Understanding Mixins in Dart
Engineering
Object-Oriented Programming
Dart
Summary
This article explores mixins in Dart, detailing how they extend class functionality without inheritance. Mixins allow behavior injection into classes, promoting code reuse and flexibility. Through examples, the article covers their structure, constraints, and advanced features like mixin composition, abstract methods, and super calls, emphasizing mixins’ role in creating modular, scalable, and maintainable systems.
Key insights:
Mixins Overview: Mixins allow developers to inject functionality into classes without altering inheritance hierarchies, promoting reusable and modular code.
Inheritance vs. Mixins: Unlike inheritance or interfaces, mixins allow behavior sharing across classes without enforcing strict class relationships.
Common Use Cases: Mixins excel in sharing behavior among unrelated classes, separating concerns, enhancing code modularity, and decoupling functionality.
Mixin Composition and Constraints: Dart allows mixins to be composed, applying behaviors to classes in a specific order while using constraints to limit mixin application to certain classes.
Abstract Methods in Mixins: Mixins can declare abstract methods, requiring classes using the mixin to implement specific functionality, ensuring a robust structure.
Super Calls in Mixins: Mixins can use the
super
keyword to extend or modify inherited behavior, providing additional flexibility in enhancing class methods.
Introduction
In modern software development, creating reusable and modular code is key practice for building maintainable and scalable systems. The concept of mixins enables this practice in object-oriented programming by allowing developers to extend a class’ functionality without the complexities associated with inheritance.
This article aims to explore mixins, providing details about their concept, structure, and implementation with practical examples in Dart.
What are Mixins?
Mixins originated as a solution to some of the limitations found in traditional inheritance mechanisms. These limitations included the inflexibility of single inheritance, tight coupling between classes (meaning that the classes are highly dependent on each other, making it difficult to change one class without affecting the other), and potential conflicts in multiple inheritance scenarios like the diamond problem (read more here).
The mixin concept was introduced to solve these problems by allowing a class to gain additional functionality without directly inheriting from multiple parent classes. Languages like Dart adopted mixins as a way to add specific behaviors to classes in a modular and reusable manner. Unlike full multiple inheritance, mixins provide a more controlled approach where attributes and methods can be added to a class without altering the fundamental class hierarchy.
At its core, a mixin is a design pattern that allows developers to inject functionality into a class without using traditional inheritance, enabling code reuse by providing a way to “mix-in” additional behavior to classes. From a theoretical perspective, mixins are an alternative to both single and multiple inheritance, offering a more flexible way of sharing methods across unrelated classes.
Inheritance, Interfaces, and Mixins
In this section, we explore the distinction between inheritance, interfaces, and mixins - concepts that often cause confusion. We will clarify their unique characteristics and provide examples of mixin usage.
1. Inheritance
Inheritance is the mechanism by which a class (child or subclass) can inherit properties and methods from another class (parent or superclass). The child class can use the functionality provided by the parent class, and it can also override or extend the parent class’ methods to provide specialized behavior.
Here is an example of inheritance in Dart:
In this example, the Dog class inherits the eat method from the Animal class. This establishes an "is-a" relationship, meaning that a dog is a type of animal. However, inheritance can become limiting when multiple classes need to share behavior that does not naturally fit into such a hierarchy.
2. Interfaces
Interfaces allow classes to define contracts that must be followed without providing implementation. A class that implements an interface must provide definitions for the methods and properties declared by the interface.
In Dart, interfaces are typically implemented using abstract classes that lay out function signatures without concrete implementations. Here is an example of creating an interface in Dart along with a class that implements the interface:
Here, Flyable is an abstract class acting as an interface. Airplane implements Flyable. Interfaces are useful when you need to define a contract that multiple classes must follow but without any shared code.
3. Mixins
Mixins allow behavior to be reused across multiple classes without forcing a parent-child relationship or requiring strict method contracts of interfaces. This makes mixins particularly useful when multiple classes need to share common behavior without altering their inheritance structure.
