Monitoring Flutter Apps with Sentry and New Relic
Summary
This guide compares Sentry and New Relic for monitoring Flutter apps, covering setup, error tracking, performance monitoring, and additional features. Sentry excels in error tracking and debugging, while New Relic offers broader observability, including infrastructure and application performance monitoring. Both tools help developers maintain app stability and optimize performance.
Key insights:
Sentry's Focus on Error Tracking: Sentry specializes in real-time error detection and performance monitoring, offering tools like exception capturing, breadcrumbs, and stack traces, making it an excellent choice for developers focused on debugging and app stability.
New Relic's Comprehensive Observability: New Relic provides a holistic monitoring solution with features like application performance monitoring (APM), real user monitoring (RUM), and infrastructure tracking, making it ideal for businesses requiring a broader view of their technology stack.
Proactive Issue Resolution: Both tools enable developers to monitor errors in real time, offering insights into user behavior and performance bottlenecks, allowing proactive detection and resolution of issues before they impact users.
Third-Party Integrations: Sentry and New Relic support integrations with popular tools like Slack, enabling real-time alerts and improving team responsiveness to errors and performance issues.
Choosing the Right Tool: Sentry is ideal for developers focused on debugging and error tracking, while New Relic's broader features make it a better fit for enterprises needing comprehensive application and infrastructure monitoring.
Introduction
Effective monitoring is essential for ensuring a smooth user experience and maintaining app stability. Monitoring provides real-time insights into application performance, errors, and user interactions, allowing developers to identify and address issues before they impact users. This article provides a comprehensive guide for setting up monitoring systems like Sentry and New Relic in a Flutter application, enabling developers to proactively manage application health and optimize performance.
Definition and Importance
1. What are Exceptions?
Exceptions are unexpected errors that occur during the execution of a program (during run time). These can range from simple errors such as accessing an empty list or more complex issues like network failures. Exceptions can cause apps to crash or behave unpredictably if they are not handled correctly - leading to poor user experience. Properly monitoring and handling exceptions is important for maintaining app stability, reducing downtime, and ensuring a seamless user experience.
2. Importance
To mitigate these risks, it is important to implement a strong strategy for exception handling and monitoring. Monitoring involves using tools to track and log these errors in real time, providing developers with insights into their frequency, impact, and root causes. Integrating monitoring in your application is considered best practice because of the benefits it brings which include:
Real-Time Error Detection: Monitoring tools like Sentry help detect errors and exceptions as they happen, allowing developers to react quickly and minimize user impact.
Performance Tracking: These tools provide insights into the performance of different parts of the app, helping identify bottlenecks and optimize the code for better efficiency.
User Behavior Insights: Monitoring tools can also capture user interactions and behaviors, offering valuable data that can be used to enhance user experience and guide feature development.
Proactive Issue Resolution: By using monitoring tools, developers can proactively identify potential issues before they escalate, reducing the risk of app crashes and maintaining app stability.
Comprehensive Reporting: These tools generate detailed reports on errors, crashes, and performance issues, providing a comprehensive overview of the app’s health.
User Experience: Repeated instances of the same bug negatively impact user experience which can cause a loss of customers.
Sentry
In this section, we will walk through the process of setting up and integrating Sentry into your Flutter application. Sentry is a powerful monitoring tool that provides real-time error tracking, performance monitoring, and insights into your app's health. By integrating Sentry, you can proactively detect issues, understand the context around errors, and improve the overall stability and performance of your application.
The steps below will guide you through creating a Sentry account, adding the necessary package to your Flutter project, and setting up best practices to ensure that your app is well-monitored and any issues are quickly identified and resolved.
1. Create an Account on Sentry
To get started, head over to the Sentry registration page and create an account if you do not have one already. Once registered, you can choose to follow their welcome guide or skip the onboarding - this article will cover all the essential steps needed to fully integrate Sentry.
