Deep Comparative Analysis Between BloC & Stacked
Summary
This article explores BloC and Stacked, two popular state management frameworks in Flutter. BloC, built on reactive streams, offers robust state control for complex apps, while Stacked, based on MVVM, prioritizes simplicity and rapid prototyping. It compares their design patterns, scalability, testing ease, and developer productivity to guide developers in selecting the right framework based on project demands.
Key insights:
BloC Principles: BloC uses reactive streams and unidirectional data flow, ensuring a strong separation between UI and business logic.
Stacked Principles: Stacked adopts the MVVM pattern with ViewModels and reactive services, emphasizing simplicity and modularity.
Scalability: BloC excels in scalability for large, complex apps, while Stacked is better suited for smaller to medium-sized applications.
Testing: BloC's decoupled architecture supports detailed testing but may pose challenges with debugging complex streams. Stacked simplifies testing with reactive ViewModels.
Developer Productivity: Stacked allows faster prototyping with less boilerplate, while BloC’s structure prioritizes long-term maintainability.
Learning Curve: BloC has a steeper learning curve due to its streams and verbosity, while Stacked is easier for teams new to state management.
Ideal Use Cases: BloC fits large-scale, test-driven applications, while Stacked caters to rapid development and smaller team setups.
Flexibility vs. Rigidity: Stacked offers a more flexible design, while BloC enforces strict, testable modularity.
Introduction
Any dynamic application's foundation is state management, which guarantees smooth communication between the user interface and the underlying data logic. As programs develop, state management becomes essential for preserving scalability, debugging effectiveness, and UI consistency. Effective state management in software development enables developers to isolate and handle complicated interactions with efficiency while guaranteeing a predictable and responsive user experience.
Two well-known methods for managing states, especially in the Flutter ecosystem, are BloC (Business Logic Component) and Stacked. BloC emphasizes the distinct division of UI and logic by utilizing streams and reactive programming concepts. On the other hand, Stacked is based on the MVVM design and prioritizes developer productivity and simplicity through structured services and reactive ViewModels. Both solutions are essential for creating reliable Flutter applications since they address specific project requirements.
A thorough analysis of BloC and Stacked reveals their core ideas, trade-offs, and best applications. Developers and software architects can match their selection of a state management solution with project objectives, team proficiency, and application complexity by looking beyond these solutions' obvious advantages.
This article attempts to present a thorough, comparative examination of BloC and Stacked for developers and software architects looking to gain a deeper understanding of state management architecture. Through a thorough technical examination of these frameworks, the conversation aims to enable readers to make well-informed choices that strike a balance between maintainability, scalability, and performance.
Core Principles
This section examines the fundamental ideas of BloC and Stacked, two well-known state management frameworks in the Flutter ecosystem. Using different design philosophies that represent their distinctive beginnings and development, each framework provides special ways for handling state in intricate applications. While Stacked expands on Provider's simplicity by adding features that increase maintainability and scalability, BloC, which has its roots in reactive programming, uses streams to handle state transitions in an organized, predictable way.
1. Origin and Inspiration from Reactive Programming (BloC)
The reactive programming paradigm, which stresses managing asynchronous data streams with decomposable actions, is where the BloC (Business Logic Component) pattern got its start. Predictably handling states and events is the foundation of reactive programming, and BloC's architecture is directly influenced by this methodology. BloC ensures a systematic separation between the business logic and user interface layers, making Flutter application state management easier. BloC uses streams as a foundational idea to enable declarative data flow and transformations, which facilitates the development of tested, maintainable, and scalable applications.
2. Fundamental Principles: Streams, Events, and States (BloC)
BloC is based on a streams-based design, where states are the reactive outputs of the user interface and events cause state changes. A unidirectional data flow is enforced by the framework: events are sent to the BloC, where they are processed to create new states, which the user interface (UI) then listens to and rebuilds as needed. Complex applications behave predictably because of the distinct division between input (events) and output (states). To keep UI and logic separate, for example, widgets like BlocBuilder and BlocListener are used to listen to states or act on changes, respectively.