You can mix in functionality using the with keyword in Dart. However, it is important to note that mixins cannot have constructors or inherit from other classes. Here is an example:
In the code above, the Swimmable mixin provides swimming behavior to both the Fish and Human classes. The Fish class also inherits from Animal, gaining both swimming and eating behavior. Mixins allow for flexible code reuse across unrelated classes, enabling behavior injection without creating a deep inheritance hierarchy.
Here is a table summarizing the key differences between inheritance, interfaces, and mixins:
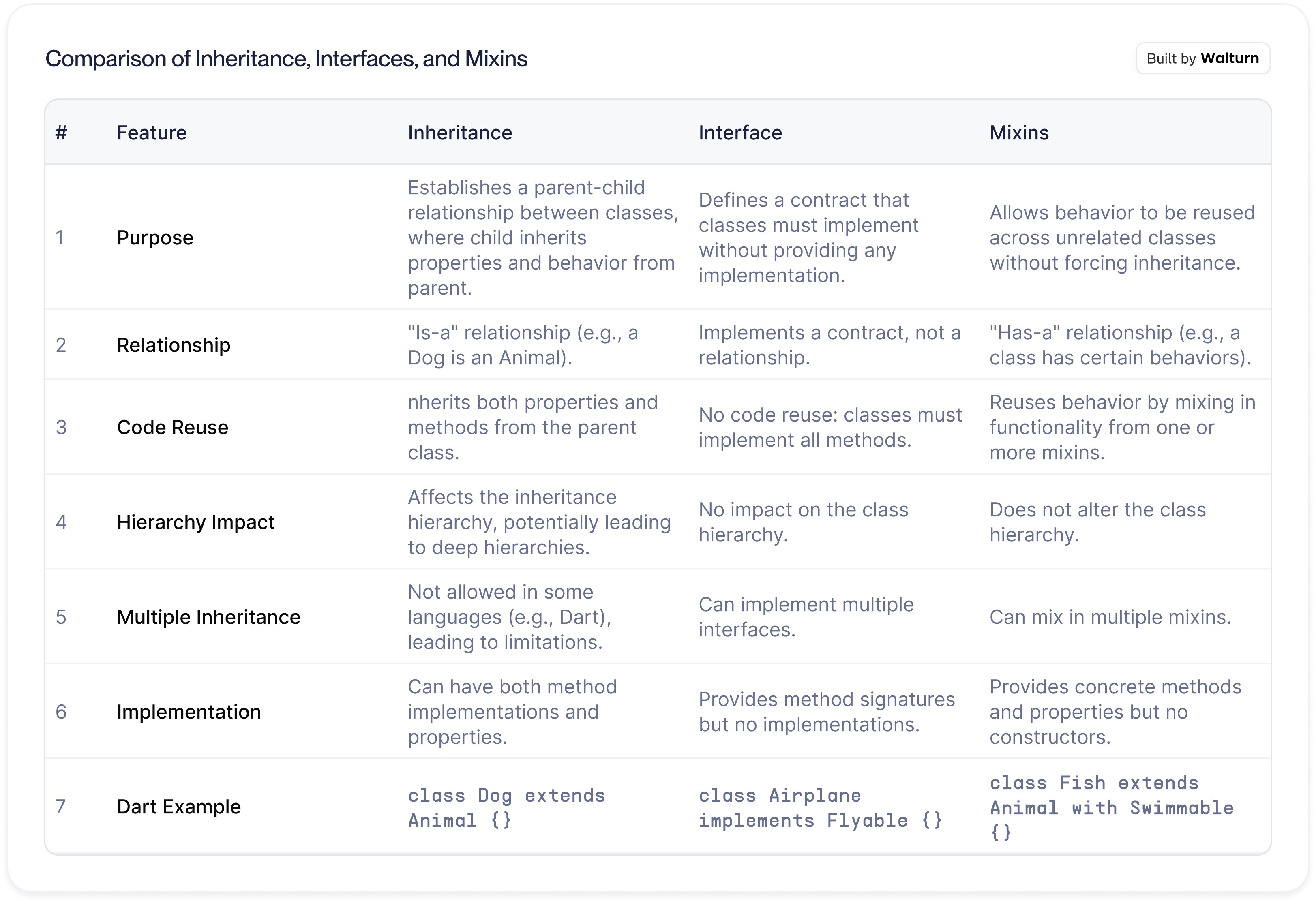
In summary, inheritance should be used when there is a clear parent-child relationship between classes. The child class inherits methods and properties from the parent class. Interfaces should be used when you need to force a class to follow a specific contract but do not want to share implementation. All methods in the interface must be implemented in the child class. Mixins should be used when you want to inject behavior into multiple classes without forcing inheritance.
