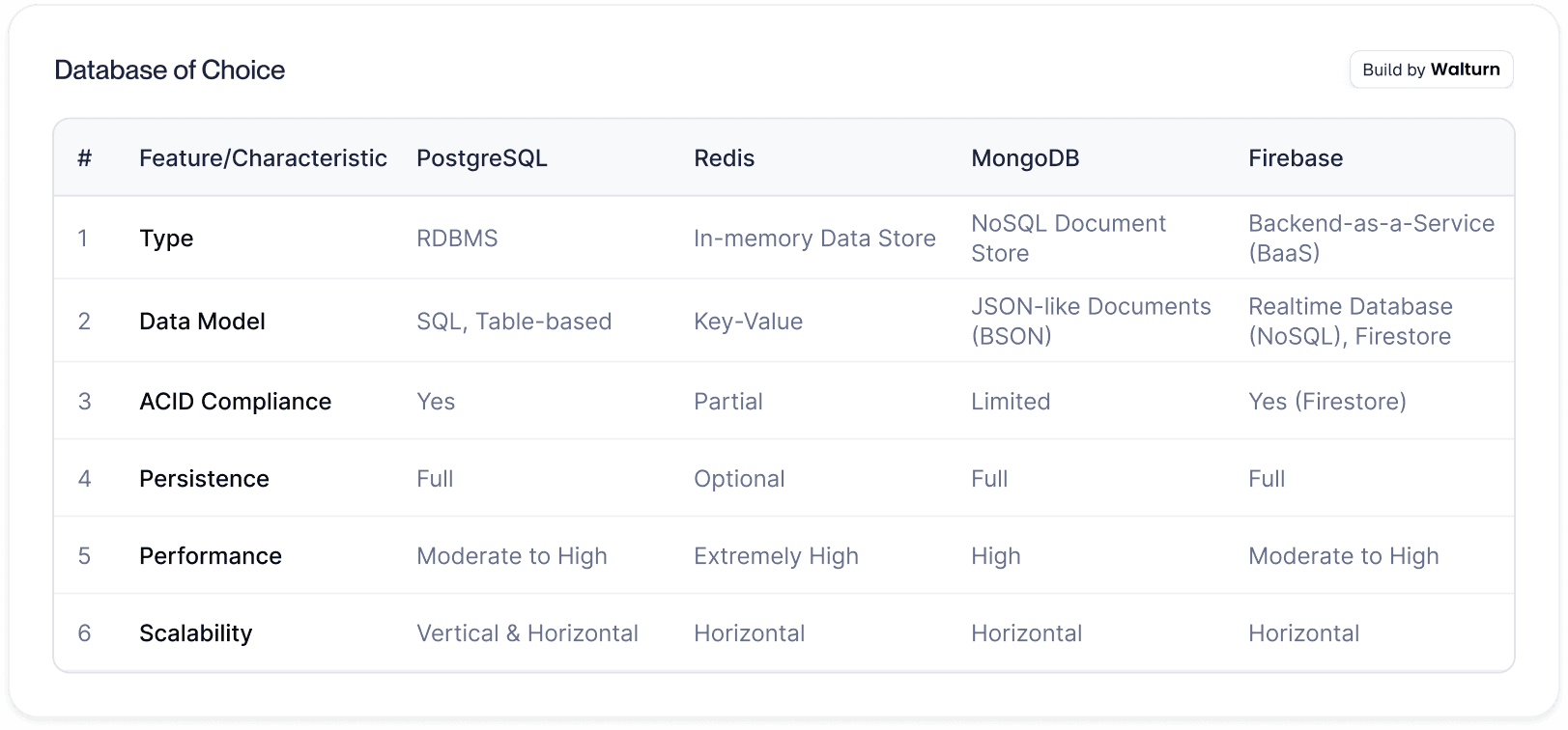
Database Hosting for Startups
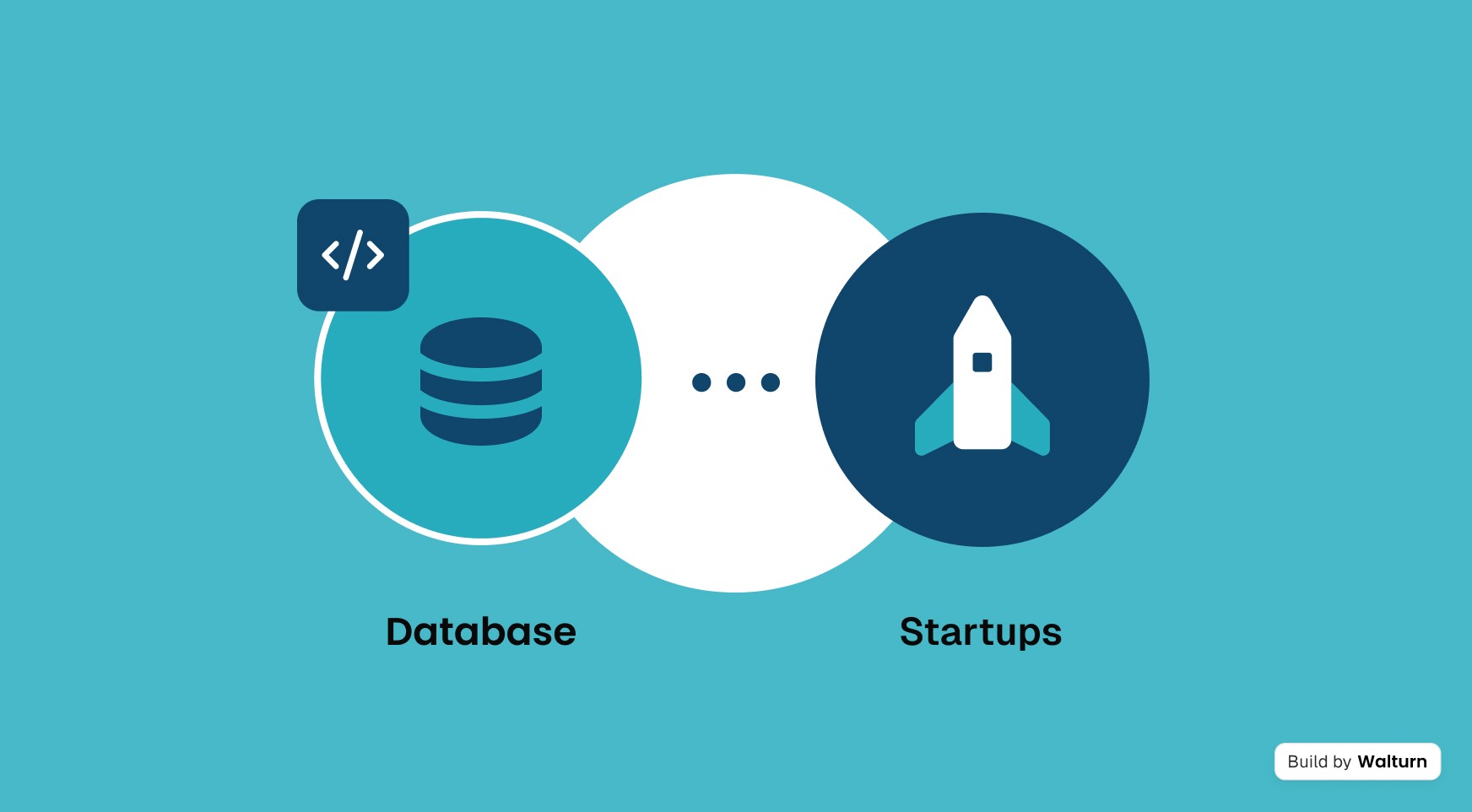
Walturn recommends Google Cloud (firebase), Supabase, Mongo Atlas, Render (PostgreSQL), and Render & Heroku (Redis) to startups for database hosting. This section provides an overview of each tool along with their key features.
1. Google Cloud (Firebase)
Google Cloud offers a comprehensive suite of backend services to support mobile and web application development. It includes the Realtime Database and Firestore for real-time data synchronization and storage. Key features include:
Real-Time Data Synchronization and Storage: Firebase's Realtime Database and Firestore enable real-time data synchronization and storage, ensuring that data is immediately available across all clients. This feature is crucial for applications that require live updates and consistent data across different platforms.
Seamless Integration: Firebase seamlessly integrates with other Firebase services, such as authentication, analytics, and cloud functions, offering a cohesive development experience. This integration simplifies the development process by providing a unified platform for various backend needs.
Hosting Services: Firebase provides hosting services for web applications, allowing developers to deploy their web apps directly from the Firebase console. This feature ensures easy deployment and scalability of web applications without the need for complex server configurations.
Automatic Scalability: Firebase automatically scales with application demand, ensuring that performance remains consistent even as the user base grows. This scalability is especially beneficial for applications with variable or rapidly increasing traffic.
Rapid Development and Deployment: Firebase offers a full suite of backend services, making it ideal for rapid development and deployment. Its optimization for mobile and web apps ensures a streamlined and efficient development process, allowing developers to focus more on building features rather than managing infrastructure.
2. Supabase
As discussed in previous sections, Supabase is an open-source alternative to Firebase that provides a suite of backend services to help developers build applications quickly. It leverages PostgreSQL for its database, offering a powerful relational database management system with a real-time API. Key features of its database include:
Dedicated PostgreSQL Database: Each Supabase project allocates its own isolated PostgreSQL instance. This ensures optimal performance, security, and full control over database configurations.
Portability: Supabase allows for integration with an existing PostgreSQL database. This helps import and migrate databases in and out at any time without lock-in.
Row Level Security: Implements PostgreSQL’s Row Level Security (RLS) mechanisms to enable precise control over who can access or modify specific rows in tables.
Real-Time Data Synchronization: Supabase enables real-time data synchronization across clients, ensuring that any changes in the database are immediately reflected in all connected clients. This feature is particularly useful for applications that require live updates, such as chat applications or collaborative tools.
Dashboard: The Supabase dashboard provides a user-friendly Table Editor for simple data manipulation and a powerful SQL Editor for running complex queries.
API Auto-generation: Supabase automatically generates APIs from your database schema, eliminating the need to manually create endpoints for CRUD operations.
Postgres Extensions: Supabase supports a wide range of PostgreSQL extensions. This extends the capabilities of existing databases without additional configuration.
Read Replicas: Supabase supports the use of read replicas, which allow you to distribute read queries across multiple databases to minimize load on the primary database and serve data closer to users.
3. MongoDB Atlas
MongoDB Atlas is a fully managed cloud database service for MongoDB. It offers the flexibility and scalability of MongoDB, combined with the convenience of automated infrastructure management.
Schema Flexibility: MongoDB Atlas provides schema flexibility, allowing for dynamic schema design. This feature is ideal for applications requiring flexible, schema-less data storage, enabling developers to work with evolving data models without the need for complex migrations.
Horizontal Scalability: The database is horizontally scalable with sharding, allowing it to handle large amounts of data and distribute it across multiple servers. This scalability ensures that MongoDB Atlas can efficiently manage growing datasets and high traffic.
Indexing for Fast Queries: MongoDB supports indexing for fast query performance, enabling efficient data retrieval. This feature is essential for applications that require quick access to large datasets, ensuring optimal performance.
Powerful Aggregation Framework: MongoDB Atlas features a powerful aggregation framework for data processing and transformation. This framework allows developers to perform complex data manipulations and analyses directly within the database.
Automated Management: MongoDB Atlas reduces operational overhead by offering automated backups, monitoring, and scaling. These automated services simplify database management, allowing developers to focus more on application development.
4. Render (PostgreSQL)
Render is a cloud platform for deploying applications and services, including PostgreSQL databases. It provides fully managed PostgreSQL instances with automated backups and scaling.
Managed PostgreSQL Services: Render simplifies the deployment and management of PostgreSQL databases by offering managed services. This includes automated backups, ensuring data safety and integrity without manual intervention.
ACID Compliance: PostgreSQL's ACID compliance ensures reliable transaction processing, making it ideal for applications requiring robust querying and transaction capabilities. This feature guarantees data consistency, integrity, and durability.
Scalability Options: Render offers vertical and horizontal scaling options, providing moderate to high performance depending on the instance type. This scalability ensures that the database can handle varying workloads efficiently.
Streamlined Developer Experience: Render provides a streamlined experience for developers by managing the underlying infrastructure. This allows developers to focus more on building their applications rather than managing database servers.
5. Render & Heroku (Redis)
Render and Heroku both offer managed Redis services, providing in-memory data storage for caching, session management, and other use cases requiring fast data access.
In-Memory Data Storage: Redis is an excellent tool for in-memory data storage, designed to provide ultra-fast access to data by storing it directly in the server's main memory. This feature ensures minimal latency and quick data retrieval.
Key-Value Pairs for Performance: Redis uses key-value pairs to enhance performance further. This simple data structure allows for rapid read and write operations, making Redis ideal for applications that require high-speed data processing.
Use Cases for Caching and Session Management: Redis is particularly suited for caching and session management. These use cases benefit from Redis's ability to quickly store and retrieve frequently accessed data, improving overall application performance.
Not Suitable for Large Data Sets: It is important to note that Redis is not suitable as a traditional database for large amounts of data, as this would be an extremely inefficient use of memory. Redis is best utilized for scenarios where speed is critical and data size is manageable.
Managed Services for Simplified Deployment: Both Render and Heroku simplify the deployment and management of Redis instances. This managed service approach ensures high performance and availability without the complexities of manual setup and maintenance.
