Introduction to Flutter Animations
Flutter
Animation
Guide
Summary
This article introduces Flutter animation fundamentals, discussing widget types and techniques to create dynamic interfaces. It covers tween-based, physics-based, and pre-canned animations, highlighting the different widgets commonly used. It also explains how to choose between code-based and drawing-based animations and when to use implicit versus explicit animations, enhancing user experience in Flutter apps with effective animation patterns.
Key insights:
Animation Basics in Flutter: Flutter animations work by rapidly updating the widget tree to create movement, reaching up to 120 frames per second for smooth animations. This approach provides a responsive, high-quality user experience.
The Role of
Ticker
and Frame Updates: Tickers control animation frame rates in Flutter. UsingSingleTickerProviderStateMixin
, developers can synchronize animations with widget lifecycles, improving performance and efficiency.AnimationController for Precise Control:
AnimationController
allows playback options such as play, pause, reverse, and stop. It enables real-time control of animations and can be paired withTween
orCurve
for smoother transitions.Creating Custom Animations: Custom animations can be created by using
setState
to update widget properties frame-by-frame. This approach allows developers to animate unique properties and offers precise control over visual effects.Tween, Physics-Based, and Pre-Canned Animations: Flutter offers three main animation styles: tween-based for smooth transitions with defined start and end states, physics-based for realistic movements using physics principles, and pre-canned animations for quick, predefined effects that align with Material Design standards.
Implicit vs. Explicit Animations: Implicit animations simplify development by automatically animating changes, suitable for basic transitions. Explicit animations require
AnimationController
and are more customizable, ideal for complex, coordinated animations.Using
TweenAnimationBuilder
for Simplified Animation:TweenAnimationBuilder
reduces complexity, making it easy to animate properties without needing anAnimationController
. It’s useful for straightforward animations that require minimal setup.Animation Patterns in UI/UX Design: Common animation patterns, such as Animated Lists for dynamic content and Hero animations for shared transitions, enhance app interaction and improve user experience in apps that require engaging effects.
Choosing the Right Animation Widget: Selecting the right widget—such as
AnimatedBuilder
,TweenAnimationBuilder
, or third-party tools like Rive and Lottie—depends on whether the animation is drawing-based or code-based, impacting both aesthetic and performance.Enhancing Animations with Curves: Adding curves like
Curves.easeInOut
modifies the animation pace, making transitions feel more natural. Custom curves further allow unique effects, adding dynamic motion to animations.Optimizing Animation Performance: Using the
child
parameter inTweenAnimationBuilder
orAnimatedBuilder
helps optimize performance by preventing redundant re-renders of static content during animations.
Introduction
Creating smooth and engaging animations is one of the most exciting aspects of building apps in Flutter. To assist you in selecting the best animation widget for your requirements, we will go over the main ideas and methods for implementing animations in Flutter in this post. Let us start by going over the fundamentals of animation and how Flutter uses quick updates to the widget tree to simulate movement. We will then explore the various animation styles that Flutter offers, such as tween-based, physics-based, and pre-canned animations, each of which offers a special method for producing dynamic and captivating user interfaces.
Additionally, we will examine popular UI/UX design animation patterns and offer suggestions for successfully implementing them in Flutter. Lastly, we will go over how to pick the best Flutter animation widget for your type of animation, including whether it is code-based or drawing-based, and whether to use programs like Rive or Lottie for animations that are more graphically intensive. With the help of this thorough guide, you will be able to choose the most appropriate animation technique for your Flutter apps.
What is the Essence of Motion in Flutter: Animation Basics
In Flutter, ‘animation’ is essentially a series of frames that are shown quickly one after the other to provide the impression of movement. With frame rates of up to 120 frames per second on contemporary devices, Flutter depends on its ability to redraw widgets quickly enough to replicate continuous motion, much like movies do. Flutter animations make use of this idea by updating the widget tree with each frame. Widgets like AnimatedBuilder
, which invokes setState()
on each frame, effectively handle this resource-intensive method, enabling responsive, fluid animations without the need for specialist hardware.