3. Use Cases and Integration Patterns (BloC)
Applications requiring intricate state transitions, such as dynamic form management or multi-page workflows, are where BloC excels. It performs exceptionally well in situations when state predictability is crucial, such as multi-user collaborative apps or real-time data syncing. Tools like BlocProvider, which permits dependency injection, and BlocSelector, which permits fine-grained UI modifications, make integration into the widget tree smooth. By enforcing uniform state management rules, these patterns not only improve scalability but also facilitate teamwork.
4. Evolution from Provider in the Flutter Ecosystem (Stacked)
Building on Provider, a state management package that became well-liked for its ease of use and reactive features, Stacked developed as an improved alternative. However, provider’s usefulness in more extensive applications exposed its scalability and maintainability constraints, particularly when handling numerous interdependent components. By including strong abstractions like dependency injection, reactive services, and ViewModels, Stacked fills these gaps and establishes itself as a comprehensive framework for creating production-quality Flutter apps with an organized and testable design.
5. Core Principles: ViewModels, Reactive Services, and Dependency Injection (Stacked)
The architecture of Stacked is based on three fundamental foundations. ViewModels ensure a clear division of responsibilities by acting as a link between the business logic and the user interface. Reactive services, which enable dynamic updates without closely linking components, are integrated with them. Lastly, dependency injection improves scalability and testability by making shared resource management easier. With its emphasis on simplicity and flexibility, this design makes it simpler to manage feature-rich applications with less boilerplate.
6. Use Cases and Simplicity-Focused Design (Stacked)
Stacked works especially well for feature-focused application development, when quick prototyping and ease of usage are key considerations. Because of its modular nature, it is perfect for individual developers or small teams working on applications that need to be highly maintainable, like dashboards or CRUD-based systems. While debugging or expanding functionality, the ViewModel-centric method lessens cognitive stress by promoting a natural organization of code. This ease of use, along with the services' inherent reactivity, guarantees that developers can produce well-designed apps quickly without compromising code quality.
Architectural Comparison
We will examine the architectural distinctions between BloC and Stacked in this section, paying close attention to how each method organizes the application and handles state. Although both systems provide strong answers for maintainability and scale, their modularity methodologies and design philosophies represent divergent goals. BloC emphasizes a reactive, unidirectional data flow with great modularity and is based on the Observer and Redux design patterns. Stacked, on the other hand, uses a service-oriented design and the MVVM pattern, offering a more adaptable and practical method of creating scalable applications.
1. Design Patterns: Contrasting Philosophies
BloC and the Observer-Redux Hybrid: To handle application state, the BloC (Business Logic Component) architecture mainly uses the Redux and Observer design patterns. By broadcasting state changes as streams that listeners (widgets) can view and dynamically respond to, the Observer pattern makes reactive programming possible. The Redux feature promotes immutability and predictability by guaranteeing a unidirectional data flow. BloC is a great option for complicated applications with strong state control and high testability because of its dual-pattern approach, which promotes a clear separation between the UI, business logic, and data layers.
Stacked and MVVM-Driven Modularity: The Model-View-ViewModel (MVVM) design, on the other hand, is adopted by Stacked and presents ViewModels as intermediaries between the user interface and underlying services. A clear separation of duties is enforced by this method, with ViewModels managing state and coordinating interactions with services while Views handle rendering. Additionally, Stacked incorporates a service-oriented design, which lets programmers package reusable features. Scaling and modularization are made easier by this combination, particularly for applications that need a simple, extensible structure with less boilerplate.
2. Layered Architecture: Structuring for Scalability
Streamlined but Verbose Layering in BloC: BloC arranges applications into three separate layers: Presentation (Widgets), Business Logic (Blocs or Cubits), and Data (Repositories and Providers). These layers, which place a strong emphasis on modularity and concern separation, precisely correspond to software design concepts. Events and states may be seamlessly chained between components thanks to the architecture's focus on streams. However, especially in projects with simple needs, its verbosity—which manifests as a large number of classes and interfaces—can cause a burden for developers.