4. Common Use Cases for Mixins
Mixins are best suited for situations where classes need to share behavior that does not naturally fit into the hierarchy of inheritance. Some of the most common use cases for mixins include:
Code Reuse: One of the most common uses for mixins is to share behavior across unrelated classes. With inheritance, you are limited to parent classes, but with mixins, you can apply the same functionality to various classes without creating a hierarchical relationship.
Let's take a look at an example:
In the example above, the Logger mixin is used to add logging functionality to both the Car and Boat classes. The mixin allows us to inject the log method into these otherwise unrelated classes without requiring them to share a common parent and repeating code.
Enhancing Code Modularity: Mixins help you modularize code by allowing you to separate specific behaviors into reusable components. This improves maintainability by reducing code duplication and keeping functionality focused, making it easier to extend or modify your codebase without affecting unrelated parts.
Separation of Concerns: Mixins can be used to achieve separation of concerns by extracting specific behaviors from classes and encapsulating them into self-contained, reusable components. This allows your classes to stay focused on their primary responsibilities while acquiring additional functionality from mixins. Here is a simple example in Dart that aims to separate UI and business logic using mixins:
In the example above, the business logic (incrementing and decrementing the counter) is separated from the user interface logic (displaying the counter value) using Dart’s mixins. This approach ensures that the logic for managing the counter can be reused independently of how the counter is displayed, making it easier to update, test, and extend each concern individually.
Decoupling Behavior: When designing large systems, mixins can decouple certain behaviors from the core business logic, making them independent and easier to manage. Decoupling behavior ensures that each part of the system can be modified, tested, or reused without impacting other parts of the system.
Advanced Topics in Mixins (Dart)
In this section, we will talk about advanced topics in Mixins in the context of Dart.
1. Mixin Composition
One of the most powerful features of mixins is their ability to be composed. In Dart, a class can mix in multiple mixins, allowing developers to inject a variety of behaviors into a class in a modular and reusable way. This form of composition ensures that functionality can be spread across different classes without creating tightly coupled dependencies between them.
When applying multiple mixins to a class, Dart uses linear ordering. This means that if multiple mixins provide methods with the same name, the method from the last mixin in the chain will be given precedence, overriding any earlier mixin methods. This behavior is useful, but it requires careful planning to avoid any conflicts between mixed-in methods. Here is an example:
In this example, both the Duck and Penguin classes mix in with Flyable and Swimmable. However, the outputs differ based on the order in which the mixins are defined for each class.
This behavior introduces the need for clear documentation and awareness of the order in which mixins are applied to avoid unintended conflicts.
2. Mixin Constraints in Dart
While mixins are highly flexible, there are cases where developers may want to apply certain constraints to ensure that a mixin can only be applied to a specific type of class. Dart allows developers to define mixin constraints by specifying that a mixin can only be applied to a class that extends or implements another class. This is done using the on keyword, which restricts the application of the mixin to a particular class or subclass. Here is an example:
In the above example, the Flyer mixin has a constraint that it can only be applied to classes that extend the Animal class. When we try to mix in Flyer in the Car class, Dart throws an error.
This constraint mechanism allows developers to ensure that mixins are only applied in appropriate contexts, making the code more predictable and easier to debug.
3. Mixin Class
A mixin class in Dart is a class that can be used both as a mixin and as a regular class, allowing it to be instantiated directly. This feature is important because it provides greater flexibility in code reuse and organization, enabling developers to create components that can be mixed into other classes or used standalone. The main restriction of mixin classes is that they cannot extend other classes, as they are implicitly assumed to extend Object, but they can implement interfaces. Additionally, we still cannot create constructors in mixin class. Here is an example:
In the code above, we can see how Logger (a mixin class) can be used to mix in with another class and act as a standalone class.