2. Create a New Project
Once logged in, head over to the Projects tab and click on Create Project:

Make sure to select Flutter as your platform when prompted:
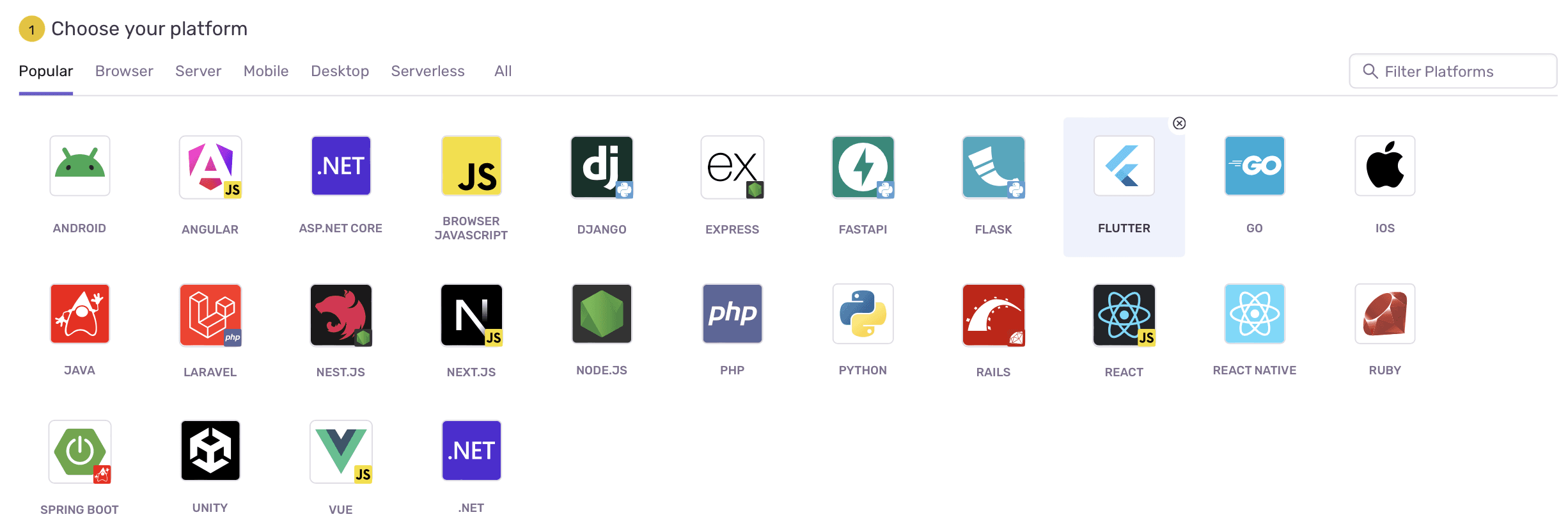
Lastly, give your project a name and finish creating your project.
3. DSN Key
After creating your project, Sentry should provide you with the setup details that contain your DSN key for the project. Keep that in hand as we will use the key later on when initializing Sentry.
If you do not see your key, you can head back over to the Projects tab, click on the project you just created, and navigate to its settings. Your DSN key should be accessible through the Client Keys tab:
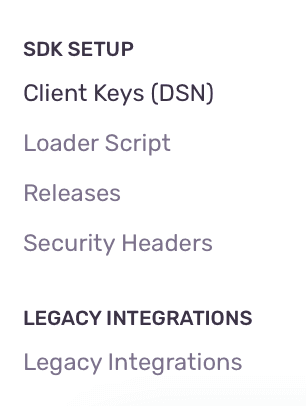
With the DSN key in hand, you are ready to move to your Flutter project and integrate Sentry.
4. Add Sentry Flutter Package
To add Sentry to your Flutter application, you can add the sentry_flutter package in your project by running the following command: flutter pub add sentry_flutter
5. Initialize Sentry in Your Flutter App
To start using Sentry, you need to initialize it at the entry point of your Flutter project. We initialize Sentry in bootstrap.dart to handle global setup and ensure it is initialized before the app runs. The bootstrap.dart file plays a key role in setting up the application environment prior to the app's execution. To learn more about its functionality, click here. Since we are using flavors, we can also customize Sentry's configuration based on the specific flavor.
An example initialization might look like this:
In the code above, we set up the initialization process for a Flutter application that follows best practices for error monitoring and handling using Sentry. The bootstrap function takes two parameters: flavor and sentryUrlDsn. The flavor parameter represents different environments (such as development, staging, or production) to help configure the app accordingly, while the sentryUrlDsn parameter provides the DSN for Sentry, which is necessary for sending error reports and monitoring data to the Sentry server.