1. The Role of Ticker: Controlling Frame Updates
In Flutter, a Ticker
is an object that executes a callback function on each frame. Since Ticker
is typically controlled by the SingleTickerProviderStateMixin
, which facilitates synchronizing animations with the widget lifetime, developers typically avoid using it directly. This is a simple illustration of a ticker that prints a message on each frame:
Always dispose of the Ticker
after use to avoid memory leaks. This is where SingleTickerProviderStateMixin
comes in handy; it makes maintenance easier by automatically assigning the Ticker
to the state of your widget.
2. AnimationController: Driving Animation Progress
The Flutter animation engine, the AnimationController
, provides playback control, including play, pause, reverse, and stop. Developers can view an animation's progress in real time by utilizing AnimationController
. For educational purposes, we show how to control animation via a direct setState()
call instead of the more popular method of pairing AnimationController
with a Curve
or Tween
:
3. Creating a Custom Animation with setState
This example determines the current animation value and calls setState()
with each frame to animate text to imitate accelerating from zero to the speed of light.
This example illustrates Flutter's ability to update states constantly at high speed, emulating fluid animations, by calculating the speed based on the current animation progress.
4. TweenAnimationBuilder: A More Concise Solution
TweenAnimationBuilder
offers comparable capability in fewer lines of code for a simpler method. You can reduce complexity and improve maintainability by defining a Tween
and duration
instead of requiring an AnimationController
or Ticker
:
By using Ticker
, AnimationController
, and setState()
on every frame, developers may create unique animations with Flutter's animation system. Although TweenAnimationBuilder
makes development easier, by fully controlling the updates for each frame, developers can create more inventive and effective animations by being aware of these lower-level techniques.
Types of Animations
Animations in Flutter can be broadly divided into three categories: tween-based, physics-based, and pre-canned. Each of these categories offers unique approaches to bringing user interface elements to life. These animations are used by developers to produce dynamic and aesthetically pleasing user interfaces. An outline of several animation styles and standard practices for successfully utilizing each are given in this section.
1. Tween Animation
With a beginning and ending state, a timeline, and a curve to regulate the timing and transition speed, tween animations—short for "in-betweening"—are characterized. Flutter computes intermediate values for tween animations, guaranteeing a seamless transition from beginning to end. For simple movements like fading, resizing, or color changes, this technique works well. With just the beginning and ending values, a duration, and a curve needed, Flutter's TweenAnimationBuilder
widget offers a simple method for producing such animations. This method eliminates the need for laborious computations and produces effects that are refined but basic.
2. Physics-Based Animation
When user interface elements require realistic dynamics, such as bouncing or springing, physics-based animations can be helpful since they replicate real-world movement. Depending on the desired effect, these animations can be tailored to react organically to forces. The AnimationController.animateWith
and SpringSimulation
APIs, developers can give animations physical characteristics like gravity and friction to make objects behave like they would in the actual world. These physics-based animations provide interactions with more nuance and make the movement seem logical and natural to users.
3. Pre-Canned Animations
Additionally, Flutter provides pre-made animations, especially via the animations
package on pub.dev
. This package offers a collection of frequently used animations, such as fade-throughs, shared axis transitions, container transformations, and fade transitions. For basic UI elements like dialogs or activity transitions, pre-canned animations make it easier to develop animations that follow Material Design requirements. Developers may ensure compatibility with Flutter's design language while saving time by utilizing pre-built choices.
Common Animation Patterns
In UI/UX design, specific animation patterns are commonly used to produce captivating user experiences. Flutter offers particular classes and widgets to easily apply these patterns.
1. Animated List or Grid
One common pattern for interactive content is to animate the addition or removal of items in a grid or list. The AnimatedList
widget in Flutter provides a simple method for animating list item changes. For instance, AnimatedList
animates the transition to graphically represent changes made to an item. For list-based content, such as task lists or shopping carts, where content updates must be both educational and aesthetically pleasing, this design is perfect.