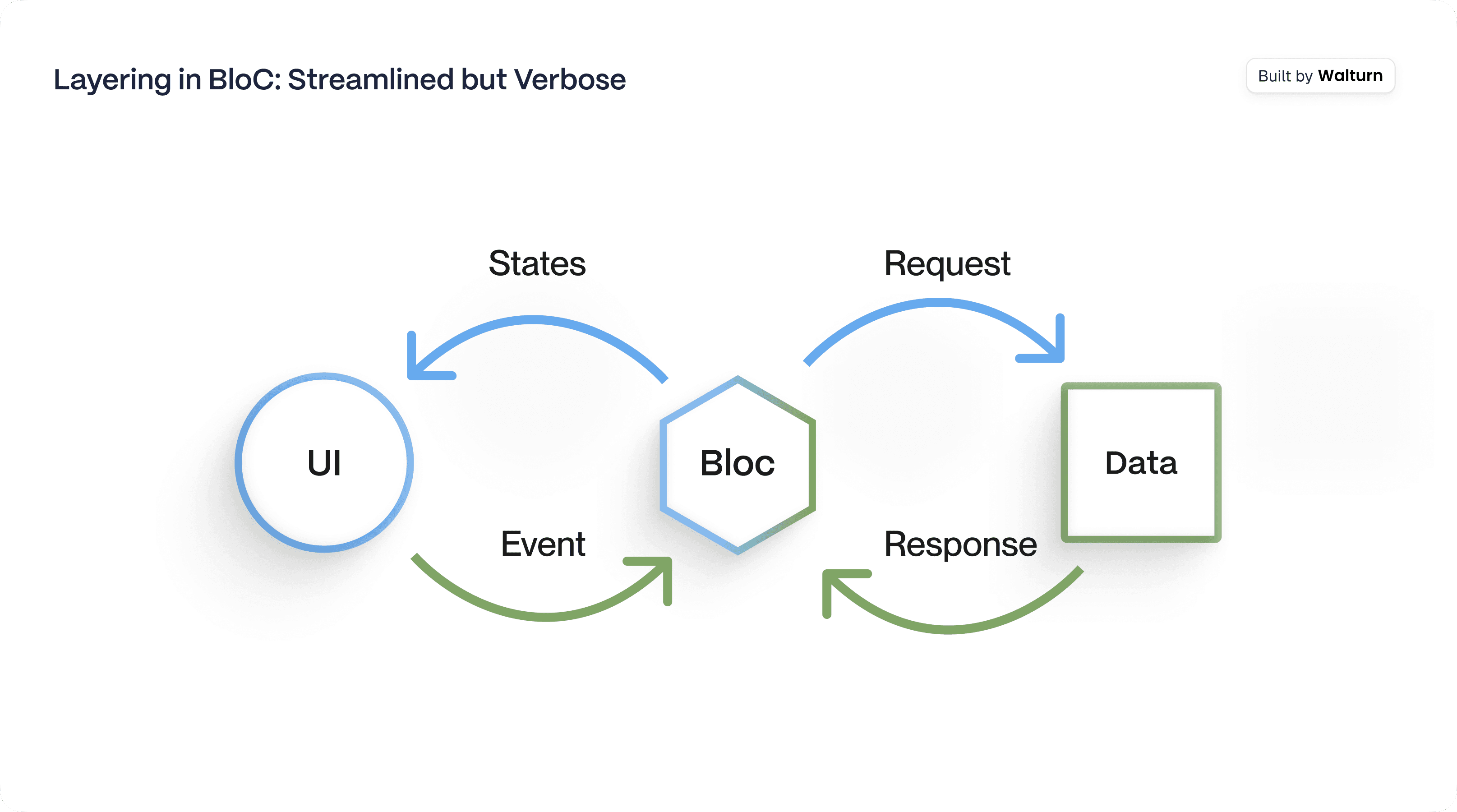
Pragmatic and Service-Oriented Layering in Stacked: Stacked likewise adheres to a three-layered architecture, with Views, ViewModels, and Services neatly correlating to the Presentation, Logic, and Data layers, respectively. Stacked reduces repetitive boilerplate code by making it easier to link Views to ViewModels by utilizing ViewModelBuilder widgets. While maintaining familiarity for Flutter developers, its usage of provider for state and dependency management aligns it with Riverpod's versatility. Although it might not have the granular state-stream handling that BloC offers, this architecture performs exceptionally well in mid-sized to large applications where clarity and maintainability are crucial.