We use runZonedGuarded, a Dart function that creates a zone to catch any uncaught asynchronous errors. This is considered a best practice because it ensures that any unexpected errors that occur during the app's execution are caught and handled properly, rather than causing the app to crash.
If you prefer not to use bootstrap.dart, you can simply initialize Sentry in your main function:
The Sentry SDK is initialized using SentryFlutter.init, where we configure it with the provided DSN and other options, such as setting tracesSampleRate and profilesSampleRate to 1.0 to enable full performance monitoring and profiling. To read more about these parameters, check out Sentry’s official documentation here.
In case any uncaught errors occur, the error handler defined in runZonedGuarded captures these errors and reports them to Sentry using Sentry.captureException, ensuring that all errors are logged and can be monitored. This setup provides a secure, efficient, and maintainable way to initialize a Flutter app with robust error handling and monitoring capabilities.
The bootstrap function we define above is called by the entry point of the Flutter project. The entry point could look something like this:
We recommend using the approach above by setting Sentry DSN as a Dart Environment variable to ensure it is not exposed in your source code. To run a Flutter application with the environment variable, you can run the following command:
flutter run --dart-define=SENTRY_DSN=[Your DSN Here]
To run the application in Visual Studio Code, add the following item in the args list in .vscode/launch.json:
--dart-define=SENTRY_DSN=[Your DSN Here]
Make sure to add .vscode/* to your .gitignore file to ensure it is not committed to your repositories.
6. Capture an Exception
With the setup we defined above, Sentry will automatically capture any uncaught exceptions and log them. To test this out, you can throw an intentional exception without a catch statement. Once your exception has been thrown, you can navigate to your project on the Sentry website to review the error. If the exception does not show up, you might have made a mistake in the initialization of Sentry.
However, you may also want to manually capture exceptions or messages at specific points in your application. To achieve this, you can directly call the Sentry.captureException method when needed.
Clicking on the log will provide a detailed view of the captured exception, including the stack trace, error message, and context around the error. This detailed view will help you identify the source of the problem in your code and understand the sequence of events that led to the exception. Sentry also offers additional insights, such as breadcrumbs, which are the recorded events leading up to the error. Breadcrumbs can provide context about the user actions or application state changes that preceded the exception, making it easier to diagnose the issue.
Furthermore, you can use the Sentry dashboard to explore other features such as performance monitoring, which helps identify slow transactions or performance bottlenecks in your application. The dashboard also allows you to set up alerts and notifications, so you can be notified in real time when new exceptions or performance issues are detected. This proactive approach enables you to address potential problems before they affect a large number of users, ensuring a smooth and reliable user experience.
7. Integrating Sentry with Third-Party Applications
Sentry can be integrated with various third-party applications to enhance your monitoring and alerting capabilities. For example, integrating Sentry with Slack allows you to receive notifications directly in your Slack channels whenever an exception occurs. You can access the full guide for integrating Sentry with Slack here.
Integrating Sentry into your Flutter application is a straightforward process that can significantly enhance your ability to handle and monitor exceptions. By following best practices and securely managing your DSN, you can leverage Sentry's powerful monitoring capabilities to improve app stability and user experience.
New Relic
New Relic is a powerful observability platform that provides real-time monitoring and insights into your application's performance, error rates, user interactions, and more. Integrating New Relic with your Flutter application allows you to track errors, monitor app performance, and gain valuable insights into user behavior. This guide will walk you through setting up New Relic for your Flutter app, including account creation, SDK installation, and proper configuration for both iOS and Android platforms.
1. Create an Account on New Relic
To get started with New Relic, visit their website and sign up for a free account if you do not have one already.
2. Create a Project
Once logged in, navigate to the Integrations & Agents tab and search for Flutter.