2. Shared Element Transition
An animation pattern known as the "shared element transition" allows an element, like a picture, to "move" from one page to another while undergoing changes in size, shape, or location. This may be accomplished in Flutter by using the Hero
widget, which enables smooth route (page) transitions. The Hero
widget improves the operation and appearance of apps by enabling the creation of fluid navigation effects, in which items expand or change to reveal more material.
3. Staggered Animation
In order to provide a cascade effect, staggered animations divide a longer animation sequence into smaller portions, with each sub-animation being delayed. With the help of controllers and tweens, Flutter allows developers to create staggered animations, in which each segment of the animation overlaps or plays sequentially. By establishing a rhythm that directs the user's attention from one piece to the next, this technique is especially helpful for onboarding screens or list item reveals.
How to Choose Which Flutter Animation Widget is Right for You
Knowing the type of animation you want to use is crucial when selecting an animation widget for your Flutter application. In general, there are two primary categories of animations in Flutter: drawing-based and code-based. Code-based animations usually make use of the built-in Flutter widget primitives and focus on improving the look or transitions of pre-existing widgets, such as altering text styles or colors. However, drawing-based animations are perfect for more graphic-heavy animations, like gaming characters or intricate visuals, because they mimic hand-drawn objects or sprites. Use third-party tools like Rive or Lottie, which provide high-level interfaces for graphic-based animations that you can integrate into Flutter, if your animation appears more like a stand-alone visual design. However, Flutter's native code-based options might be more appropriate if the animation calls for simple widget modifications. The sets of decisions that you can take to choose you flutter animation are expressed in the decision tree below:
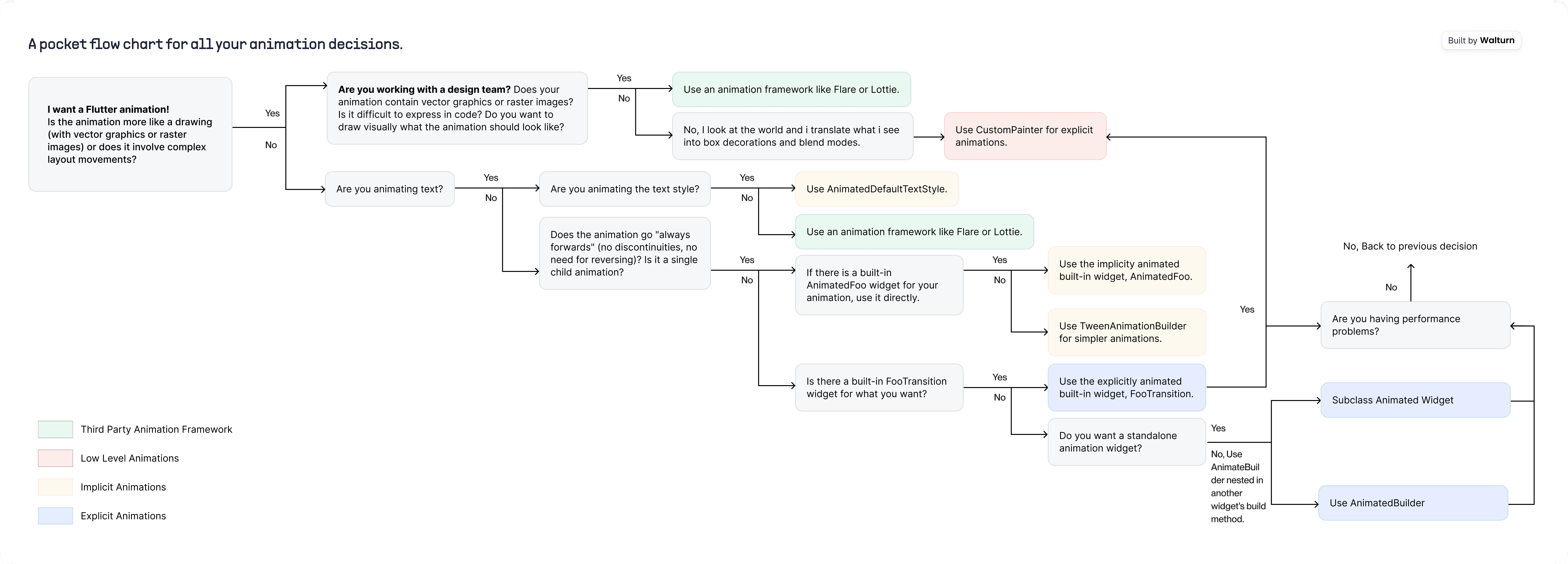
1. Implicit or Explicit Animations?
The dilemma of whether to utilize implicit or explicit animations arises once you decide that your animation will be code-based. Implementing implicit animations is easy because all that is needed is to set a new property value, after which Flutter will automatically animate it from the old value to the updated one. Because of their simplicity and low scripting requirements, these animations are great for novices and work quite well for simple transitions. Explicit animations, on the other hand, offer more control but necessitate a manual animation lifecycle management tool called an AnimationController
. Animations that need additional customization, synchronization with other widgets, or continuous behavior—like recurring animations—benefit from this method.
2. Making the Final Widget Selection
It all boils down to determining whether a built-in widget meets your needs before selecting one. Flutter provides a number of widgets for implicit animations, like AnimatedOpacity
and AnimatedContainer
, which manage typical animation requirements with little setup. Consider using TweenAnimationBuilder
to create bespoke implicit animations if the built-in widget is not suitable for your purposes. If they fit your property requirements, search for widgets like SlideTransition
or ScaleTransition
for explicit animations. If not, using AnimatedBuilder
or extending AnimatedWidget
would be your best bet if your animation includes intricate or synchronized movements. Additionally, if speed is an issue, think about utilizing CustomPainter
to draw straight to the canvas instead of the standard widget structure, which can optimize some animations.
In conclusion, you can choose the best Flutter animation widget for your app with confidence if you follow a methodical process that starts with the animation type, then decides whether implicit or explicit control is required, and ultimately chooses particular widgets. In addition to making development easier, this decision-making process guarantees that your animations are fluid, effective, and consistent with the desired visual experience.
3. Understanding When to Use AnimatedBuilder or AnimatedWidget in Flutter
When you want to animate a complex widget tree or multiple properties in Flutter, the AnimatedBuilder
widget is perfect because it allows you to wrap the entire widget tree you want to animate within a builder
function, which saves resources by allowing you to make efficient and repeated animations without having to rebuild the tree every time you update a frame. The builder
function, which takes the controller values, is only called during animation frames.
Nonetheless, When the animation logic can be contained in a smaller, more modular widget, the AnimatedWidget
class is helpful. By extending AnimatedWidget
, you can obtain a clean structure without requiring a separate AnimatedBuilder
or complicated build
function, which is especially helpful when you want to create a widget that is dedicated to a single animation because it minimizes boilerplate and keeps the code organized. Because it allows you to animate only specified elements of the widget hierarchy, the AnimatedBuilder
is very helpful when you need to create intricate animations inside a bigger widget tree. It is the preferred option if your animation uses a lot of widgets or if it would be inefficient to render the entire widget tree again for every frame.
In conclusion, If you require an easily reusable, self-contained animation widget, go with AnimatedWidget
. If the functionality of your animation can be condensed into a single, stand-alone widget, AnimatedWidget
aids in code organization and modularity. Additionally, this widget can be used for new widgets that extend pre-existing animations or when layering build
functions inside of build
functions is not desired.
Flutter Animation Basics with Implicit Animations
Flutter's implicit animations offer a quick and easy method of animating widget properties without the need for intricate coding or in-depth animation expertise. By simply adjusting property values, implicitly animated widgets can create fluid and captivating animations by handling transitions automatically. In order to give Flutter apps life, this part delves into the basics of implicit animations, emphasizing the robust AnimatedContainer
widget and providing information on how to handle durations, curves, and custom animations.