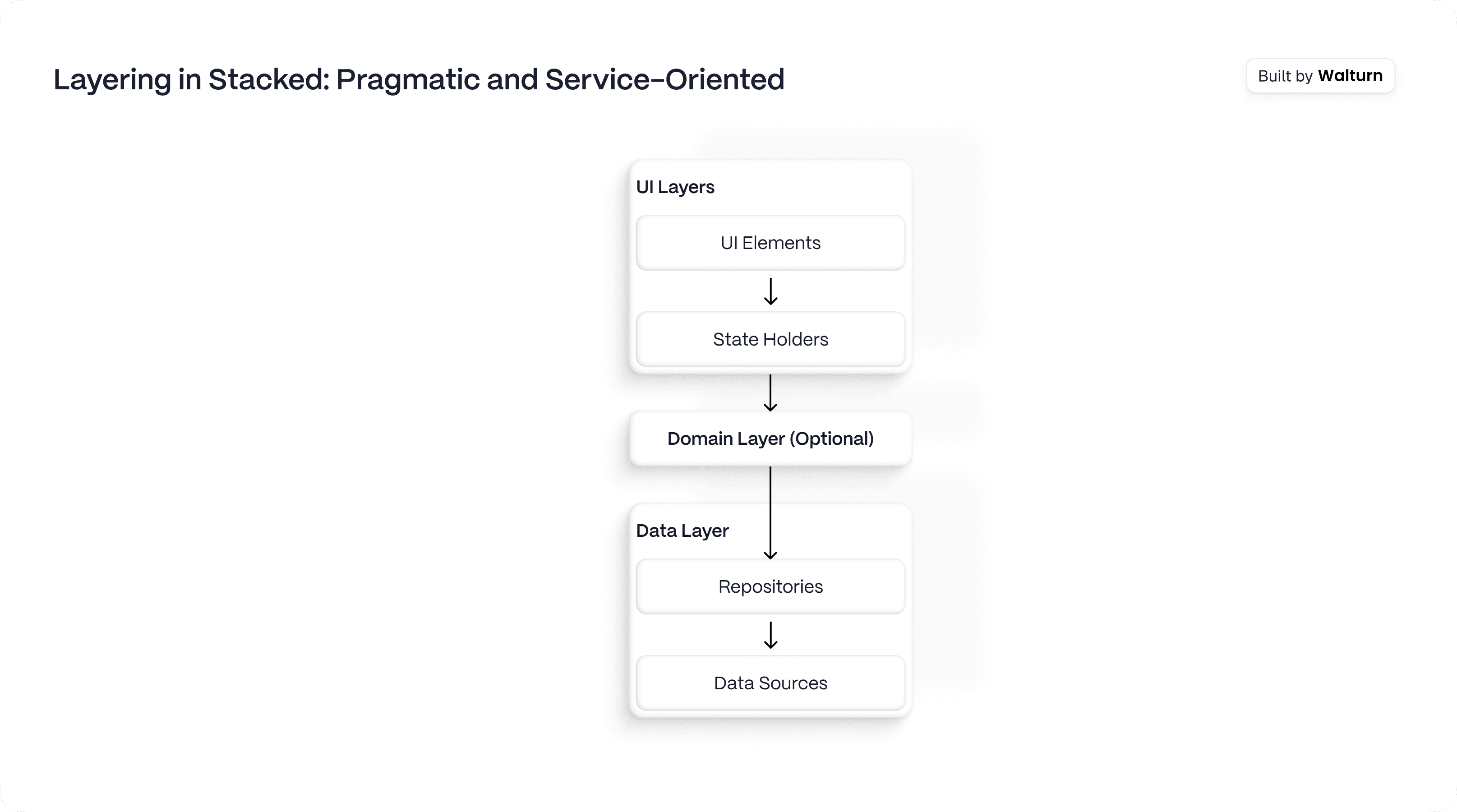
3. Modularity and Scalability: Contrasting Strengths
BloC - Rigorous and Test-Driven: Because each element is isolated into its own Bloc or Cubit, BloC's architecture naturally encourages modularity. This division guarantees the complete testability and reusability of business logic. Rapid prototyping or iterative development may be discouraged by the effort needed to construct streams for each state change, and its structured nature may appear inflexible in simpler projects.