Database Hosting for Enterprises
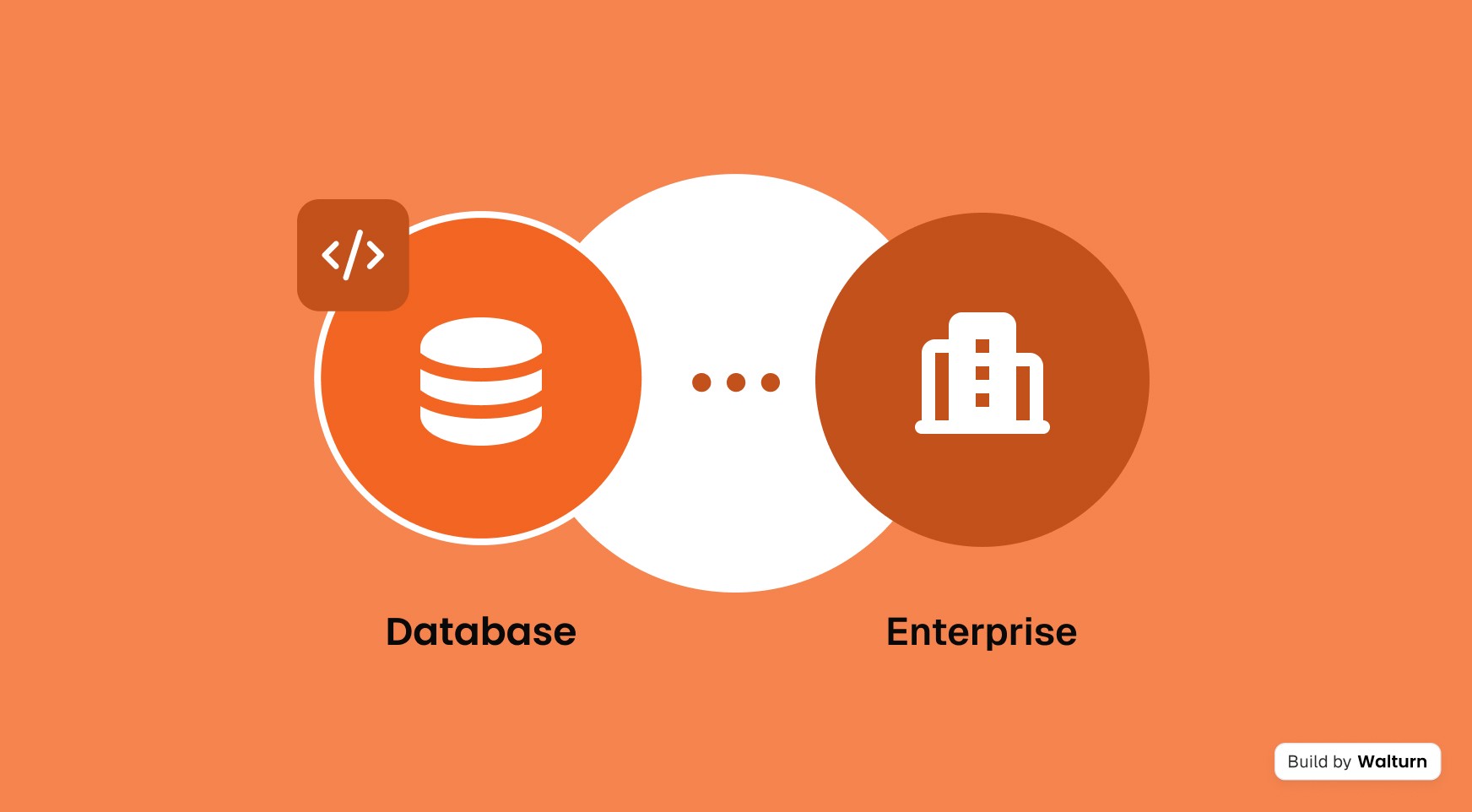
Walturn recommends Amazon Web Services (AWS) to enterprises for database hosting. As discussed previously, AWS is a comprehensive and robust platform that caters to the specific needs of enterprises that require large-scale operations.
AWS provides a broad array of cloud-based services, including database solutions like Amazon RDS, DynamoDB, and ElastiCache. AWS is renowned for its extensive features, reliability, and scalability, making it a premier choice for enterprise-level applications that require robust infrastructure, integration capabilities, and high-performance solutions.
1. Amazon RDS
Amazon RDS (Relational Database Service) supports various relational databases, including PostgreSQL, MySQL, and MariaDB. It offers managed services with automated backups, scaling, and high availability, ensuring reliable and efficient database management for enterprise applications.
2. DynamoDB
DynamoDB is a managed NoSQL database service that delivers high performance and scalability with automatic scaling and support for key-value and document data models. This flexibility makes DynamoDB ideal for applications with dynamic data requirements and high transaction volumes.
3. ElastiCache
ElastiCache is a managed caching service supporting Redis and Memcached, designed to enhance application performance by caching frequently accessed data. This service is particularly beneficial for improving response times and reducing the load on primary databases.
4. Comprehensive Solution for Enterprises
AWS offers extensive features for reliability, scalability, and integration with other AWS services, making it a comprehensive solution for diverse application needs. Its global reach and robust infrastructure make AWS particularly well-suited for large organizations with complex and demanding IT environments, emphasizing its suitability for enterprise-level applications.
DevOps
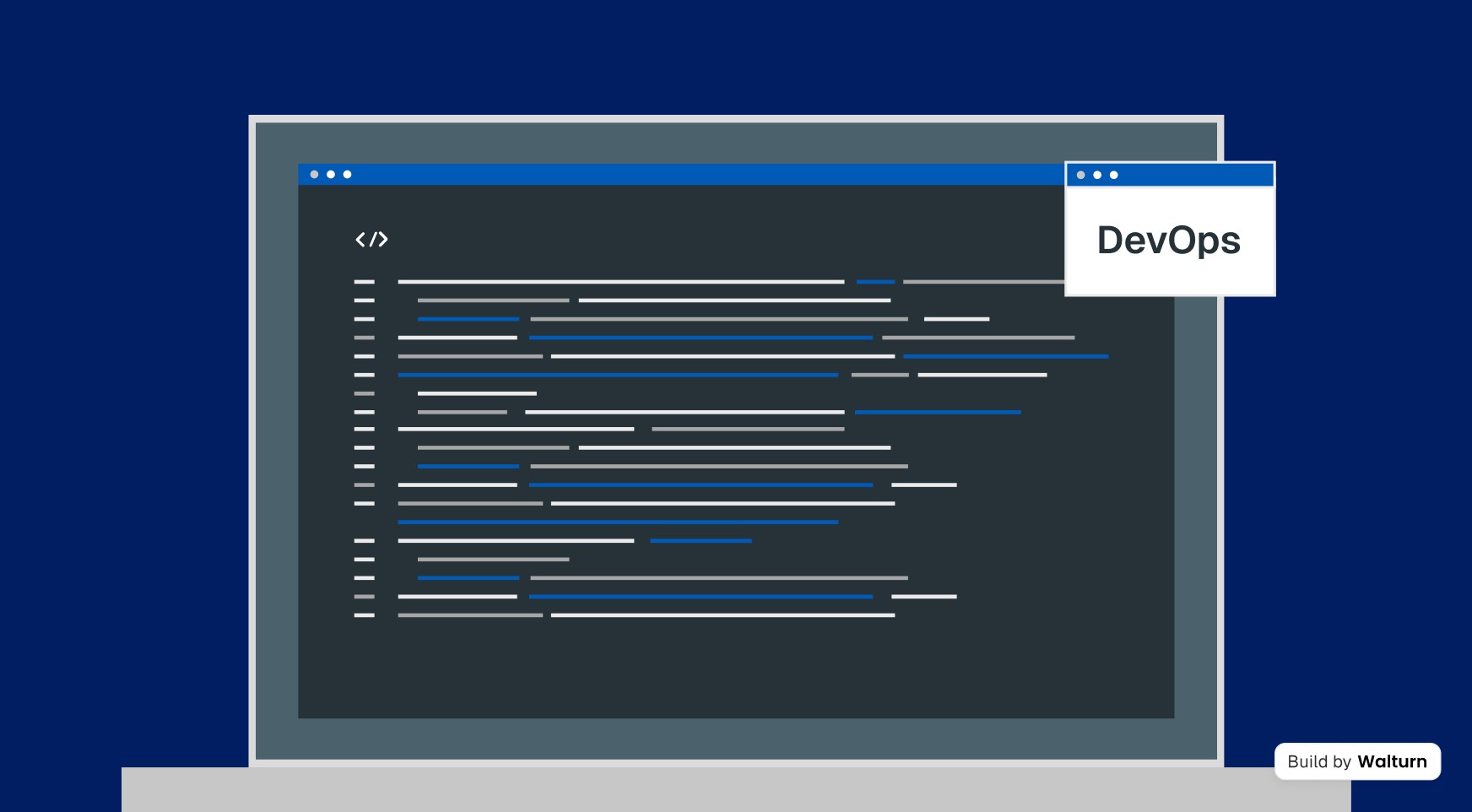
DevOps is a technical approach that enhances an organization’s ability to deliver high-quality applications rapidly and reliably. By combining the software development and operations teams, DevOps (Development and Operations) encourages collaboration, automation, and continuous improvement throughout the software lifecycle.
At Walturn, we leverage GitHub Actions, Heroku CI/CD, and Render CI/CD as our primary tools for DevOps in backend development.
Multiple case studies, including a 2019 analysis of five companies and a separate 2017 study, identified several common benefits of integrating DevOps into the software development life cycle:
1. Faster Time-to-Market
DevOps enables quicker release cycles by integrating development and operations. This integration significantly reduces the average release cycle time, improving the speed of software delivery. Furthermore, automation reduces manual steps and handoffs, lowering human error and improving the productivity of operations work
2. Better Communication
DevOps encourages open communication and shared responsibility between development and operations teams.
3. Improved Product Quality
By encouraging communication, DevOps reduces friction and helps in identifying and fixing bugs earlier, leading to higher-quality products. This results in increased confidence in deployments, improved code quality, and enhanced product value to customers.
4. Cultural Change
DevOps promotes a cultural shift towards shared responsibility and team collaboration. This encourages a mindset of trust and empowerment within the organization which not only boosts the organization’s productivity and reputation but also supports continuous improvement and innovation.
However, DevOps is a challenging strategy to incorporate within organizations. Therefore, it is essential to follow best practices. The following points cover industry best practices that Walturn follows to achieve a successful DevOps strategy:
1. Shift Left with CI/CD
Bring testing into the development process early by shifting left. This approach allows developers to address bugs and improve code from the very beginning, ensuring quality from the offset of the project.
2. Automation
Utilize a variety of tests such as end-to-end tests, unit tests, integration tests, smoke tests, performance tests, and security tests to ensure high coverage.
3. Emphasize Observability
Identify and focus on key metrics that are essential for your organization or project such as deployment time, failure rate, and code coverage. Establishing specific objectives for implementing DevOps guides the transitioning process for organizations that are looking to incorporate DevOps into their workflow.
Moreover, starting with small and manageable projects can be beneficial for smaller startups to evaluate DevOps practices, allowing for the gradual refinement of successful practices.
4. Learn from Past Failures
Continuously improve your processes to prevent repeating mistakes. Analyze the root causes of issues like unreported bugs or delayed resolutions and implement changes to mitigate these problems in the future.
5. Encourage DevOps Adoption
Encourage all team members to adopt DevOps by providing necessary training and support. This investment will allow your organization to take advantage of the benefits that DevOps methodologies have to offer.
6. Infrastructure as Code
This involves managing computing infrastructure through machine-readable definition files rather than physical hardware configuration. It allows for the reproducibility of environments which results in reduced configuration drift and improved consistency across development, testing, and production environments.
Monitoring
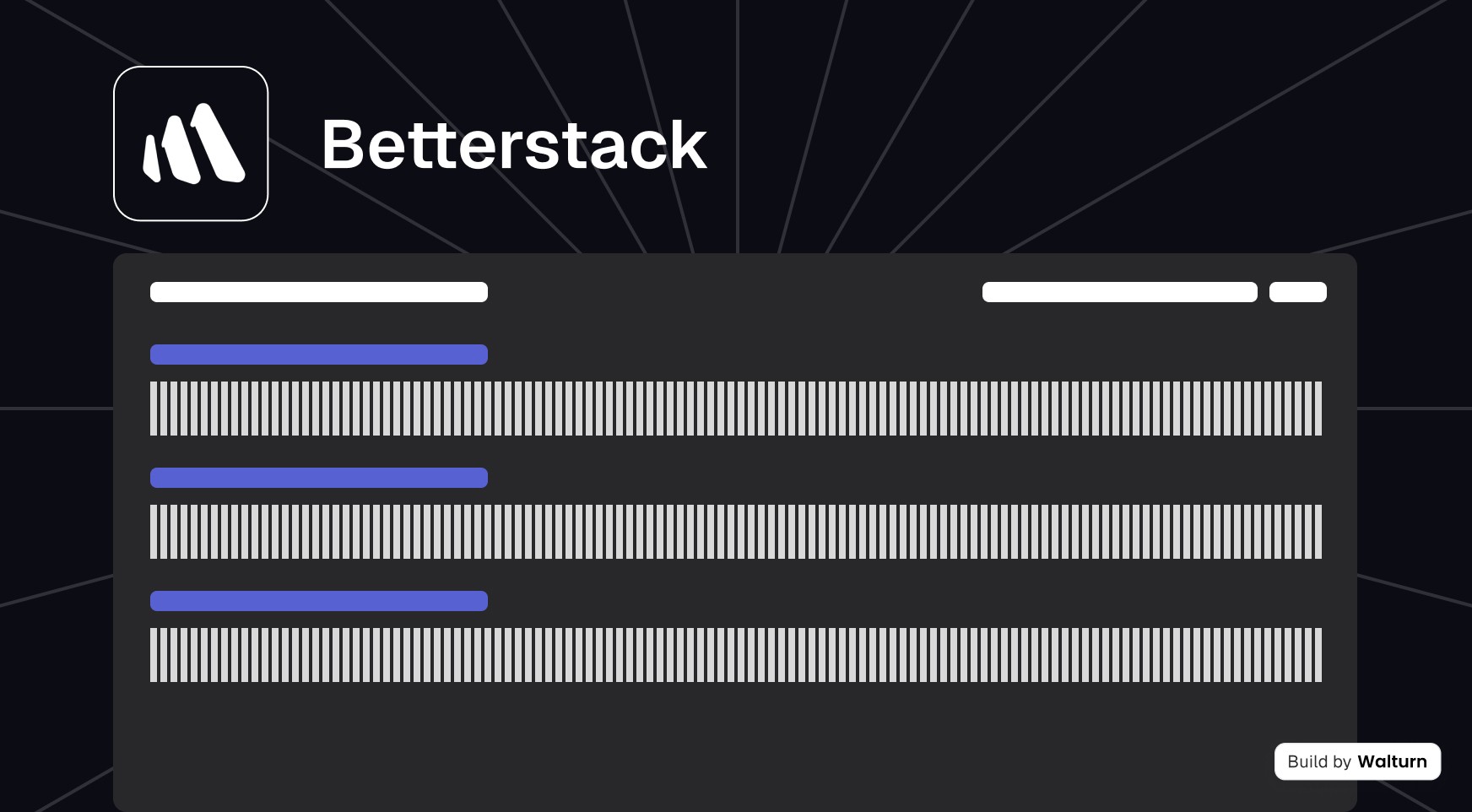
Monitoring involves tracking system performance, resource utilization, and application health to ensure optimal operation and reliability. This allows early detection of issues allowing teams to address them before they impact users.
At Walturn, we utilize Better Stack to monitor our systems and be notified of issues in real-time. The following points cover why monitoring your systems is considered best practice along with Better Stack-specific benefits.
1. Improved Performance Optimization
By continuously monitoring system metrics, our team can identify performance bottlenecks and optimize resource usage. This approach ensures that applications run smoothly and efficiently, enhancing the overall user experience.
2. Enhanced Security
Monitoring helps us detect unusual patterns or anomalies that could indicate security threats. Early detection of such issues allows our team to respond promptly, safeguarding the system from potential breaches and ensuring compliance with security policies.
3. Data-Driven Insights for Better Decision-Making
Monitoring provides valuable data on system usage and performance which enables teams to make informed decisions. These insights can guide infrastructure improvements, capacity planning, and feature enhancements, aligning technical efforts with business objectives.