1. Understanding Implicitly Animated Widgets
Improved versions of ordinary widgets, implicitly animated widgets in Flutter are made to smoothly animate property changes. AnimatedContainer
, AnimatedOpacity
, and AnimatedPositioned
are a few examples. Developers can create seamless transitions with few code modifications by enclosing standard widgets in implicitly animated counterparts. These widgets improve user experience without the hassle of managing animation lifecycles by automatically adjusting between old and new values as properties are altered. For example, the code that follows shows how to simply alter a Container
width to make it animated:
Here, AnimatedContainer
transitions its width over one second, which creates a smooth resizing effect with minimal setup.
2. Using Duration and Curves to Control Animation Flow
The speed and style of transitions can be altered using attributes like duration
and curve
provided by AnimatedContainer
and other implicitly animated widgets. curve
uses a mathematical function to alter the transition's feel, while duration
determines how long the animation will take to finish. For instance, the animation feels more natural when a quintic curve (Curves.easeInOutQuint
) is used, which accelerates at first and slows down as it finishes. Simple changes to these parameters improve the animation's flow and add visual interest:
This code snippet alters the animation's smoothness, and it transforms the transition into a more dynamic experience.
3. Custom Curves for Unique Animation Styles
Custom curves provide additional customization when the default curves are unable to satisfy particular requirements. Curve
can be subclassed to allow developers to provide special behaviors. The following SineCurve
example uses the sine function to provide a bouncing effect to the animation, creating a rhythmic, oscillating motion:
This curve can be used to give an animation a fun, unique touch, making it ideal for applications that want to amuse or visually engage consumers.
4. Recap and Next Steps
Flutter's implicit animations make animation creation easier by automating changes between property values. One notable feature of AnimatedContainer
is its adaptability, which enables fluid, editable animations with little coding. By simply altering properties within predetermined durations and curves, widgets like AnimatedOpacity
, AnimatedPositioned
, and AnimatedPadding
also provide simple, aesthetically pleasing transitions. Investigating explicit animations could be helpful for intricate animations or those that require cooperation between several widgets. Deeper customization and fluidity are made possible by Flutter's many animation features, which guarantee a responsive and aesthetically pleasing user experience.
Introduction to Custom Implicit Animations in Flutter
There are multiple ways to implement animations with Flutter, and each is appropriate for a certain situation. For simple, one-widget animations that do not repeat endlessly, TweenAnimationBuilder
is especially helpful. In order to demonstrate TweenAnimationBuilder
's adaptability and control over animations without requiring AnimationController
, this article examines the situations in which it is better than other implicit animation widgets. Here are some real-world instances and how it operates.
1. Setting Up Basic TweenAnimationBuilder
To begin using TweenAnimationBuilder
, use the tween
argument to define the animation time and the range of values. A snapshot of your animated widget at any given time is provided by the builder
function. Here is an example that changes the color of a widget over a predetermined amount of time:
In this setup, TweenAnimationBuilder
interpolates between Colors.white
and Colors.red
. It smoothly transitions the container’s color over two seconds.
2. In-Depth Example: Animating a Color Filter
Let us make an app that shows how a star's color changes as it moves in space, a phenomenon known as the Doppler effect. We accomplish this by gradually altering the star's color using ColorFiltered
with a BlendMode
. TweenAnimationBuilder
makes it simple to animate between two colors, but Flutter does not include a built-in widget for color filter animations:
By nesting ColorFiltered
within TweenAnimationBuilder
, you animate the star image’s color between white and orange. This simulates the Doppler effect.
3. Dynamic Tweens and Interactive Animations
You may improve interactivity by dynamically updating the animation's end value with TweenAnimationBuilder
. Here is an example where users can change the color in real time using a slider to modify the animation's color filter. To guarantee seamless transitions, the animation continually interpolates from the current to the new end value.