4. Super Calls in Mixins
Mixins in Dart can use the super keyword to call methods in the superclass or in mixins that come earlier in the mixin chain. This feature allows mixins to extend or modify the behavior of existing methods rather than completely overriding them. Here's an example:
In this example, the Barker mixin calls the superclass' (Animal’s) makeSound method before adding its own behavior. When executed, it first prints out “The animal makes a sound” and then “Bark!”.
This capability allows mixins to augment existing behavior rather than completely replace it, providing more flexibility in how mixins can modify class behavior.
5. Abstract Methods in Mixins
Mixins in Dart can declare abstract methods, which must be implemented by the class that uses the mixin. This feature allows mixins to define a contract that classes must fulfill, ensuring that certain functionality is available when the mixin is used. Here's an example:
In this example, the Swimmer mixin declares an abstract swim method and provides a concrete performSwim method that uses it. Any class that uses this mixin must provide an implementation for the swim method. This allows the mixin to define both behavior and structure, making it a powerful tool for sharing code across different class hierarchies.
Abstract methods in mixins provide a way to ensure that classes using the mixin implement certain required functionality, making mixins more robust and self-contained.
Conclusion
In conclusion, mixins represent a powerful tool in modern software development, offering a flexible approach to code reuse and modular design. They provide a solution to the limitations of single inheritance and the complexities of multiple inheritance, allowing developers to inject behavior into classes without altering their fundamental structure.
From a computer science perspective, mixins embody the principles of composition over inheritance, promoting more flexible and maintainable code architectures. They enable developers to create modular, reusable components that can be easily combined to build complex systems.
As we have seen through various examples in Dart, mixins can be applied in a wide range of scenarios, from simple behavior sharing to more advanced use cases involving composition, constraints, and abstract methods. The introduction of mixin classes in Dart further extends their utility, allowing for even greater flexibility in code organization.
Authors
Elevate Your Code Quality with Walturn’s Expertise
At Walturn, we specialize in writing high-quality, scalable, and maintainable code tailored to your project’s needs. Our solutions are designed to ensure code reusability and flexibility, allowing your software to grow and adapt seamlessly. Partner with us to build efficient systems that stand the test of time.
References
Bishworaj Poudel. “Inheritance in Dart.” Dart-Tutorial.com, 2020, dart-tutorial.com/object-oriented-programming/inheritance-in-dart.
“Dart Interface.” Dart Tutorial, 4 June 2022, www.darttutorial.org/dart-tutorial/dart-interface.
Ibrahim, Syed. “Mixins in Dart (Flutter) — and How It Is Different from Inheritance ? | Flutter Community.” Medium, Flutter Community, 8 Mar. 2024, medium.com/flutter-community/exploring-mixins-in-dart-flutter-43514cd9952b.
“Mixins.” Dart.dev, dart.dev/language/mixins.
Romain Rastel. “Dart: What Are Mixins? - Flutter Community - Medium.” Medium, Flutter Community, 16 Sept. 2018, medium.com/flutter-community/dart-what-are-mixins-3a72344011f3.
Santhosh Adiga U. “Abstract Classes and Interfaces – Flutter - Santhosh Adiga U - Medium.” Medium, Medium, 17 Mar. 2023, santhosh-adiga-u.medium.com/abstract-classes-and-interfaces-flutter-456d95cc78ac.
Vimal DGM. “What Is the Diamond Inheritance Problem? Unravelling the Complexities of a Key OOP Issue.” Medium, Medium, 18 Aug. 2023, medium.com/@etherservices.vimalraj/what-is-the-diamond-inheritance-problem-unravelling-the-complexities-of-a-key-oop-issue-7d3c8eefdf0b.
Yaqoob, Eman. “Mixins in Dart - Eman Yaqoob - Medium.” Medium, Medium, 13 June 2023, medium.com/@emanyaqoob/mixins-in-dart-aed7e89de15d.