4. Cost Efficiency
By identifying and resolving issues quickly, we reduce both monetary and user experience costs associated with downtime.
5. Proactive Issue Resolution
Continuous monitoring enables the early detection of potential problems. With Better Stack, our engineers receive real-time alerts on any issues and have the option to escalate the issue with the entire team for more intensive troubleshooting. This allows our team to address issues quickly before they impact users.
6. Graphs
Better Stack allows our team to build useful dashboards with different graphs for metrics such as availability, request time, etc. This helps visualize system performance and allows us to gain quick insights to make the necessary changes for better system performance.
Automated Testing
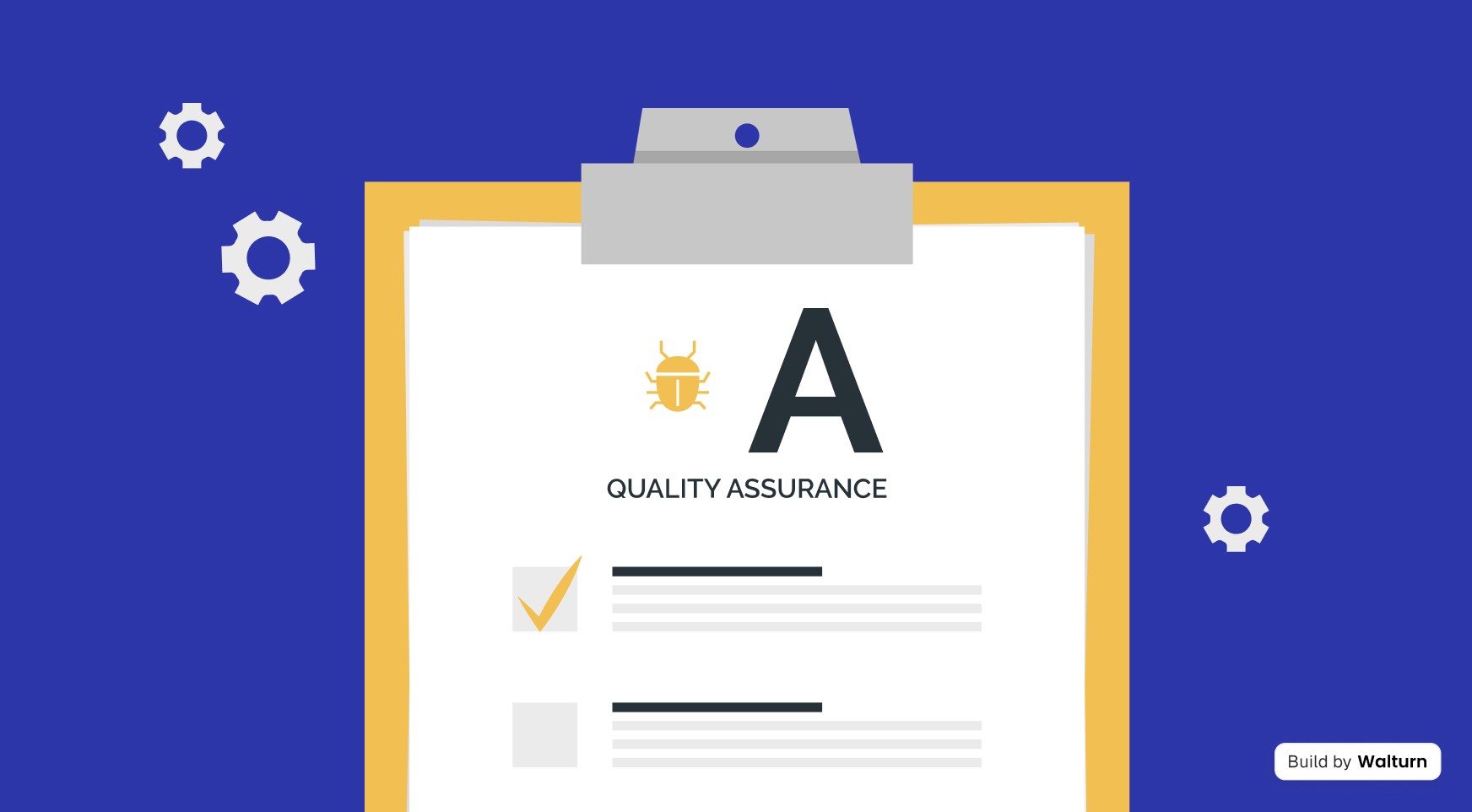
Software testing is an essential part of the software development process to ensure Quality Assurance (QA). It aims to find and fix defects or bugs in the software before it is released to the public. The main goal of software testing includes identifying errors, verifying requirements, and validating user experience.
While manual testing is required to ensure that the software works under different conditions in the real world, some aspects of the process can be automated (for example, integration testing). The ability to automate testing allows software developers to save time and focus on deploying further features. At Walturn, we use Jest and supertest to automate testing on backend systems.
Research studies unveil that automated testing brings significant benefits to the software development process. These include:
1. High Reusability of Test Cases
Automated testing enables the creation of reusable test cases, allowing teams to run the same tests across multiple environments and scenarios without additional effort. This not only saves time but also ensures consistency and reliability in test execution.
2. Improved Test Coverage
Automation allows for more test cases to be executed in a shorter time frame, resulting in better test coverage. This comprehensive coverage ensures that more aspects of the application are tested resulting in reduced errors for deployed applications.
3. Reduced Testing Costs
By minimizing the need for manual intervention, automated testing significantly reduces the costs associated with testing. Once test scripts are developed, they can be run repeatedly with minimal expenses, allowing developers to focus on other activities.
4. Faster Feedback Loop
Automated tests provide quick feedback on code changes, allowing developers to quickly fix issues in the development stage.
5. Consistency
Automated testing eliminates the variability of human error in manual testing, ensuring that tests are executed consistently every time. This provides teams with more confidence in their application to maintain high standards of quality.
However, it is also important to execute automated testing by following best practices. A study, published in 2016, conducted a multivocal literature review to describe when and what should be automated in the software development lifecycle. This allows us to retrieve general best practices across both research studies and gray literature (e.g., blog posts). Some of the best practices include:
1. Focus on Stability
Automate tests for stable features and functions to minimize maintenance and reduce the impact of frequent changes in requirements.
2. Automate Repetitive Tests
Prioritize automating tests that are run frequently, such as regression tests, to decrease manual effort and enhance efficiency.
3. Prioritize High-Value Tests
Automate tests that are critical to business functionality or have a high likelihood of detecting defects to maximize the value of automation.
4. Utilize Automation for Performance Testing
Implement automation for complex scenarios like performance and load testing, which are challenging and time-consuming to perform manually.
5. Consider Test Oracles
Ensure that the expected outcomes of automated tests, known as test oracles, are well-defined and predictable to facilitate effective automation.
6. Assess Automation ROI
Conduct a cost-benefit analysis to evaluate the Return on Investment (ROI) for automating specific tests, focusing on those where automation offers significant time and resource savings compared to manual testing.
7. Utilize Modular Test Designs
Adopt modular test patterns to enhance the reusability and maintainability of automated test cases.
8. Selecting a Tool
Choosing the right tool requires careful evaluation of compatibility, ease of integration, support for necessary testing types, scalability, and cost-effectiveness. The selected tool should align with the team’s skills and fit well into the existing technology stack.
9. Organization Approach
The organization must have a robust approach to process improvements to successfully establish automated testing. This involves having structured processes, effective communication, and a culture that supports continuous improvement.
CI/CD pipelines
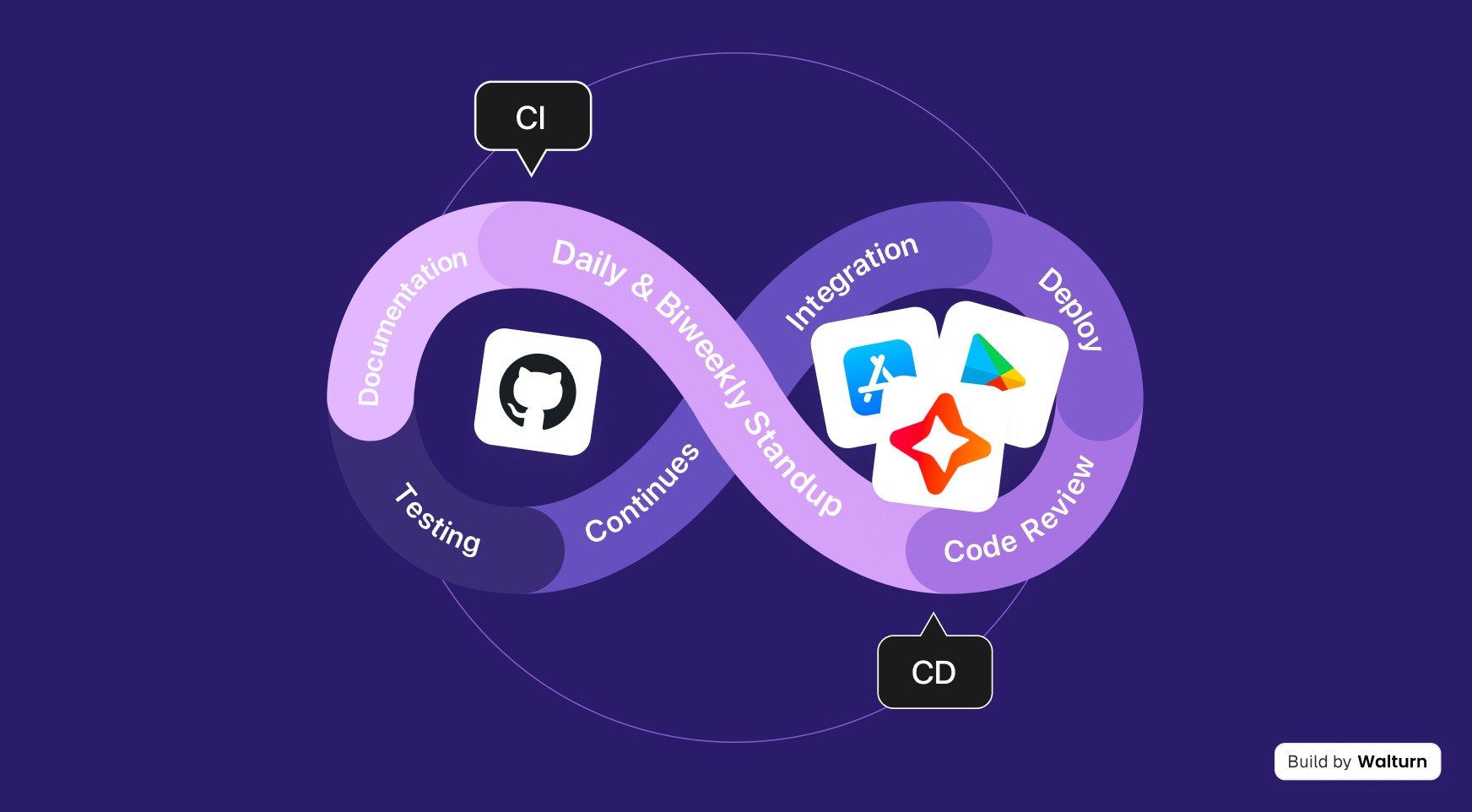
Continuous Integration/Continuous Deployment (CI/CD) is a set of practices and tools designed to automate and streamline the software development lifecycle. It enables teams to deliver more frequent and reliable code changes to projects. Continuous Integration focuses on automatically testing and merging code changes, whereas Continuous Deployment ensures those changes are automatically deployed to the production environments.
The introduction of CI/CD can be traced back to the need for faster and more reliable software delivery processes. Historically, organizations leaned towards waterfall methods, which followed a linear process model. This meant significant time needed to be allocated for the planning phase and delayed the actual development of the software. Moreover, it restricted teams from making changes to the development plan once it had been set. Agile methodologies, introduced in the 2000s, aimed to provide teams with more flexibility through iterative development and feedback loops - which led to the need for CI/CD platforms.
At Walturn, we follow robust agile methodologies to enable the rapid and reliable development of projects. To facilitate CI/CD pipelines, we take advantage of GitHub Actions. The benefits of incorporating CI/CD into the software development process include:
1. Improved Code Quality
CI/CD ensures that the codebase is continuously tested and validated, leading to higher code quality with fewer bugs in production environments.
2. Faster Delivery
By automating the build, test, and deployment processes, CI/CD accelerates the software delivery pipeline, enabling teams to release new features and updates more quickly.
3. Collaboration
CI/CD encourages collaboration among development, operations, and QA teams by providing a unified framework for managing code changes and deployments.
4. Reduced Risk & Cost
Automated testing and deployment reduce the risk of errors and failures in production. This eventually leads to lower costs in the later stages of the project as teams can detect issues in the early stages.
The best practices in CI/CD pipelines include:
1. Automate Testing and Deployment
Implement automation throughout the CI/CD pipeline to ensure consistency, reduce manual errors, and speed up the release process.
2. Implement Incremental Changes
Favor frequent, small code changes over larger updates to make it easier to identify issues and minimize the impact of bugs.
3. Utilize Version Control
Use version control systems to manage code changes and ensure traceability. This facilitates rollback if necessary. Furthermore, implement semantic versioning that follows this format: [MAJOR].[MINOR].[PATCH] for builds.
4. Monitor and Measure Performance
Continuously monitor the CI/CD pipeline and measure key performance metrics to identify bottlenecks for improvement.
5. Security Practices
Integrate security checks into the CI/CD pipeline to identify vulnerabilities and ensure secure code deployment.
6. Deployment Pipelines
Develop deployment pipelines that consist of multiple phases such as testing, staging, and production. This approach allows for systematic verification and validation of code changes at each change, ensuring that only approved code is merged into the production environment.
7. Blue-Green Deployments
Use blue-green deployment strategies to reduce downtime during software releases by maintaining two identical environments: a live blue environment and a green environment for the new version. After testing and preparing the new release, traffic is redirected to the green environment, facilitating seamless updates and enabling quick rollbacks if necessary. This minimizes risks and downtime.