This example updates ColorTween
's end color based on the slider's position. It provides an interactive color animation.
4. Enhancing Animations with Curves and Callbacks
Additionally, TweenAnimationBuilder
has a curve
parameter that lets you adjust the animation pacing, and the onEnd
callback lets you do things like start a new animation or toggle widgets when the animation is finished. If you want an animation that repeats continuously, though, you should think about using explicit animation controls.
In this example, the scale
animation uses Curves.easeInOut
for a smooth transition.
5. Optimization with the Child Parameter
Use the child
parameter for static content that does not require frame-by-frame rewriting to maximize efficiency. Passing the picture as a child, for example, minimizes rebuilds when animating just the color filter of an image, concentrating only on the filter updates.
This ensures the image remains static, improving performance, especially when animating complex widgets.
TweenAnimationBuilder
is a powerful tool for creating smooth animations without explicit control; it is perfect for one-time, "set-it-and-forget-it" animations and offers straightforward yet powerful options with dynamic Tweens and performance optimizations. You can easily create captivating animations in Flutter by leveraging the curve
and onEnd
parameters or by adjusting the end value.
More resources
Discover more about Flutter animations by looking through the Flutter Sample app catalog, which offers a variety of animation samples for you to study and get ideas from. Additionally, the Flutter YouTube channel offers animation lessons and instructions that include step-by-step walkthroughs and visual explanations. Furthermore, the Flutter API documentation provides a thorough rundown of the animation library, along with examples and comprehensive documentation to help you better grasp how to use animations in Flutter.
Conclusion
In conclusion, mastering Flutter animation necessitates familiarity with a wide range of techniques and materials in order to create compelling and successful user experiences. We began by discussing the basics of motion in Flutter and how animations use fast frame changes to simulate movement. We next looked at the many animation styles that Flutter offers, such as tween-based, physics-based, and pre-canned, and how each satisfies different design specifications. We looked at common UI/UX motion patterns and the Flutter widgets that implement them after establishing that foundation.
Making the right animation widget choice is crucial to optimizing both performance and aesthetic appeal. Regardless of whether you prefer code-based or drawing-based animations, the user experience of your app can be greatly impacted by selecting the appropriate widget, such as the AnimatedBuilder
, or third-party tools, such as Rive and Lottie. The flexibility of TweenAnimationBuilder
for more complex animations and the simplicity of basic transitions with widgets like AnimatedContainer
were highlighted in the discussion about implicit animations. Finally, we examined the adaptability and efficiency of AnimatedBuilder
in animating complex widget trees. Flutter developers can create dynamic and fluid animations that enhance the user experience overall if they have the right tools and understand these concepts.
Authors
References
Flowers, Alethea K. “Flutter Animation Basics with Implicit Animations - Flutter - Medium.” Medium, Flutter, 4 Dec. 2019, medium.com/flutter/flutter-animation-basics-with-implicit-animations-95db481c5916. Accessed 11 Nov. 2024.
Fortuna, Emily. “Custom Implicit Animations in Flutter…with TweenAnimationBuilder.” Medium, Flutter, 15 Dec. 2019, medium.com/flutter/custom-implicit-animations-in-flutter-with-tweenanimationbuilder-c76540b47185. Accessed 10 Nov. 2024.
---. “When Should I UseAnimatedBuilder or AnimatedWidget?” Medium, Flutter, 19 Jan. 2020, medium.com/flutter/when-should-i-useanimatedbuilder-or-animatedwidget-57ecae0959e8. Accessed 10 Nov. 2024.
Gibbon, Andrew Fitz. “How to Choose Which Flutter Animation Widget Is Right for You?” Medium, Flutter, Apr. 2020, medium.com/flutter/how-to-choose-which-flutter-animation-widget-is-right-for-you-79ecfb7e72b5. Accessed 10 Nov. 2024.
“Introduction to Animations.” Docs.flutter.dev, docs.flutter.dev/ui/animations.