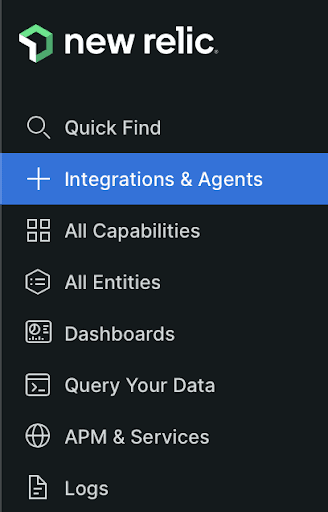
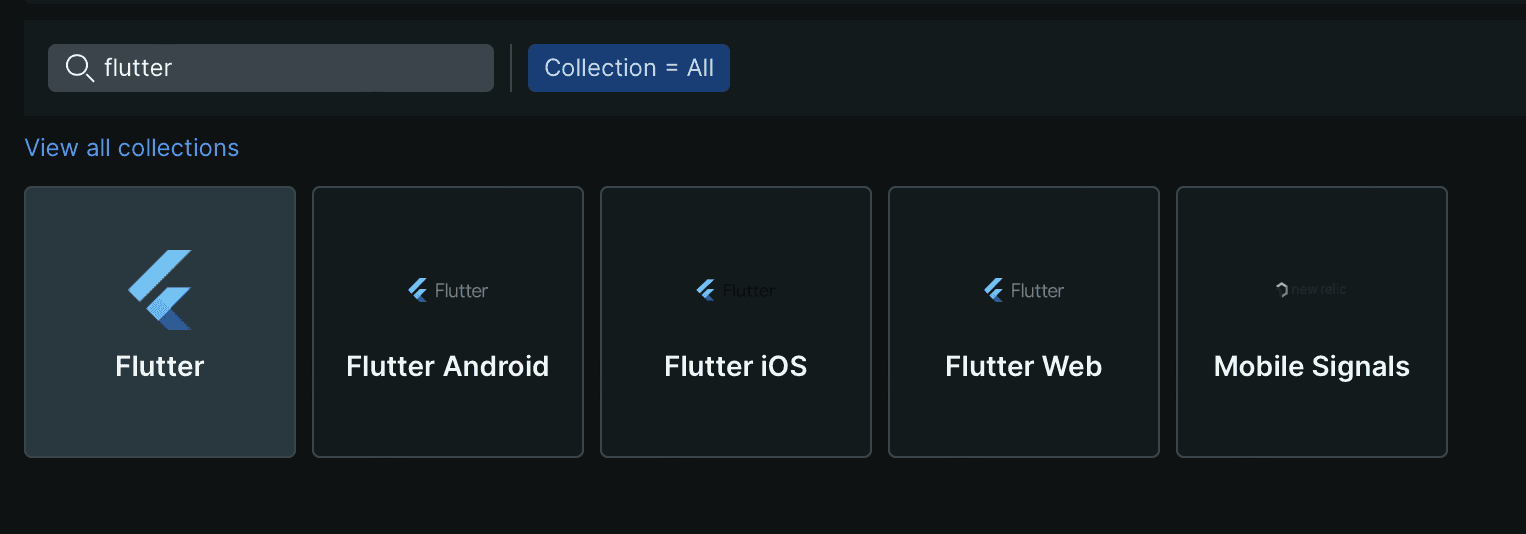
Provide a name for your application that distinguishes it from other apps you might monitor. You will need to create separate entries for both iOS and Android, as they will each have their own specific configuration tokens (app tokens).
3. App Token
Follow the steps on the screen until you reach Flutter Setup. On this page, scroll down to find your App Tokens for both iOS and Android. Once you have your keys, you are ready to integrate New Relic into your Flutter app.
4. Add New Relic Package
Run the following command to add New Relic to your Flutter project: flutter pub add newrelic_mobile
5. Update Android Configuration
To properly integrate New Relic with your Android app, you need to modify the build.gradle files. Add the New Relic repository and plugin to your project-level build.gradle file located at android/build.gradle:
If your build.gradle file is already populated, you can add the code shown above at the top of the file. Note that the classpath may change in future versions. This can be found in the setup guide offered by New Relic upon creating a new project.
Secondly, you need to add the New Relic plugin to your app-level build.gradle file located at android/app/build.gradle:
Lastly, add the following permissions to your AndroidManifest.xml file located in android/profile/.
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
6. Initialize New Relic in Your Flutter Application
Now that the package is added to your project and the necessary changes have been made, you can initialize New Relic in your Flutter application's entry point:
In the code above, the bootstrap function takes three parameters: flavor, iosRelicToken, and androidRelicToken. Similar to the setup described for Sentry, we use runZonedGuarded to catch any uncaught asynchronous errors. We are using the default configuration for New Relic in this code. However, you can make changes as required. To read more, click here.