Documentation
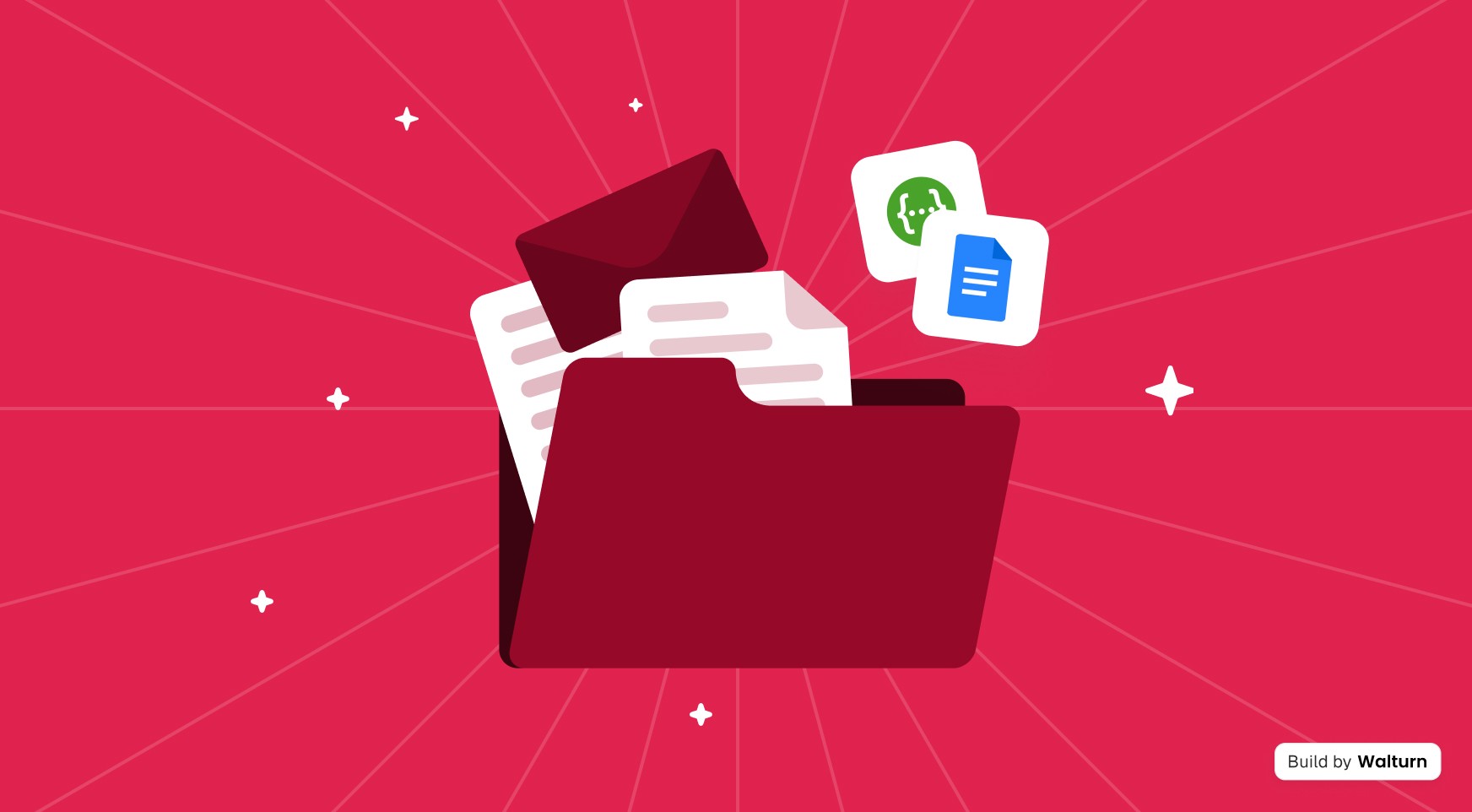
Code documentation includes written text/illustrated information that accompanies the codebase to explain how it operates. In a collaborative environment, where multiple developers work together on a single project, the importance of code documentation cannot be understated.
At Walturn, we utilize Swagger, Google Docs (for complex feature explanations), and descriptive pull requests (PRs) for complex releases. This approach to documentation ensures that all team members have access to comprehensive and up-to-date information about the codebase and its features.
Swagger allows our team to document APIs, providing a standardized and interactive way to describe RESTful web services. Google Docs allows collaborative editing and easy sharing of detailed documentation such as flowcharts, diagrams, and extensive textual descriptions. Lastly, descriptive pull requests serve the purpose of providing a clear and concise summary of the changes being introduced, making code reviews more effective. A well-written PR description includes the rationale behind certain decisions, potential impacts on other parts of the system, and any necessary steps required for testing and deployment.
The benefits of comprehensive code documentation include:
1. Improved Understanding
Well-maintained documentation helps developers understand the codebase, reducing the time needed to get up to speed and enabling them to contribute more effectively.
2. Facilitate Maintenance
Documentation provides a clear reference for future maintenance, making it easier to identify the implications of code changes.
3. Collaboration
Comprehensive documentation ensures that all team members, regardless of their role, can easily access and understand the software’s design and functionality, promoting better collaboration and knowledge sharing within the organization.
4. Supports Onboarding
New team members can rely on existing documentation to learn about the project’s structure and coding standards, speeding up the onboarding process.
To ensure high-quality and comprehensive code documentation, Walturn follows these best practices:
1. Keep Documentation Up-to-Date
Regularly update the documentation to reflect changes in the codebase, ensuring that it remains accurate and useful. Be sure to delete dead documentation. These comments can misinform and slow down engineers on the team.
2. Use Clear and Concise Language
Write documentation in clear and straightforward language to make it accessible to all readers, including those who may not be familiar with the codebase.
3. Don’t Repeat Yourself (DRY)
Apply the DRY principle to code documentation by ensuring that information is not repeated unnecessarily across different documents. Instead, maintain a single, authoritative source of information for each aspect of the codebase. However, when writing README files, this principle may be overlooked to avoid potential errors. For example, when writing instructions to run different flavors of the application.
4. Examples and Code Snippets
Include practical examples and code snippets to illustrate complex concepts and provide real-world context.
5. Organize Documentation
Structure documentation logically, using headings, subheadings, and a consistent format to make it easy to navigate.
Libraries and Packages
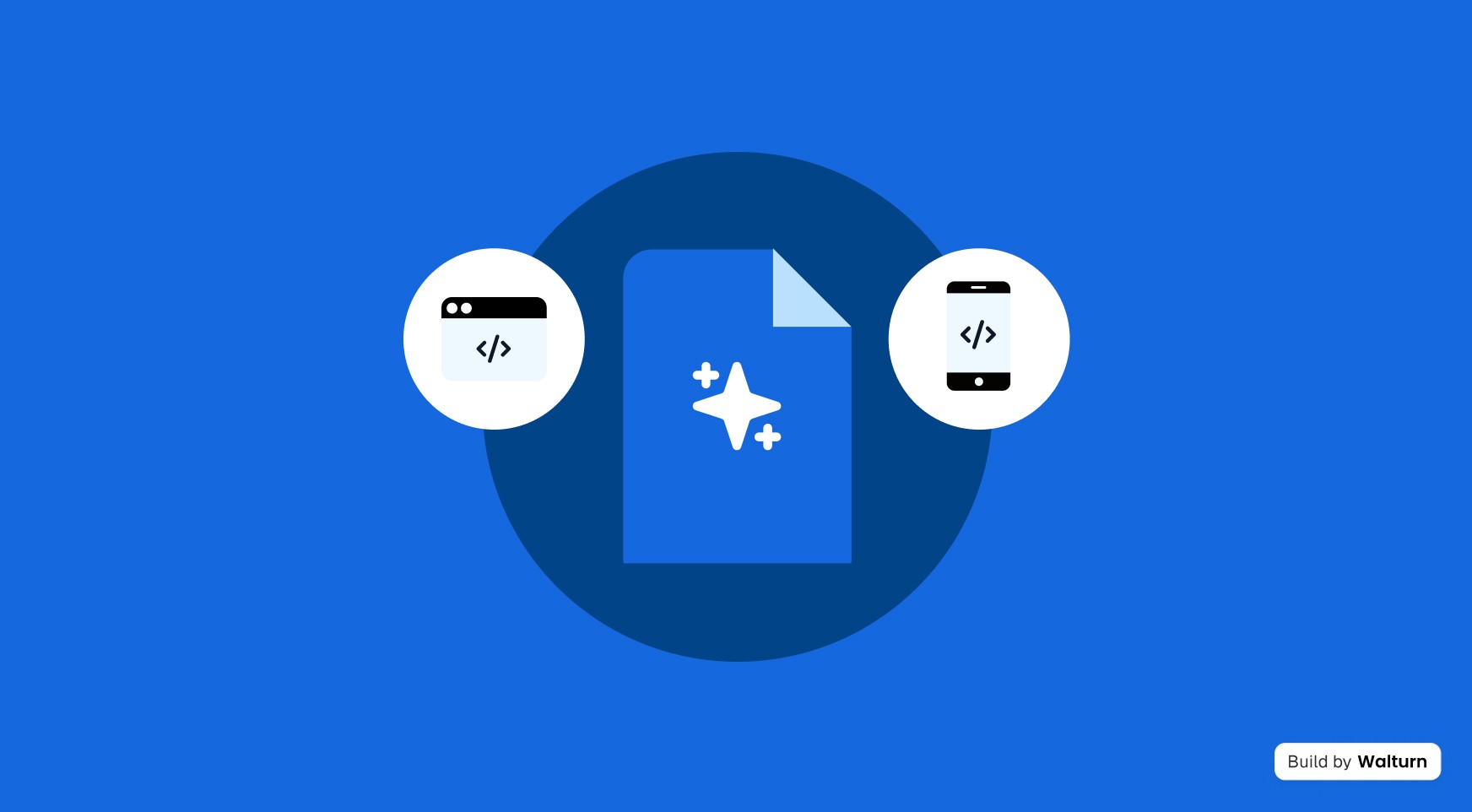
A package is a collection of related modules that are grouped whereas a library is a collection of pre-written code that provides specific functionality or services. Both packages and libraries are essential when writing high-quality code. These allow developers to maximize code reusability.
At Walturn, we encourage developers to create custom packages when working on large projects. This introduces Separation of Concerns into our codebase, enhancing the reusability and maintainability of our codebase. Additionally, reliable third-party packages and libraries allow our team to speed up development. The benefits of utilizing libraries and packages include:
1. Code Reusability
Packages and libraries provide code that developers can reuse across different projects, reducing the need to write code from scratch. This accelerates development and ensures consistency in implementing common functionality.
2. Efficiency
By utilizing libraries, developers can focus on building unique features rather than spending time on routine tasks. This allows teams to allocate resources to more critical aspects of the project.
3. Simplified Maintenance
With well-organized packages, maintaining a codebase becomes more manageable. Changes and updates can be made to specific packages without affecting other parts of the application.
4. Access to Community Support
Popular libraries and packages are backed by active communities that provide documentation, tutorials, and support. This involvement helps developers quickly resolve issues and stay updated on best practices.
However, when using third-party libraries and packages, it is important to conduct proper research. Walturn follows these best practices for incorporating third-party libraries:
1. Carefully Select Packages
When using third-party packages, it is essential to carefully select and manage them to ensure they integrate smoothly with your project. Start by evaluating the package's fit for your specific use case.
Before adding a new package, evaluate its popularity, maintenance status, and community support. Check how many people are actively using it as this can indicate its reliability and community acceptance.
2. Testing Packages
Thoroughly test each package to ensure it works as expected in your project. Testing the package is essential to understanding its behavior and preventing the introduction of breaking changes.
3. Lock Versions
Lock the versions of your dependencies in your codebase to prevent breaking changes from future updates. Use exact versions for critical packages to ensure consistency across different development environments and CI/CD pipelines. Regularly check for updates to your dependencies and apply them in a controlled manner.
API Versioning
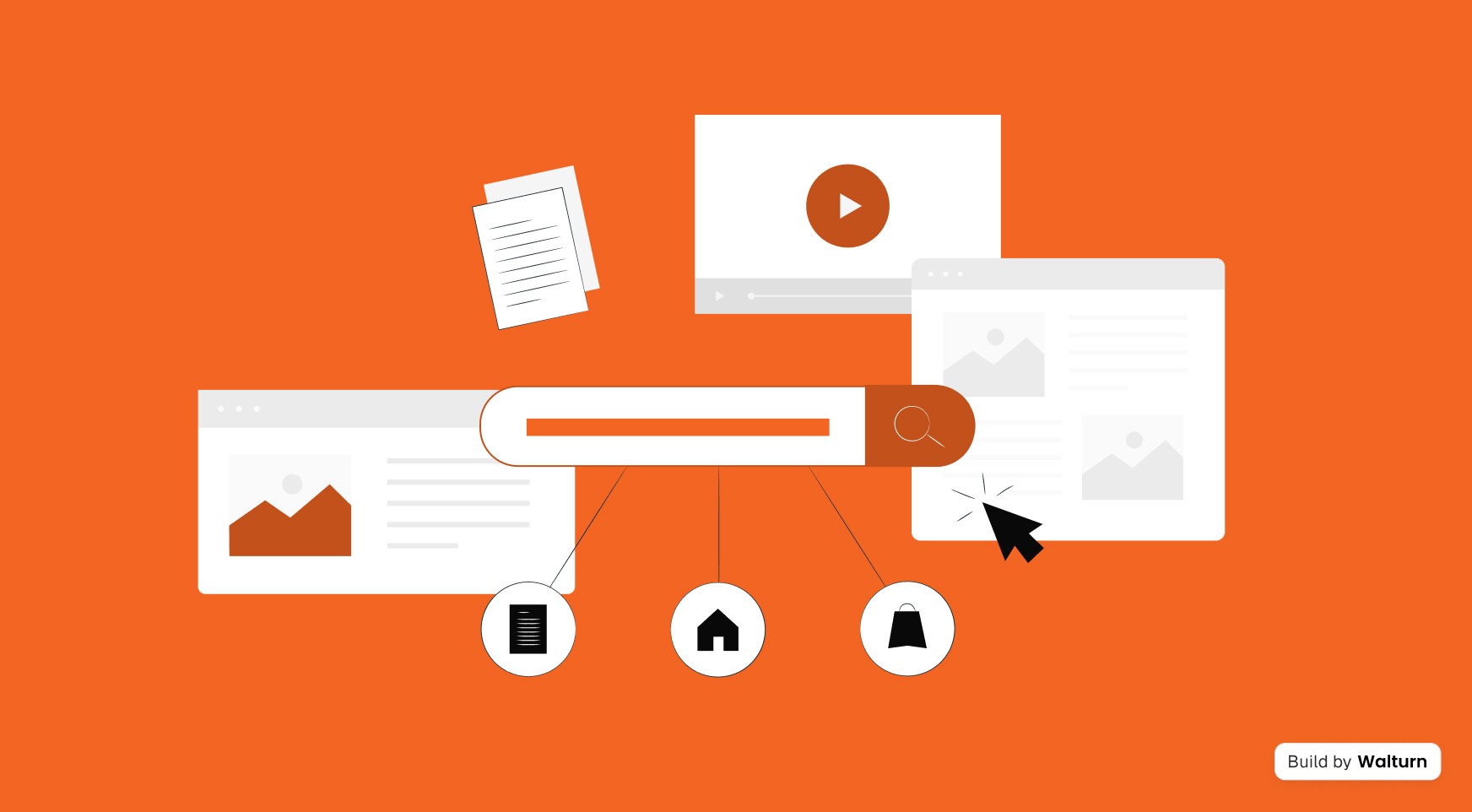
Managing updates to an API contract is a major challenge when exposing services. Clients may not want to update their applications when the API changes, so having an effective versioning strategy is crucial for improving APIs without breaking client applications. There are four common ways to version a REST API. Content negotiation offers more granular control but has a higher implementation cost. Other methods, such as URI paths, query parameters, and custom headers, are easier to implement but limit flexibility when introducing breaking changes. Choosing the right strategy for your product ensures your API remains robust and client-friendly.