The bootstrap function we define above is called by the entry point of the Flutter project. The entry point could look something like this:
To run a Flutter application with the environment variables defined above, you can run the following command:
To run the project in Visual Studio Code, please refer to the instructions provided in the Sentry setup earlier.
7. Test Connection
Run your application and return to the New Relic setup page. Here, you will be given the option to test your connection to ensure New Relic is connected to your Flutter application.
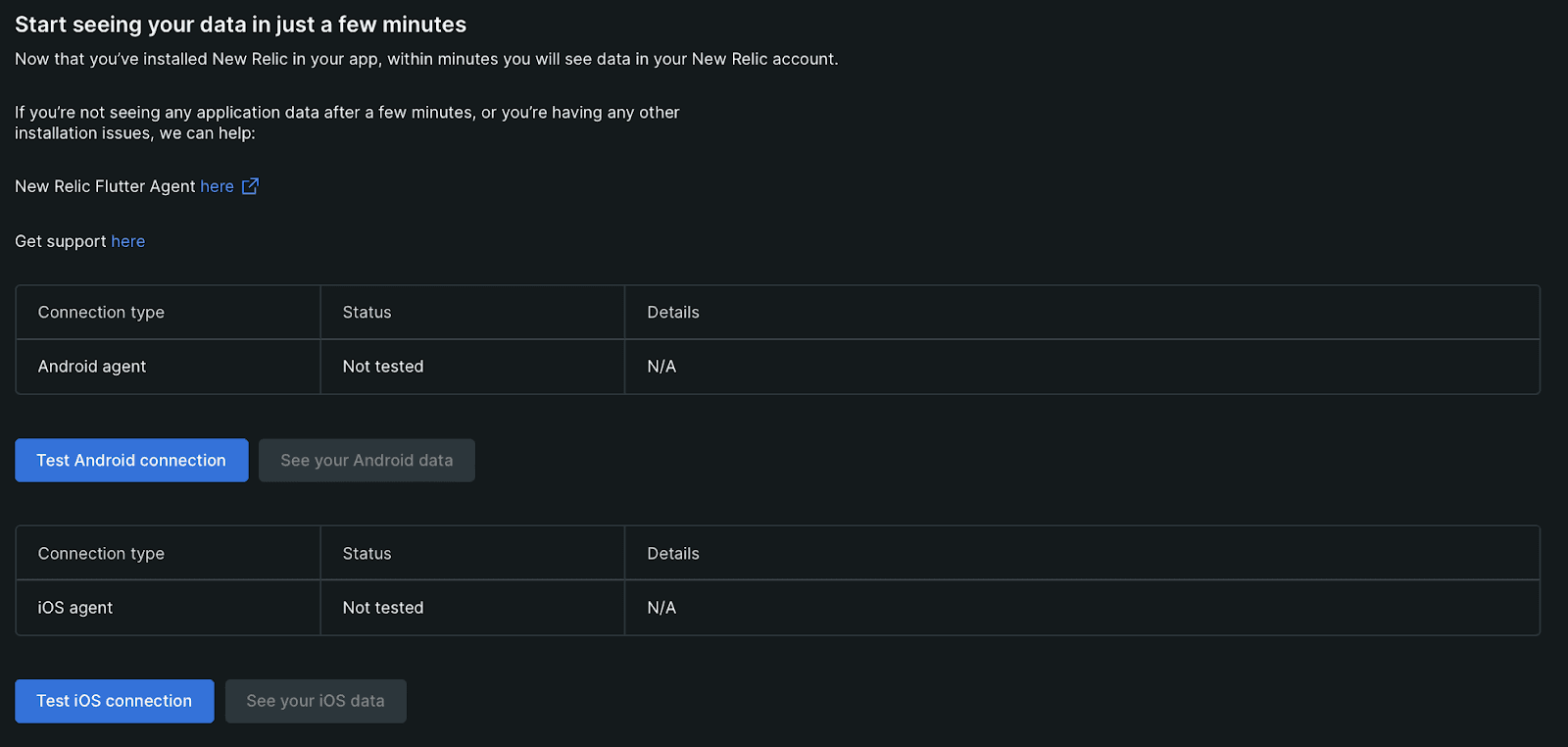
Testing your connection may take a few minutes. A successful connection will appear as below. Once connected, you are ready to monitor your application by clicking on see your data.

Once New Relic is integrated and your app is running, errors should start appearing on your New Relic dashboard, along with other useful information like user metrics, crash rates, HTTP Response Time, App Launches, and more.
8. Additional Features of New Relic
While this guide focuses on setting up monitoring for errors and crashes, New Relic offers much more. You can explore their website to learn about advanced features like synthetic monitoring, infrastructure monitoring, application performance monitoring (APM), and real user monitoring (RUM). These features provide comprehensive insights into every aspect of your application's performance and user experience.
New Relic also supports integration with Slack to receive real-time notifications. The official guide can be found here.
By integrating New Relic with your Flutter application, you gain a powerful tool for monitoring and improving app performance, reducing crashes, and providing a better user experience. For more information on what New Relic has to offer, visit their website.
Comparing Sentry and New Relic
Sentry and New Relic are both powerful monitoring tools, but they serve slightly different purposes and excel in different areas.
1. Sentry
Sentry is primarily focused on error tracking and performance monitoring, making it an excellent choice for developers who want to capture and analyze application exceptions, crashes, and performance bottlenecks. It provides real-time insights into the health of an application by capturing errors and stack traces, along with context such as breadcrumbs that help trace user actions leading to an error. Sentry is particularly valuable for debugging and maintaining application stability, as it allows developers to see precisely where and why an issue occurred.
2. New Relic
New Relic offers a broader range of observability features beyond error tracking, including infrastructure monitoring, application performance monitoring (APM), real user monitoring (RUM), and synthetic monitoring. This makes New Relic a more comprehensive solution for businesses looking to gain a holistic view of their entire technology stack, from backend servers to client-side applications.
Here is a table that compares the availability of key features in both platforms that developers would generally require for monitoring their apps:
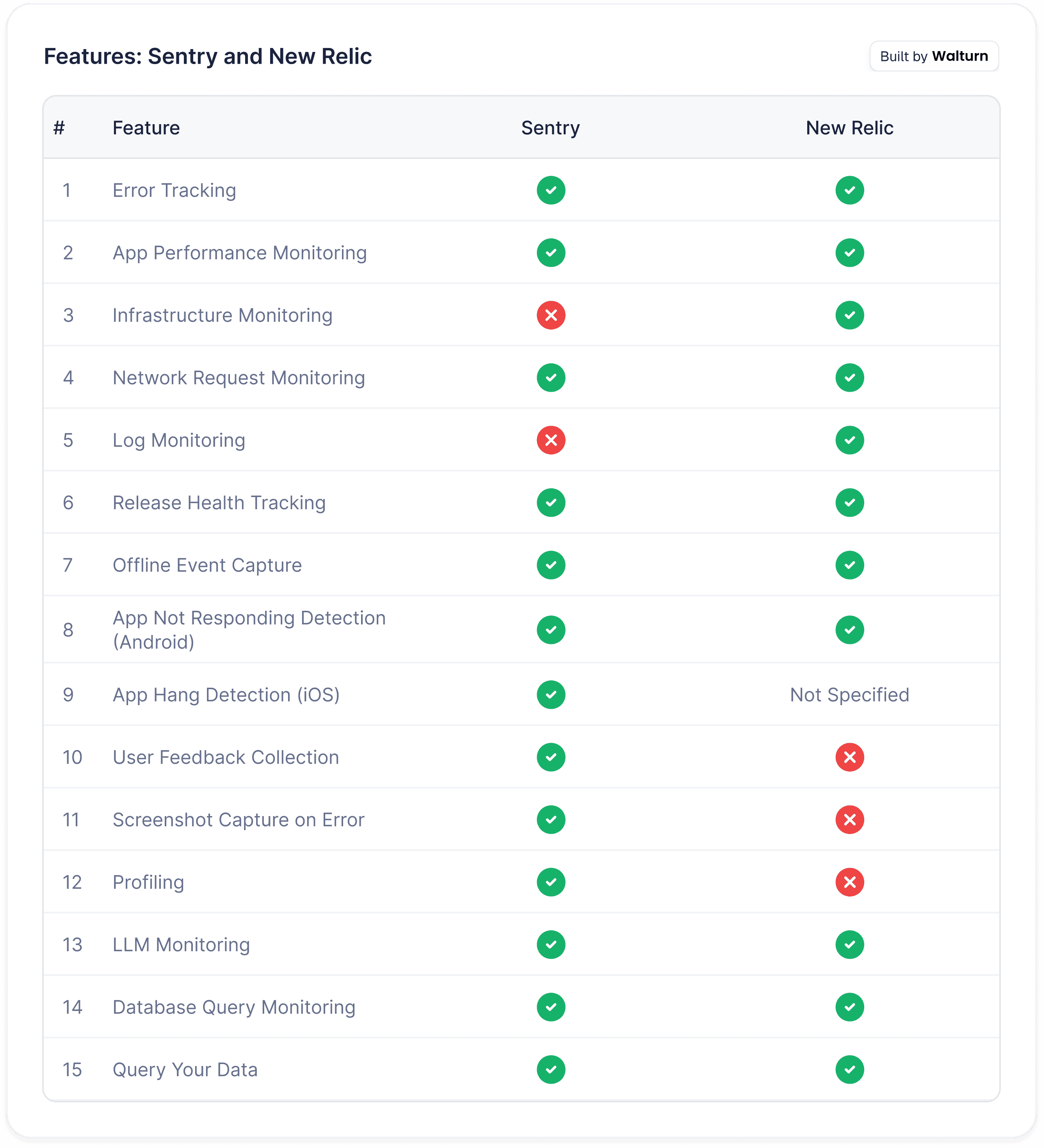
While Sentry focuses more on developers' needs for debugging and exception management, New Relic provides a unified platform for monitoring the performance and health of applications across different environments. Both tools can be integrated with third-party services like Slack to provide alerts and notifications, enhancing team responsiveness to issues. Choosing between Sentry and New Relic depends largely on the specific needs of the project: Sentry is ideal for focused error tracking and debugging, while New Relic offers a more expansive suite of monitoring capabilities suitable for enterprise-level observability.