Once you have chosen which type of API versioning you want to use, a well-thought-out approach to API versioning is essential to avoid negative consequences for both API consumers and producers.
Here are some best practices that we follow at Walturn to ensure the success of an API versioning strategy:
1. Design with Extensibility in Mind
During the design process, it is important to avoid using data types prone to breaking changes, such as booleans and arrays of atomics. By considering versioning strategically, a more robust and adaptable API can be created.
2. Know Your Consumers
Understanding how consumers use the API is crucial. Awareness of unexpected implementations, such as accessing properties by index rather than name, helps account for the invisible API contract and informs decisions about changes.
3. Include a Versioning Policy in Your Terms of Service
Defining what constitutes a breaking change and communicating this to consumers is essential. The terms of service should include details on when consumers will be warned about upcoming changes and how long they will have to migrate to a new version. This practice is especially important for partner and public APIs, particularly those that are monetized.
4. Decouple Implementation Versioning and Contract Versioning
It is important to separate the versioning of the API’s implementation from its contract. For instance, if the backend is rewritten in a different language but the contract remains unchanged, a new API version should not be released. This distinction helps maintain stability for consumers.
5. Test Thoroughly
Versioning is a major event in an API’s lifecycle, so thorough testing is essential. Testing during development and deployment ensures that the new version works as expected and does not introduce new issues for consumers.
6. Plan for Deprecation
A clear deprecation policy should be developed and communicated to consumers. Monitoring the usage of old versions and ensuring that clients have sufficient time to transition to the new version is crucial. Careful planning and communication can reduce the risk of surprises and ensure a smooth transition.
7. Enable Backward Compatibility
Striving to make new API versions backward compatible can reduce the need for creating new versions and simplify the migration process for clients. Techniques for achieving backward compatibility include adding new resources, methods, parameters, headers, or fields without removing existing ones; providing default values or fallback options for new features; using aliases for renamed or deprecated elements; and indicating compatibility with multiple versions using version ranges or wildcards.
8. Refresh API Documentation to Reflect New Versions
Clear and accurate documentation for each API version is essential. A consistent naming scheme for version identifiers should be used, and changelogs or release notes that summarize changes should be provided. Examples and code snippets for each version should be included, along with links to related resources or other versions.
9. Adapt API Versioning to Business Requirements
Aligning the versioning strategy with business goals is important. The frequency of new version releases and the support duration for old versions should be determined, and effective communication with clients about new versions should be maintained. Incentivizing or encouraging clients to upgrade to new versions is also beneficial.
10. Put API Security Considerations at the Forefront
All API requests and responses should use HTTPS and SSL/TLS encryption. Robust authentication and authorization mechanisms should be implemented, and data integrity and confidentiality should be protected with encryption, hashing, or signing techniques. Rate limiting, throttling, or caching should be used to prevent abuse or overload of the API.
By following these best practices, API versioning can be managed effectively, ensuring a smooth experience for both API producers and consumers.
GitHub Setup
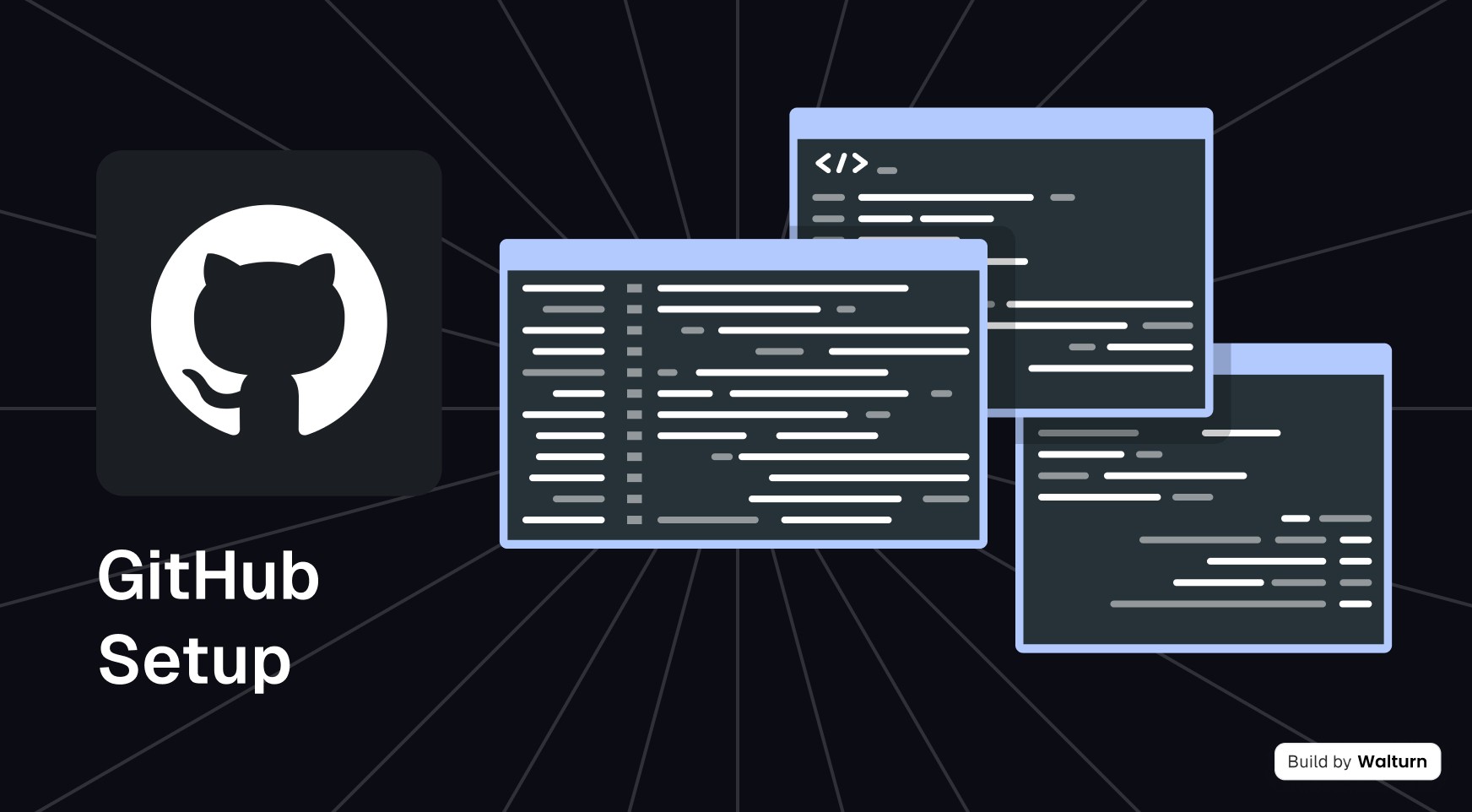
GitHub is a widely used web-based platform that offers a free and integrated environment for hosting source code, documentation, and project-related web content, primarily for open-source projects. It also provides paid plans for private repositories, catering to individuals and businesses, along with free plans for research and educational use.
At its core, GitHub relies on the well-known and open-source version control system Git, originally designed by Linus Torvalds for developing the Linux kernel. GitHub extends beyond simple source code hosting by providing a dynamic and collaborative environment, often referred to as a social coding platform, supporting peer review, commenting, and discussion. This makes GitHub an essential tool for a diverse range of projects, from individual endeavors to global collaborations.
At Walturn, we use GitHub for all our development projects and make sure to follow the following best practices:
1. Repository Name
Choosing a name for a GitHub repository requires careful consideration to ensure it is descriptive, readable, consistent, contextual, future-friendly, extensible, reusable, and brief. Here are key guidelines for selecting an effective name:
Follow a Clear Naming Convention: Establishing a consistent naming convention across all your projects promotes organization and clarity. This approach allows for easy identification of the repository’s purpose and content at a glance, facilitating quick searches and retrievals. It also supports a standardized approach across teams, making workflows more efficient and enabling effective automation.
Naming for Website Projects: For website-related projects, naming the repository after the domain is practical, such as domain.com.git or sub.domain.com.git. This approach provides immediate context and relevance to the project’s purpose.
Use Kebab Case: For other types of projects, use lowercase letters and dashes (e.g., star-wars.git). This style, known as Kebab case (kebab-case), replaces spaces with hyphens and is familiar due to its similarity to CSS properties and HTML attributes. Avoid CamelCase to minimize confusion and issues with auto-completion.
Descriptive and Technology-Specific Names: Incorporate descriptive names that reflect the technology stack or purpose of the repository. Examples include customer-support-ticketing-system or image_processor_python. Indicating the use case with names like authentication_lib or payment_api_service enhances clarity.
Avoid Special Characters: Stick to simple alphanumeric characters and hyphens in your repository names, as in Kebab case. Avoid special characters to ensure compatibility and simplicity.
Be Specific: Choose specific names to avoid future confusion. For instance, use wildlife-locator-rest-service instead of a generic name like locator-service.
Avoid Organizational Names: Refrain from using names of organizations, departments, or teams, as these may change over time and cause stability issues. Instead, provide context without overloading the name. For example, using an abbreviation like “BC” for “Province of British Columbia” is acceptable, but avoid starting every repository name this way to simplify sorting and searching.
Exclude Version Numbers: Do not include version numbers in repository names. Instead, manage versions using GitHub’s release features, which provide a more organized and scalable approach to versioning.
By following these guidelines, you ensure repository names are clear, effective, and conducive to efficient team navigation and collaboration. This leads to better project management, enhanced team cohesion, and significant time savings.
2. README
A README file is the first point of contact for visitors to a repository, making it essential for it to reflect the project. It should communicate the project’s purpose, why it is useful, how users can get started, where they can seek help, and who maintains it. A comprehensive README sets expectations and manages contributions by providing essential information and guidelines.
Research indicates that a GitHub repository should include a detailed README file in the root directory, complemented by domain-specific documentation. To support first-time users and ensure data standards are clear and reusable, a README should include the following subheadings:
About: An overview of the project.
Getting Started: Instructions for setup and installation.
How to Contribute: Guidelines for contributing to the project.
License: Information about the project’s licensing.
Funding and Acknowledgments: Details on project funding and recognition of contributors.
Recommended Citation: Citation instructions for open-source projects.
At Walturn, we emphasize the importance of a well-crafted README to ensure project continuity and quality. Our best practices include:
Clear Project Title: Provide a straightforward title that reflects the project’s purpose.
Concise Description: Offer a brief yet informative summary of what the project does.
Table of Contents: Include a table of contents for easy navigation.
Detailed Installation and Setup Instructions: Guide users through setting up the project.
Project Structure Overview: Explain the organization of the project files and directories.
Usage Examples: Provide examples that demonstrate how to use the project.
Contribution Guidelines: Outline how others can contribute to the project.
Testing and Quality Assurance Details: Describe the testing processes to ensure the project’s quality.
Deployment Instructions: Offer guidance on how to deploy the project.
This structured and detailed documentation helps team members quickly get up to speed and maintain consistency across the project, even as team members change.
3. Managing Releases
Effective release management is crucial for maintaining project stability and ensuring smooth deployment. Releases bundle and deliver project iterations to users, and can include release notes, contributor mentions, and binary files. Releases can be managed via the GitHub web interface or the GitHub API.
Tags in Git are references to specific commits, often used to mark releases or milestones. They maintain a fixed position in the project’s history. The two types of tags are:
Lightweight Tags: Simple pointers to a commit, lacking additional metadata.
Annotated Tags: Include metadata such as the tagger’s name, email, and date, providing a more comprehensive record.
When drafting a new release, the version tags you use with your commits will determine which changes get published in the release. Since tags are so integral to the process, it is essential to follow best practices when tagging your commits:
Be Descriptive: Use clear, descriptive names.
Follow Semantic Naming Convention: Multiple studies agree that tags in versioning should follow the semantic versioning format (X.Y.Z): X represents the major version, indicating significant changes or breaking updates; Y is the minor version, showing backward-compatible feature additions; and Z is the patch version, which includes bug fixes or minor improvements. This system helps maintain clear documentation of changes and ensures easy version recovery and comparison. This is also the recommended versioning convention endorsed by GitHub.
Prefer Annotated Tags: Use for public releases or significant milestones.
Tag Strategically: Mark important points in the project’s history.
Keep Tags in Sync: Regularly push tags to the remote repository to maintain collaboration.
Consistent tagging ensures that release processes can be smooth. In conclusion, the best practices for GitHub releases would be to:
Use Branching for Releases: Employ branching strategies in Git to effectively manage and identify different releases.
Utilize GitHub’s “Release” Feature: Save a snapshot of the repository at a specific point in time and assign a semantic version number.
Assign Clear Version Numbers: Ensure users can easily identify when to migrate data or locate previous documentation versions by following the best tagging practices.