Conclusion
In conclusion, both Sentry and New Relic are valuable tools for monitoring and improving the reliability of Flutter applications, but they cater to slightly different needs. Sentry excels in real-time error tracking and performance monitoring, providing detailed insights that are essential for debugging and maintaining app stability. New Relic, meanwhile, offers a more comprehensive monitoring platform that covers a wide range of observability needs, making it ideal for organizations looking to monitor not only their applications but also the underlying infrastructure and user interactions.
By integrating monitoring tools into a Flutter application, developers can enhance their ability to detect, diagnose, and resolve issues quickly, ensuring a smooth and reliable user experience.
Authors
Monitor and Optimize Your Flutter Applications with Expert Solutions
Ensure your Flutter applications are always running at peak performance with expert monitoring solutions. At Walturn, we specialize in integrating powerful tools like Sentry and New Relic to provide real-time insights, track performance, and resolve issues proactively. Whether you need focused error tracking or comprehensive infrastructure monitoring, we have the expertise to help you implement the right solution tailored to your needs. Reach out today to enhance your app’s stability and performance.
References
Hamid Musayev. “Exception Tracking with Sentry in Flutter (Global Exception Handling).” Medium, Medium, 20 Sept. 2023, medium.com/@hamidmusayev/this-article-has-given-practical-and-detailed-information-about-what-are-errors-in-flutter-2d0d0998d058.
Josephine, Maureen. “Error Reporting Using Sentry in Flutter - Podiihq - Medium.” Medium, podiihq, 2 July 2021, medium.com/podiihq/error-reporting-using-sentry-in-flutter-c4e7c8030b88.
New Relic. “Monitor Your Flutter Mobile App | New Relic Documentation.” Newrelic.com, 2024, docs.newrelic.com/docs/mobile-monitoring/new-relic-mobile-flutter/monitor-your-flutter-application/.
---. “Slack Notifications Performance Monitoring | New Relic.” New Relic, 2024, newrelic.com/instant-observability/slack-notifications.
“Newrelic_mobile - Dart API Docs.” Pub.dev, 2024, pub.dev/documentation/newrelic_mobile/latest/.
Sentry. “Slack.” Sentry.io, 2024, docs.sentry.io/organization/integrations/notification-incidents/slack/.
---. “User Interaction Instrumentation | Sentry for Flutter.” Sentry.io, 2024, docs.sentry.io/platforms/flutter/integrations/user-interaction-instrumentation/.