Security and Privacy
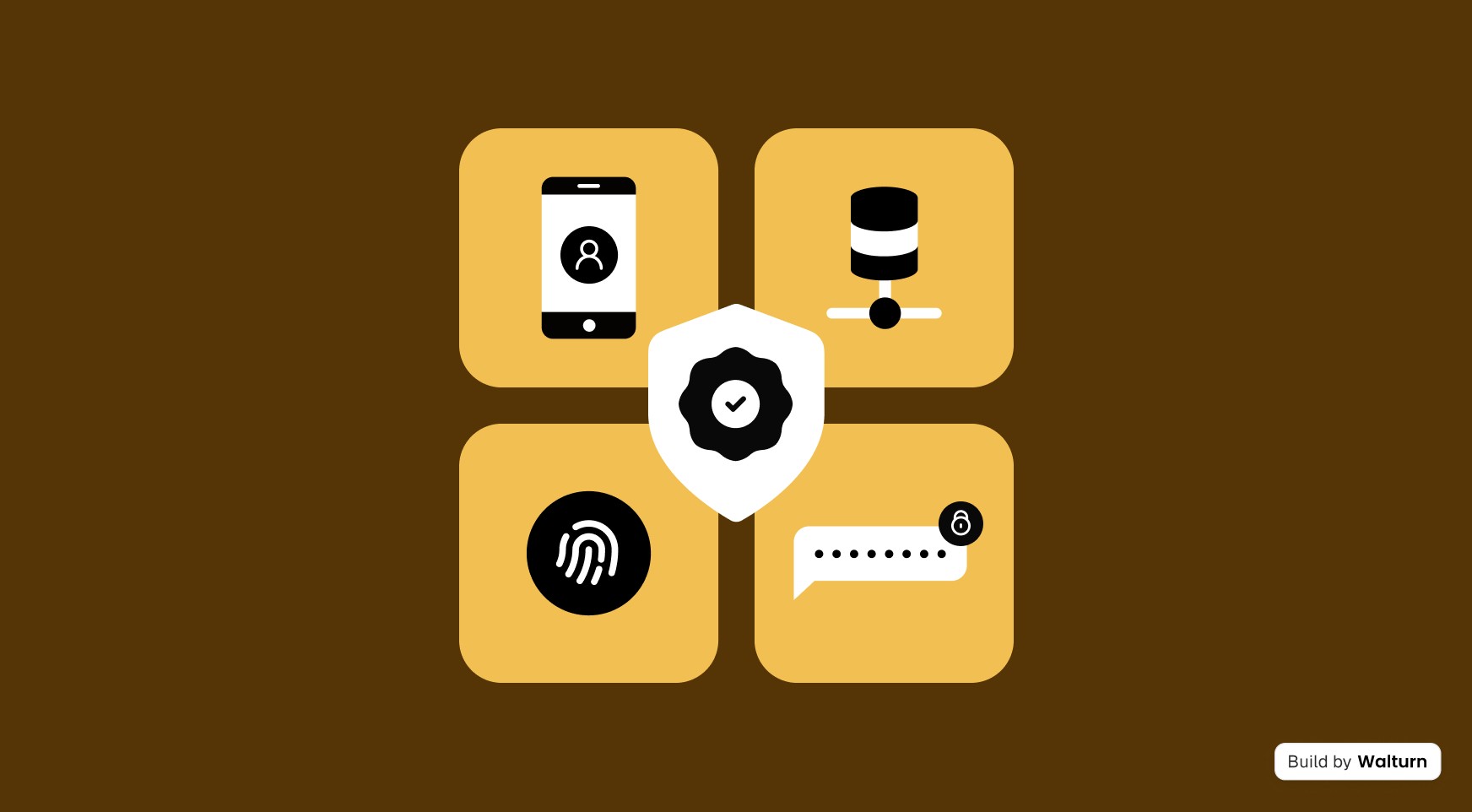
In today’s digital age, embedding security and privacy within the Software Development Life Cycle (SDLC) is increasingly vital. The concept of Secure Software Engineering (SSE) encompasses the entire process of designing, building, and testing software to ensure it is resilient against potential threats. However, many businesses mistakenly view security as an afterthought—a task to be addressed post-development. This approach can lead to significant vulnerabilities, resulting in financial losses, compromised data, and reputational damage.
Security and privacy are not just technical requirements; they are essential components that must be integrated into the SDLC to produce high-quality, trustworthy software. Neglecting these aspects can have severe consequences, including exposure to hidden threats, financial and data losses, and diminished trust among users and stakeholders.
According to research, the benefits of including security and privacy measures in the SDLC include:
1. Enhanced Software Quality
Integrating security from the beginning ensures that the software is robust and resilient, reducing vulnerabilities that could be exploited by malicious actors.
2. Reduced Development Costs
Addressing security issues during the early stages of development is more cost-effective than fixing them post-deployment. It minimizes the need for extensive rework and patching.
3. Increased User Trust
Secure software fosters confidence among users, stakeholders, and customers, which is essential for the software's success and widespread adoption.
4. Compliance with Regulations
Incorporating security and privacy measures ensures that the software complies with industry standards and legal requirements, avoiding potential legal liabilities.
5. Protection of Sensitive Data
By embedding security practices into the SDLC, organizations can better protect sensitive data, ensuring that confidentiality and integrity are maintained throughout the software's lifecycle.
6. Proactive Threat Mitigation
Secure SDLC processes enable the identification and mitigation of security threats during the design and development phases, reducing the risk of exploitation.
7. Long-term Maintainability
Secure software is easier to maintain and update over time, as it is built with a solid foundation that considers future security challenges.
8. Business Continuity
Ensuring software security and privacy is critical to maintaining business operations, especially in the event of cyberattacks or data breaches. It helps in minimizing downtime and recovering swiftly.
At Walturn, we are committed to incorporating the following security and privacy best practices into our backend development process:
1. Key Storage
Storing unencrypted secrets and API keys directly in .git repositories, even private ones, poses significant security risks. It is a common but dangerous misconception to consider private repositories as secure vaults for such sensitive information. Private repositories are attractive targets for malicious actors because they often contain secrets.
Additionally, the nature of Git leads to repository sprawl: repositories get cloned, forked, and accessed by new developers, spreading any hard-coded secrets throughout all derived repositories. If a secret is committed to a repository, whether private or public, it should be assumed compromised.
To mitigate these risks, using local environment variables is recommended. Environment variables are dynamic objects whose values are set externally to the application, making them easy to rotate without altering the application code. This practice keeps sensitive data out of the source code and reduces the likelihood of such information being checked into a repository. Overall, using environment variables provides a simple, clean, and secure method for handling sensitive data.
2. Local Development Environments
In local development environments, it is necessary to avoid using wildcard commands like git add * or git add . as they can inadvertently include files that should not be committed to the repository. These may include generated files, configuration files, and temporary source code.
Instead, developers should add each file by name and use git status to review tracked and untracked files. This approach ensures complete control and visibility over what files are committed, reducing the risk of unwanted files entering source control and preventing API key leaks. Although this method takes more time and can occasionally result in missed files, committing early and often helps manage file history effectively and reduces the temptation to use wildcard commands.
Additionally, use a comprehensive .gitignore file to prevent sensitive files from being committed to the repository. This file should exclude environment variable files such as .env, configuration files like .zshrc or application.properties, files generated by other processes (e.g., application logs or unit test reports), and files containing real data such as database extracts.
By following these techniques, developers can ensure that sensitive information and unnecessary files do not get committed to the repository, maintaining a clean and secure local development environment. This is especially important when storing keys as local environment variables.
3. Anti SQL Injection
SQL injection is a common web attack mechanism where attackers exploit vulnerabilities in data-driven applications to insert malicious SQL statements into input fields, enabling unauthorized access to the underlying database. These vulnerabilities arise due to improper coding practices, allowing untrusted input to interact directly with the database. SQL injection can lead to theft, modification, or destruction of sensitive data, elevation of privileges, and further network exploitation.
To protect against SQL injection, developers should use parameterized database queries with bound, typed parameters and parameterized stored procedures across various programming languages.
Essentially, parameterized database queries help SQL see that certain parameters are data, not executable code. Instead of embedding values directly into code, they are provided at runtime.
Without parameterization, an SQL query from another programming language might look like this:
With parameterization, it looks like:
The actual parameters are supplied later when the query is being executed.
For PostgreSQL, the PREPARE and EXECUTE keywords are used:
Regularly updating all web application components and adhering to the principle of least privilege for database accounts are highly recommended. The principle of least privilege means granting accounts only the minimal permissions necessary to perform their functions. Additionally, avoid using shared database accounts, validate user inputs for expected data types, and configure proper error reporting to prevent attackers from leveraging technical details.
These practices significantly reduce the risk of SQL injection vulnerabilities.
4. ORM
Object Relational Mapping (ORM) is a technique that connects object-oriented programming (OOP) languages with relational databases, facilitating CRUD (Create, Read, Update, Delete) operations without directly writing SQL queries. ORM tools simplify database interactions for OOP developers by speeding up development time, reducing costs, handling complex database logic, enhancing security by mitigating SQL injection risks, and requiring less code.
Prisma ORM is a modern ORM that addresses the shortcomings of traditional ORMs. Traditional ORMs map database tables to model classes, often leading to object-relational impedance mismatch issues. In contrast, Prisma ORM uses a declarative schema as the single source of truth for database and application models, enabling type-safe data operations through Prisma Client. This approach simplifies data querying, making it more natural and predictable by returning plain JavaScript objects, thereby eliminating the complexities of managing model instances.
Node postgres or 'pg' is a popular package for interfacing with PostgreSQL databases in Node.js environments. It offers flexibility for executing complex queries that ORMs may struggle with, while providing essential security features like protection against SQL injection through parameterized queries, and built-in connection pooling for stability.
5. Stateless JWT Authentication
JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. Encoded as a JSON object, a JWT contains information to identify a user and token validity and is cryptographically signed to prevent tampering. JWTs are one of the industrial standards for implementing stateless authentication.
Stateless authentication, facilitated by JWTs, allows users to access services without repeatedly using their username and password. This method offers several benefits, including lower server overhead since session data is stored on the client side, ease of scaling as any backend server with the shared private key can validate the token, and enhanced security and compliance, particularly for industries with stringent data regulations, as sensitive credential information remains on-premise.
6. Password Hashing
User passwords should never be stored as plain text or using outdated hashing methods, since that puts them at risk. Employ industry-standard hashing techniques to ensure passwords are securely handled and never accessible at any stage of the application.
Error Handling
Effective error handling is crucial in backend development. Proper error handling ensures stability, graceful responses to unexpected issues, and meaningful feedback for users and developers.
Walturn follows these best practices for implementing robust error-handling mechanisms:
1. Define Clear and Consistent Error Codes
Establish a comprehensive set of error codes that clearly define various types of errors. This approach simplifies issue identification for both developers and automated systems, promoting a standardized method across the application.
2. Use HTTP Status Codes Effectively
Apply appropriate HTTP status codes to indicate the outcome of API requests. Familiarize yourself with standard codes like the following to enhance clarity in communication between backend and frontend components:
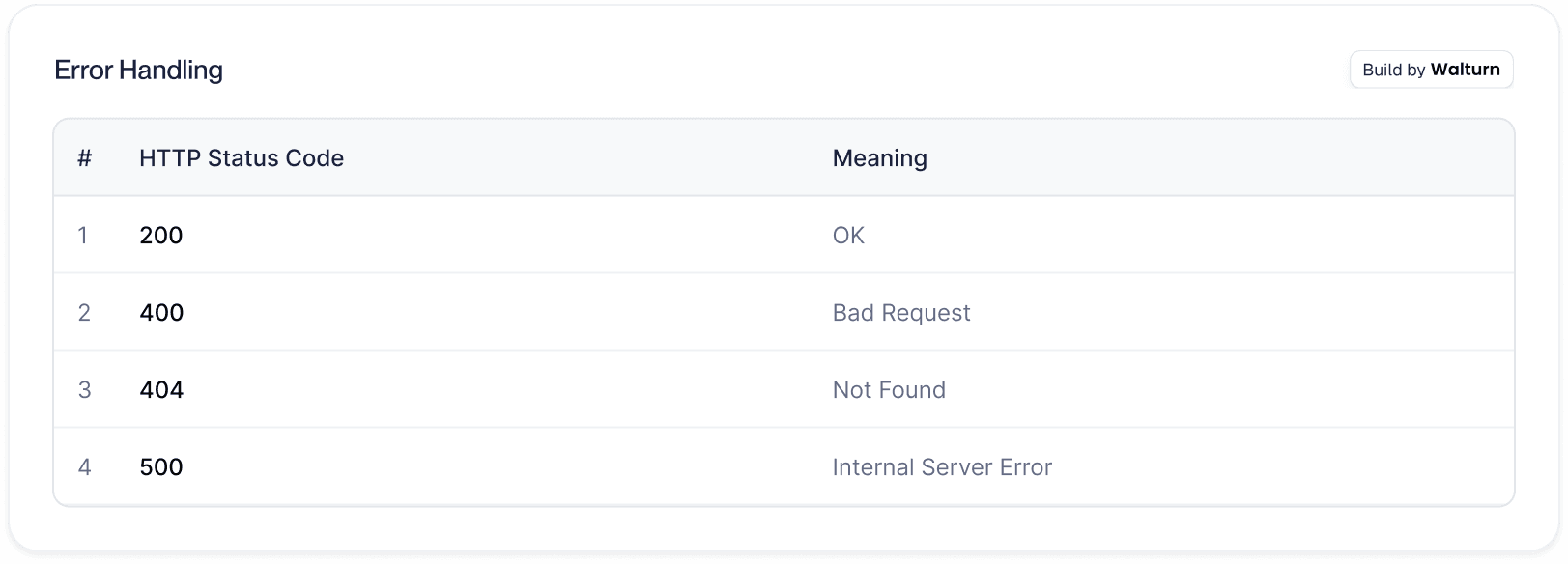
3. Provide Descriptive Error Messages
Ensure that error messages are both informative and user-friendly. Messages should help users understand what went wrong and guide them toward potential solutions while avoiding the disclosure of sensitive information to maintain security.
4. Log Errors Appropriately
Implement robust logging mechanisms to capture relevant error details, such as timestamps, user context, error codes, and stack traces, while respecting privacy and security. This practice aids in diagnosing issues during development and supports post-mortem analysis for production incidents. Use different log levels to balance detail with performance and employ logging services in production to manage and analyze log data.
5. Proper Use of Try/Catch
Employ try/catch blocks to handle errors that can be managed effectively. Avoid catching errors that cannot be handled, as it is often better to let them propagate to a global error handler.
This is an example in NestJS which overrides the entire response body and provides an error cause:
6. Use Database Transactions
When performing complex operations in database management, use database transactions to ensure that all actions are executed as a single, atomic unit. This approach guarantees that either all operations are completed successfully, or none are, as the transaction will automatically roll back any changes if an error occurs.
By doing so, you prevent data inconsistencies and corruption, making transactions essential for robust error handling. Additionally, using transactions helps enforce the ACID principles—Atomicity, Consistency, Isolation, and Durability—ensuring your database operations remain reliable and consistent.
7. Handle Validation Errors Early
Validate user input at the earliest point in the backend processing pipeline. Early validation reduces the impact on downstream components and enhances overall system efficiency.
8. Document Error Handling Procedures
Document error handling procedures thoroughly, including expected behaviors for each error code and troubleshooting steps for common issues. Comprehensive documentation supports team collaboration and facilitates the onboarding of new developers.
9. Test Error Scenarios Rigorously
Include extensive testing of error scenarios in your test suites. Conduct unit tests, integration tests, and end-to-end tests to simulate various error conditions and edge cases, ensuring the backend system responds appropriately and reducing the likelihood of production issues.
10. Monitor and Analyze Errors in Production
Implement real-time monitoring solutions to track and analyze errors in a live production environment. Effective monitoring allows teams to address issues proactively, identify patterns, and continuously improve error-handling mechanisms.
In summary, robust error handling and logging are essential for backend development. A well-designed error-handling framework helps systems respond to unexpected issues gracefully and provides valuable insights for ongoing improvements. Investing time in establishing effective error-handling practices will lead to more stable and reliable applications.
Combined Error Analytics
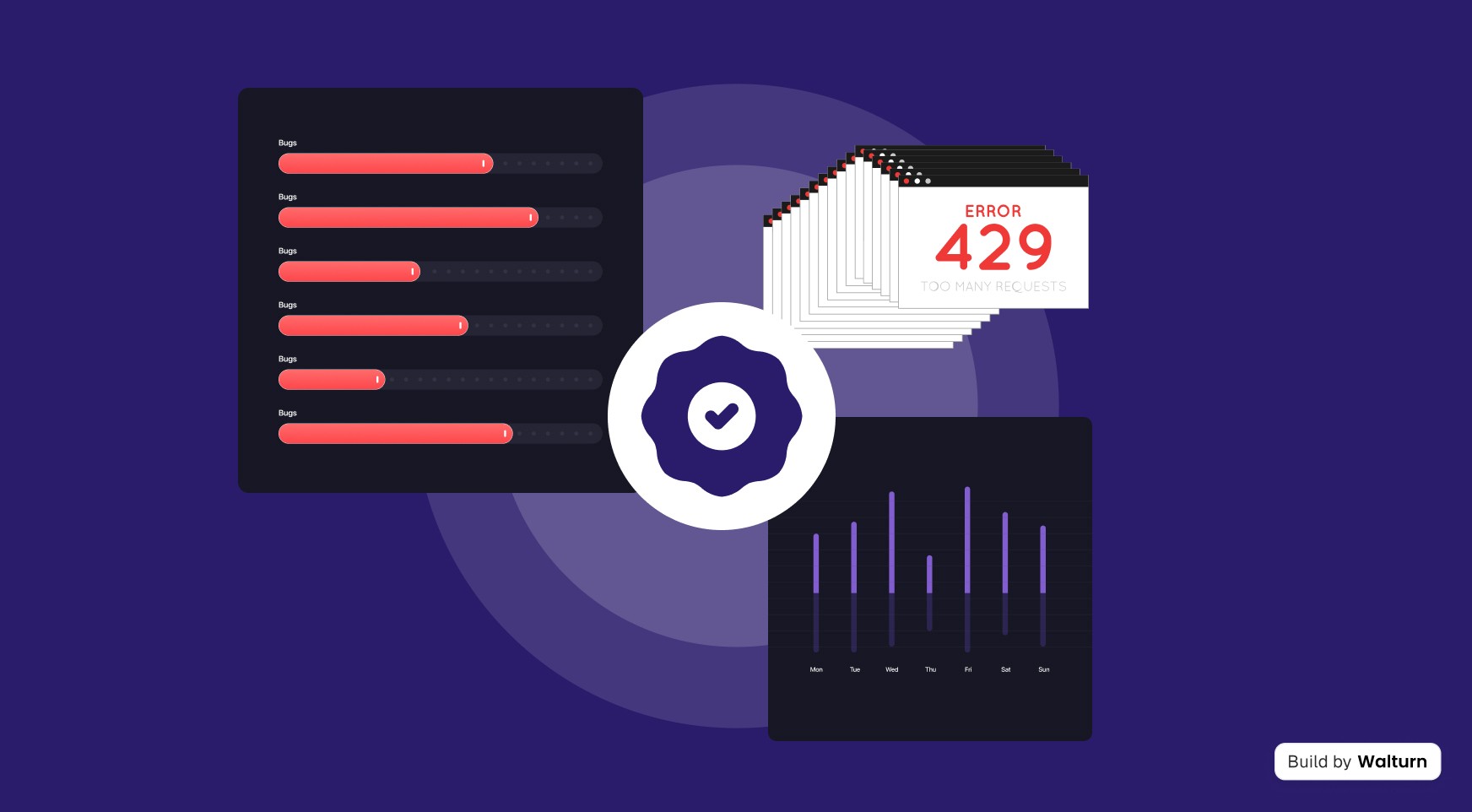
Modern software engineering trends favor decoupling large monolithic systems into microservices to improve scalability, efficiency, and maintainability. However, the increased complexity of these Fine-Grained Distributed Systems (FGDS) presents challenges in anomaly detection. Operators use state observation techniques like monitoring, logging, and end-to-end tracing to manage this complexity. The benefits of these methods are:
1. Real-Time Performance Insights
Provide continuous measurement of system metrics like CPU usage and network latency.
2. Event Tracking
Log discrete events for troubleshooting and auditing.
3. Execution Flow Visibility
Trace the flow of requests through different system modules, helping identify performance bottlenecks.
4. Causality Preservation
Maintain relationships between events across distributed components and locations.
5. Anomaly Detection
Help detect and diagnose issues by analyzing execution traces for unusual behavior.
6. Improved Troubleshooting
Facilitate quick identification and resolution of issues by highlighting problematic areas.
Despite their effectiveness, these techniques face limitations due to the sheer volume of data and the manual effort required to identify anomalies. Distributed tracing tools often require operators to manually query and analyze traces, making it challenging to pinpoint issues swiftly. To address these challenges, there is a pressing need for automation in tracing analysis to automatically detect anomalies and provide actionable insights, streamlining the process and enhancing system reliability.
Application Performance Monitoring (APM) tools that consolidate and automate these processes are a good investment. Some best practices that these tools should include are:
1. Automate Tracing Analysis
Implement automated tracing analysis to convert raw trace data into higher-order constructs, providing actionable insights for anomaly detection.
2. Real-Time Monitoring
Continuously measure infrastructure metrics such as CPU usage, network latency, and storage performance to maintain system health.
3. Event-Triggered Logging
Use logging to capture discrete events and provide an overview of system activities, enabling quick identification of issues.
4. Distributed Tracing
Employ distributed tracing to maintain causality relationships across multiple components, helping trace the flow of requests through the system.
5. Visibility into Performance
Ensure comprehensive visibility into every layer of the application and its infrastructure to detect and resolve performance issues effectively.
6. Centralized Issue Management
Aggregate errors and performance issues across multiple projects into a single view for efficient triage and resolution.
7. Custom Grouping and Filtering
Use custom grouping algorithms and filters to manage and prioritize events, reducing noise and focusing on critical issues.
8. Ownership Rules and Alerts
Implement ownership rules to assign issues to responsible team members and set context-specific alerts to ensure timely responses to performance problems.
Walturn uses the APM tool Sentry to implement these practices. Sentry enhances application performance monitoring by integrating error monitoring with tracing to provide comprehensive insights into application performance. It automates user management with SCIM and SSO, enables detailed event grouping and filtering, and supports issue ownership and alerting to streamline resolution processes. Sentry’s trace view and trace navigator tools offer visualizations of transaction flows, helping teams quickly identify and resolve performance bottlenecks. By consolidating communication and providing advanced querying and dashboard capabilities, Sentry ensures that teams can effectively manage and improve their application’s health and performance.
Use of AI
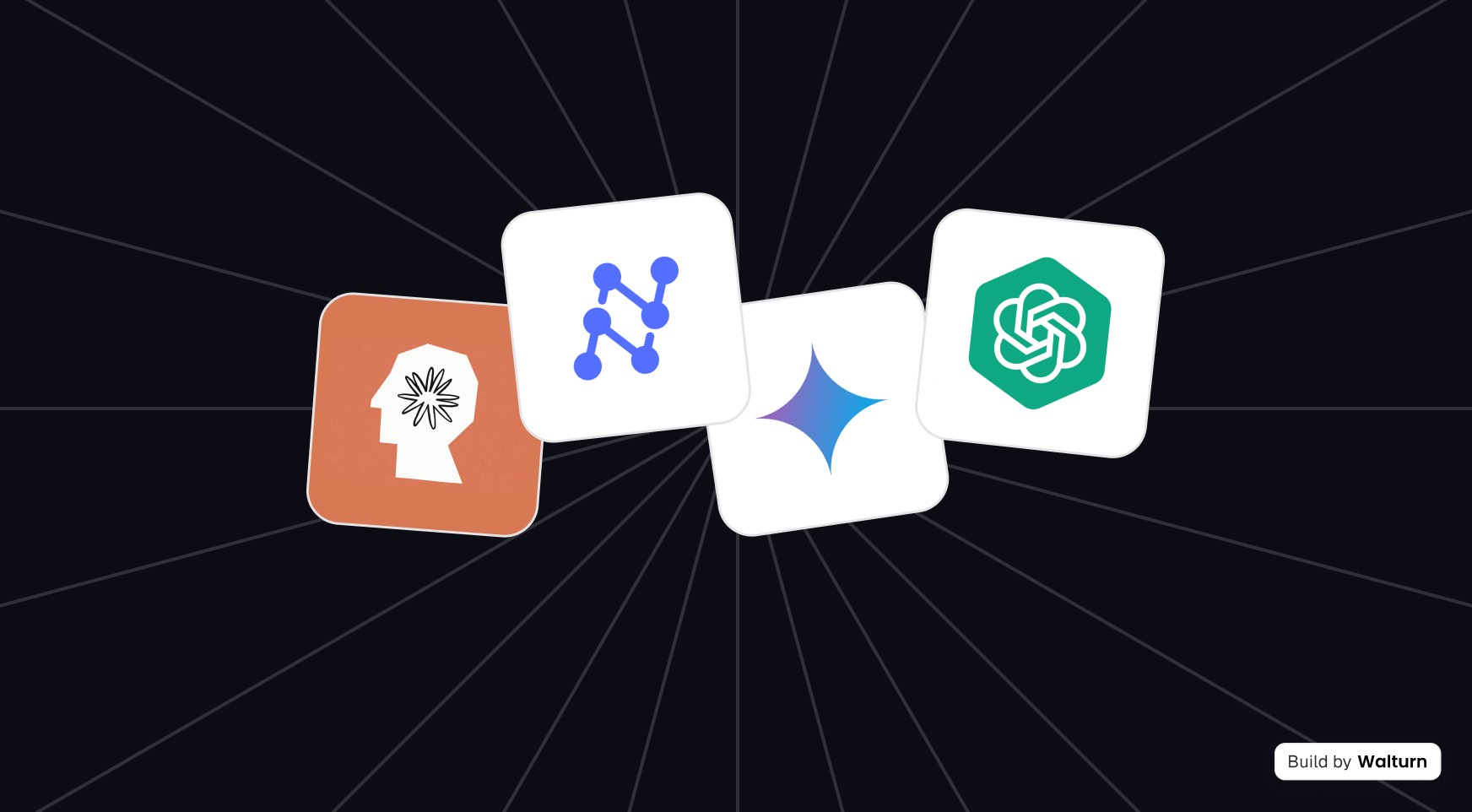
The recent boom of generative AI has put a spotlight on incorporating AI into the software development cycle and business strategies. A recent study conducted a systematic review of the strategic use of AI in the business world, and recommended that AI be used for the following focus areas:
1. Enhanced Decision-Making
AI processes large volumes of data quickly, uncovering patterns that help businesses make faster and more accurate decisions, complementing human judgment.
2. Improved Engagement
AI enhances customer experiences and boosts employee engagement by personalizing interactions and optimizing workflows, leading to a more competitive business environment.
3. Streamlined Automation
AI automates routine tasks, allowing employees to focus on more critical activities, which increases productivity and delivers rapid returns on investment.
4. Innovation in Products and Services
AI drives deeper innovation by enabling the creation of new products and services that are tailored to meet evolving customer needs.
5. Competitive Edge
When integrated with a well-planned digital strategy, AI offers a significant competitive advantage by improving decision-making, engagement, automation, and innovation across the business.
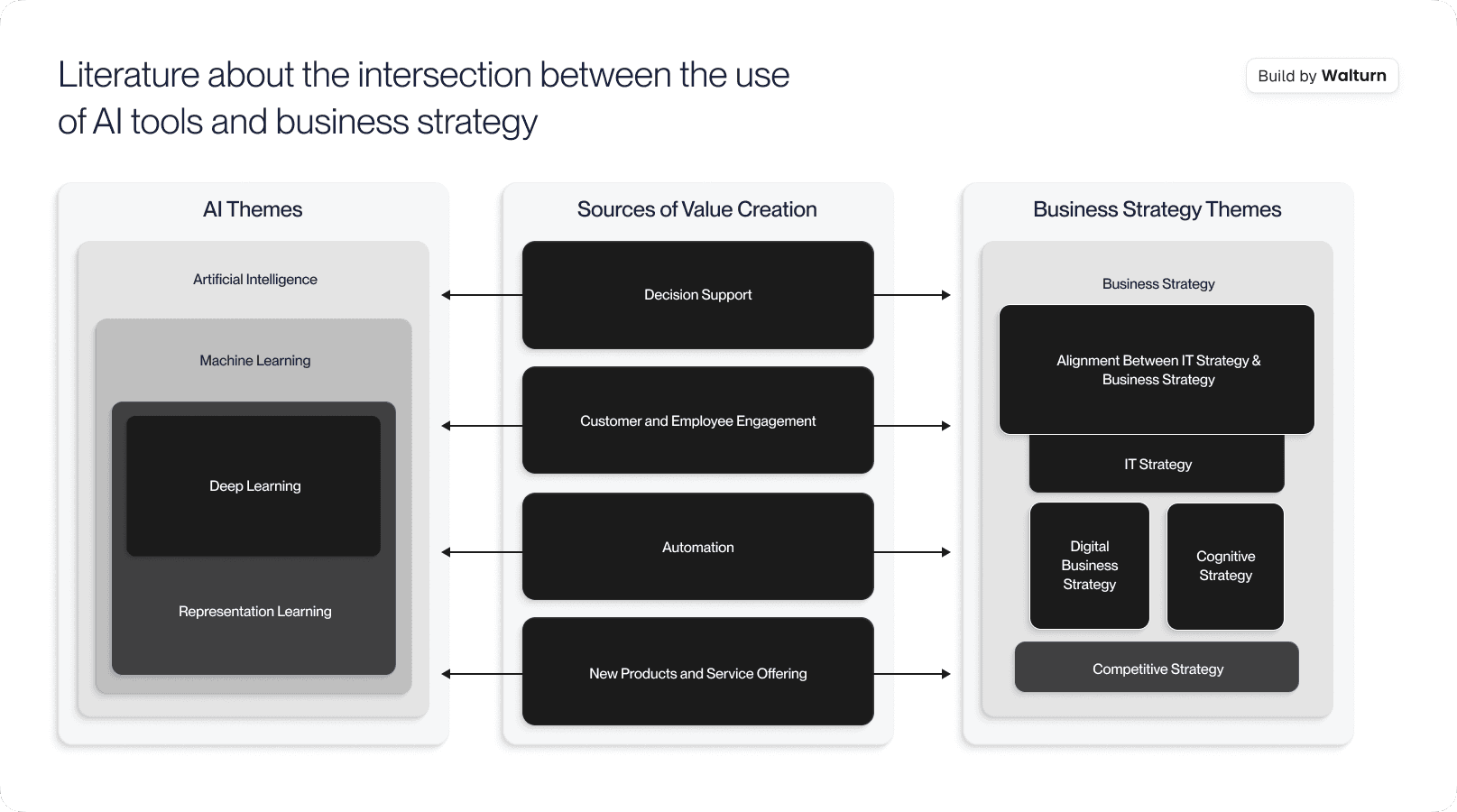
There are many innovative ways to incorporate AI into backend development. These include:
1. Automated Bug Detection and Resolution
AI analyzes code to identify and fix potential bugs or vulnerabilities, saving time and enhancing application security.
2. Efficient Database Management
AI-powered tools optimize database operations by analyzing usage patterns and automatically adjusting configurations, improving performance and scalability.
3. Intelligent Error Handling
AI learns from past errors and adapts to handle similar situations in the future, reducing downtime and improving user experience.
4. Advanced Security Measures
AI enhances security by detecting potential threats, identifying suspicious activity patterns, and enabling proactive defense mechanisms.
5. Code Completion Tools
AI tools like GitHub’s Copilot generate code snippets based on natural language queries, streamlining the coding process.
6. Bug Prediction and Detection Tools
AI tools like DeepCode predict and identify potential issues in code, helping developers address them proactively.
7. Automated Testing Tools
AI tools like Applitools conduct visual testing of software applications, ensuring consistency and reliability.
AI in back-end development is not about replacing human developers but augmenting their capabilities. AI serves as a powerful ally, automating repetitive tasks, optimizing processes, and enhancing security. However, the human touch remains essential in overseeing, refining, and guiding AI-generated outputs to ensure the best possible outcomes. Together, AI and human developers create a synergistic partnership that accelerates development while maintaining quality, scalability, and innovation.
Here are some best practices Walturn is partaking in to make sure the integration of AI into backend development is seamless:
1. Assess AI Readiness
Evaluate your organization’s AI readiness, including funding, technical expertise, and infrastructure. Identify and address any gaps.
2. Identify AI Use Cases
Determine specific scenarios where AI can enhance backend operations, such as predictive analytics, natural language processing, or anomaly detection.
3. Select Appropriate AI Technologies
Research and choose AI technologies that align with your business goals, ensuring they integrate well with your backend systems and are scalable.
4. Invest in Data Management
Implement strong data management practices to support AI initiatives, ensuring ethical data collection, storage, processing, and quality control.
5. Foster Collaboration
Promote collaboration between business stakeholders and technology teams to align AI projects with business objectives, encouraging open communication and cross-departmental cooperation.
6. Testing and Quality Assurance
Validate the accuracy and performance of AI modules using specialized testing methods. Ensure that AI-driven features meet all requirements and are reliable for software development.
7. Deployment and Maintenance
Facilitate the smooth deployment and ongoing maintenance of AI-enabled features, ensuring they perform optimally. Establish protocols for seamless integration of AI tools into production environments and continuous improvement of their efficiency.
Conclusion
In conclusion, creating and maintaining high-quality backend systems requires best practices across the software development lifecycle. At Walturn, we implement these best practices and use widely recognized tools to build systems that guarantee a seamless and productive life cycle for our team.
Authors
Boost Your Backend with Best Practices
Discover how Walturn can elevate your backend systems with cutting-edge tools and industry-leading practices. Learn the secrets to optimizing performance, enhancing security, and scaling your applications efficiently. Dive deep into our methodologies and start transforming your backend infrastructure today.
References
“16 DevOps Best Practices Every Developer Should Know.” Spacelift, 20 May 2024, spacelift.io/blog/devops-best-practices.
Abba, Ihechikara. “What Is an ORM – the Meaning of Object Relational Mapping Database Tools.” freeCodeCamp.org, 21 Oct. 2022, www.freecodecamp.org/news/what-is-an-orm-the-meaning-of-object-relational-mapping-database-tools.
Abid, Marwen. “Four REST API Versioning Strategies.” xMatters, 5 June 2024, www.xmatters.com/blog/blog-four-rest-api-versioning-strategies.
“About READMEs - GitHub Docs.” GitHub Docs, docs.github.com/en/repositories/managing-your-repositorys-settings-and-features/customizing-your-repository/about-readmes.
Aby, Aravind. “What Are Git Tags: Types, Commands, and Best Practices.” Hatica, 5 Apr. 2023, www.hatica.io/blog/git-tags.
Ahmed, Abdullah. Best Practices at Walturn: Driving Excellence and Innovation - Walturn Insight. www.walturn.com/insights/best-practices-at-walturn-driving-excellence-and-innovation.
Ahmed, Abdullah, et al. Best Practices for Flutter Development - Walturn Insight. www.walturn.com/insights/best-practices-for-flutter-development.
AnalyticsMentorIo, Brandon Southern-. “SQL Best Practices - Brandon Southern - AnalyticsMentor.io - Medium.” Medium, 17 Feb. 2023, medium.com/@BrandonSouthern/sql-best-practices-e1c61e96ee27.
“Application Performance Monitoring Explained | Sentry.” Sentry, sentry.io/resources/application-performance-monitoring-explained.
Architect, Pradeep Tiwari Solution. “Five Github Actions Do’s and Don’ts for DevOps Developers.” Medium, 17 Sept. 2023, medium.com/@pradeeptiwari.bhumca10/five-github-actions-dos-and-don-ts-for-devops-developers-a869f088b551.
Atlassian. “DevOps Best Practices | Atlassian.” Atlassian, www.atlassian.com/devops/what-is-devops/devops-best-practices#.
Bcgov. “BC-Policy-Framework-For-GitHub/BC-Gov-Org-HowTo/Naming-Repos.md at Master · Bcgov/BC-Policy-Framework-For-GitHub.” GitHub, github.com/bcgov/BC-Policy-Framework-For-GitHub/blob/master/BC-Gov-Org-HowTo/Naming-Repos.md.
Beighton, Brad. “NestJS Pros and Cons From a Solution Architect | Medium.” Medium, 9 Aug. 2023, bradbeighton.medium.com/nestjs-the-pros-and-cons-aff714607b07.
Bello, Gbadebo. “Best Practices for API Error Handling.” Postman Blog, 10 July 2024, blog.postman.com/best-practices-for-api-error-handling.
“Benefits and Limitations of Automated Software Testing: Systematic Literature Review and Practitioner Survey.” IEEE Conference Publication | IEEE Xplore, 1 June 2012, ieeexplore.ieee.org/document/6228988.
Bennett, Terence. “Applying AI in Software Development: Best Practices and Examples.” DreamFactory, 7 May 2024, blog.dreamfactory.com/applying-ai-in-software-development-best-practices-and-examples.
Bento, Andre, et al. “Automated Analysis of Distributed Tracing: Challenges and Research Directions.” Journal of Grid Computing, vol. 19, no. 1, Feb. 2021, https://doi.org/10.1007/s10723-021-09551-5.
Bierman, Gavin, et al. “Understanding TypeScript.” Lecture notes in computer science, 2014, pp. 257–81. https://doi.org/10.1007/978-3-662-44202-9_11.
Borges, Aline F. S., et al. “The Strategic Use of Artificial Intelligence in the Digital Era: Systematic Literature Review and Future Research Directions.” International Journal of Information Management, vol. 57, Apr. 2021, p. 102225. https://doi.org/10.1016/j.ijinfomgt.2020.102225.
Burdiuzha, Roman. “Building an Effective CI/CD Pipeline: A Comprehensive Guide.” Medium, 1 May 2024, gartsolutions.medium.com/building-an-effective-ci-cd-pipeline-a-comprehensive-guide-bb07343973b7.
Choi, Kenneth. “Stateful and Stateless Authentication - Kenneth Choi - Medium.” Medium, 26 Oct. 2022, medium.com/@kennch/stateful-and-stateless-authentication-10aa3e3d4986.
Cloud Application Hosting for Developers | Render. www.render.com.
Cloud Application Platform | Heroku. www.heroku.com.
“Cloud Computing Services | Google Cloud.” Google Cloud, cloud.google.com/?hl=en.
“Cloud Computing Services - Amazon Web Services (AWS).” Amazon Web Services, Inc., www.aws.amazon.com.
“Code Documentation.” IEEE Journals & Magazine | IEEE Xplore, 1 Aug. 2010, ieeexplore.ieee.org/document/5484109.
“Compliance Programs - Amazon Web Services (AWS).” Amazon Web Services, Inc., aws.amazon.com/compliance/programs.
Crystal‐Ornelas, Robert, et al. “A Guide to Using GitHub for Developing and Versioning Data Standards and Reporting Formats.” Earth and Space Science, vol. 8, no. 8, Aug. 2021, https://doi.org/10.1029/2021ea001797.
“Documentation | NestJS - a Progressive Node.js Framework.” Documentation | NestJS - a Progressive Node.js Framework, docs.nestjs.com/exception-filters.
Documentation - Do’s and Don’ts. www.typescriptlang.org/docs/handbook/declaration-files/do-s-and-don-ts.html.
“Documentation Best Practices.” Styleguide, google.github.io/styleguide/docguide/best_practices.html.
Firebase. “Firebase.” Firebase, https://firebase.google.com.
The Future of Artificial Intelligence in Back-End Development | MoldStud. 27 Jan. 2024, moldstud.com/articles/p-the-future-of-artificial-intelligence-in-back-end-development.
Garousi, Vahid, and Mika V. Mäntylä. “When and What to Automate in Software Testing? A Multi-vocal Literature Review.” Information and Software Technology, vol. 76, Aug. 2016, pp. 92–117. https://doi.org/10.1016/j.infsof.2016.04.015.
GoldenbergLab. “GitHub - GoldenbergLab/Naming-and-documentation-conventions: Naming Conventions Are Helpful Practices That Teams Follow to Write Code Together.” GitHub, github.com/GoldenbergLab/naming-and-documentation-conventions.
“How Can I Avoid Charges on My Account When Using AWS Free Tier Services?” Amazon Web Services, Inc., aws.amazon.com/free.
How to Protect Against SQL Injection Attacks | Information Security Office. security.berkeley.edu/education-awareness/how-protect-against-sql-injection-attacks.
Insighture. “Release Strategies Using Git Branching - Insighture - Medium.” Medium, 17 Jan. 2024, medium.com/@Insighture/release-strategies-using-git-branching-025b2edcc67a.
“Is Prisma ORM an ORM? | What Is an ORM?” Prisma Documentation, www.prisma.io/docs/orm/overview/prisma-in-your-stack/is-prisma-an-orm.
Jackson, Mackenzie. “API Keys Security and Secrets Management Best Practices - GitGuardian Blog.” GitGuardian Blog - Code Security for the DevOps Generation, 29 Apr. 2024, blog.gitguardian.com/secrets-api-management.
Johnston, Victoria. “Backend Error Handling: Practical Tips From a Startup CTO.” DEV Community, 17 Aug. 2023, dev.to/ctrlaltvictoria/backend-error-handling-practical-tips-from-a-startup-cto-h6.
“Kebab Case - MDN Web Docs Glossary: Definitions of Web-related Terms | MDN.” MDN Web Docs, 1 Aug. 2024, developer.mozilla.org/en-US/docs/Glossary/Kebab_case.
Lwakatare, Lucy Ellen, et al. “DevOps in Practice: A Multiple Case Study of Five Companies.” Information and Software Technology, vol. 114, Oct. 2019, pp. 217–30. https://doi.org/10.1016/j.infsof.2019.06.010.
“Managing Releases in a Repository - GitHub Docs.” GitHub Docs, docs.github.com/en/repositories/releasing-projects-on-github/managing-releases-in-a-repository.
“Managing Tags in GitHub Desktop - GitHub Docs.” GitHub Docs, docs.github.com/en/desktop/managing-commits/managing-tags-in-github-desktop.
Marcel.L. “GitHub Repository Best Practices.” DEV Community, 17 Aug. 2024, dev.to/pwd9000/github-repository-best-practices-23ck.
MongoDB. “MongoDB: The Developer Data Platform.” MongoDB, www.mongodb.com.
“NestJS - a Progressive Node.js Framework.” NestJS - a Progressive Node.js Framework, www.nestjs.com.
“Npm: Pg.” Npm, www.npmjs.com/package/pg.
Paul, Joshua Idunnu. “Database Transactions Explained: A Deep Dive Into Reliability.” Medium, 19 Feb. 2024, cybernerdie.medium.com/database-transactions-explained-a-deep-dive-into-reliability-17ab4e17117a.
Perez-Riverol, Yasset, et al. “Ten Simple Rules for Taking Advantage of Git and GitHub.” PLoS Computational Biology, vol. 12, no. 7, July 2016, p. e1004947. https://doi.org/10.1371/journal.pcbi.1004947.
Phyo, Myat Su. “Best Practices for Error Handling in Backend Development.” Medium, 5 Feb. 2024, medium.com/@myat.su.phyo/best-practices-for-error-handling-in-backend-development-0f9faea39a66.
“PostgreSQL.” PostgreSQL, 21 Aug. 2024, www.postgresql.org.
“PostgreSQL on Render – Render Docs.” PostgreSQL on Render – Render Docs, docs.render.com/databases.
“Railway.” Railway, www.railway.app.
“Redis Software - Redis.” Redis, 26 July 2024, redis.io/enterprise.
“Render Vs Heroku – Render Docs.” Render Vs Heroku – Render Docs, docs.render.com/render-vs-heroku-comparison.
Secret Double Octopus. “What Is Stateless Authentication ? - Security Wiki.” Secret Double Octopus, 2 July 2024, doubleoctopus.com/security-wiki/network-architecture/stateless-authentication.
Sentry. Automate, Group, and Get Alerted: A Best Practices Guide to Monitoring Your Code. www.sentry.dev/static/8d9621f6308bbf7d211ef13eb9a48847/sentry-automate-group-alert-ebook.pdf.
Silveira, Scheila Farias. “5 Tips on How to Use AI in Software Development - Ubiminds.” Ubiminds. You, International., 26 June 2024, ubiminds.com/en-us/leveraging-ai-for-software-development.
SQL Injection Prevention - OWASP Cheat Sheet Series. cheatsheetseries.owasp.org/cheatsheets/SQL_Injection_Prevention_Cheat_Sheet.html.
“Supabase | the Open Source Firebase Alternative.” Supabase, www.supabase.com.
“Systematic Literature Review on Security Risks and Its Practices in Secure Software Development.” IEEE Journals & Magazine | IEEE Xplore, 2022, ieeexplore.ieee.org/document/9669954.
“Technology.” Stack Overflow 2024 Developer Survey, survey.stackoverflow.co/2024/technology#most-popular-technologies-language-prof.
Triare Ukraine. “Unlocking the Power of AI in Frontend and Backend Development.” TRIARE, 2 Apr. 2024, triare.net/insights/ai-web-development.
Ugochukwu, Barry. “Mastering API Versioning: Best Practices &Amp; Strategies for 2023.” DevOps Blog, 17 Oct. 2023, kodekloud.com/blog/api-versioning-best-practices.
View of Beyond the Buzz: A Journey Through CI/CD Principles and Best Practices. ejtas.com/index.php/journal/article/view/286/236.
Welcome to Uptime by Better Stack | Better Stack Documentation. 28 May 2023, betterstack.com/docs.
“What Is API Versioning? Benefits, Types and Best Practices | Postmann.” Postman API Platform, postman.com/api-platform/api-versioning.
“What Is DevOps? - DevOps Models Explained - Amazon Web Services (AWS).” Amazon Web Services, Inc., aws.amazon.com/devops/what-is-devops.
“What Is SQL? - Structured Query Language (SQL) Explained - AWS.” Amazon Web Services, Inc., aws.amazon.com/what-is/sql.
Winkler, Dietmar, et al. “Software Quality. Complexity and Challenges of Software Engineering in Emerging Technologies.” Lecture notes in business information processing, 2017, https://doi.org/10.1007/978-3-319-49421-0.