Stacked - Lean and Flexible: Stacked excels at being versatile. It enables teams to create highly reusable components without imposing the same degree of rigor as BloC by emphasizing service-based logic encapsulation. Because of this, it is perfect for agile settings where developers value clear, understandable codebases and features change regularly. Faster development cycles and a lower barrier to entry make up for the loss of some of the granular control provided by BloC's stream-based paradigm.
Testing and Maintainability
The ease of testing and long-term maintenance of a framework is frequently used to assess its efficacy. Two well-liked state management strategies in Flutter, the BloC and Stacked structures, will be compared in this section with regard to testing and maintainability. While maintainability is critical for long-term success, particularly in dynamic development contexts, testing is necessary to guarantee an application's stability and resilience. This comparison will show each framework's advantages and disadvantages.
1. Testing with BloC
Ease of Unit Testing Due to Decoupled Architecture: The architecture of BloCs is naturally testable. This is because of its highly decoupled structure, in which the business logic and user interface are entirely distinct. The BloC itself, which controls state via streams and sinks, serves as the main testing unit of BloC. To test each method in a BloC, events are simulated and the subsequent states are asserted. It is simple to provide mock inputs and validate outputs when streams are used since the state changes are predictable. Because the BloC offers a transparent and isolated testing environment and allows you to directly feed events into it and listen to state outputs, test cases are simple.
Challenges in Debugging Complex Stream Chains: Debugging intricate stream chains in BloC can be difficult, despite the testing advantages. Because streams are asynchronous, they can cause memory leaks, race situations, and unhandled stream events, among other hard-to-trace problems. While unit testing guarantees that individual BloCs function as intended, it takes a lot of work to debug the data flow over several streams, especially in bigger systems. Because the interactions between streams might result in unanticipated behaviors that are difficult to recreate in a test environment, this problem is exacerbated when streams are deeply nested or depend on external services.
2. Testing with Stacked
Testability of ViewModels and Reactive Services: One of Stacked's main advantages is that ViewModels are testable. Like BloC, a ViewModel in Stacked is made to be easily tested separately and encapsulates display logic. Verifying its operations, like communicating with services or changing the user interface's state, can be used to test it. ViewModels may be tested by subscribing to changes in service data because Stacked's use of services is reactive, guaranteeing that UI elements accurately represent the state. Although services can be mocked and are injectable, integrating reactive programming (for example, by using ReactiveViewModel) adds complexity, particularly when testing numerous services simultaneously. Nevertheless, efficient testing procedures are made possible by the distinct division of responsibilities between ViewModels and services.
Maintenance Simplicity in Smaller Teams: Stacked provides a substantial maintenance benefit for smaller teams. Because of its limited boilerplate, its architecture makes managing state, services, and UI logic easier. A smaller team may more easily stay up to date with changes thanks to features like the BaseViewModel's built-in busy and error states, which lessen the amount of code developers must write and maintain. Additionally, by allowing teams to concentrate on specific components without worrying about tight coupling, Stacked's ability to modularize services and ViewModels improves maintainability. For small teams that might lack the resources to handle complex dependencies or large boilerplate, the integrated support for dependency injection and reactive patterns makes updates and scaling simpler.
In conclusion, BloC and Stacked both offer strong testing frameworks; however, BloC's decoupled architecture strengthens its testability, which makes it perfect for standalone business logic testing. On the other hand, Stacked is better at testing ViewModels since it has less boilerplate and integrated reactive handling, which makes it simpler to maintain, particularly for smaller teams. When working with complicated state management and asynchronous data flows, debugging in both frameworks can become challenging.
Strengths and Weaknesses
BloC and Stacked have unique advantages and disadvantages suited to various application requirements. BloC is perfect for large, complicated applications that need strong state management and a clear separation of responsibilities because of its exceptional scalability, modularity, and maintainability. However, development may be slowed down by its difficult debugging of large stream chains, boilerplate-heavy structure, and high learning curve.
Stacked, in comparison, offers a flexible ViewModel-based architecture, less boilerplate, and a focus on simplicity, quick prototyping, and developer productivity. Although it might not provide the same level of granular control as BloC for extremely complex state management, it is ideal for smaller teams or projects where speed and maintainability are top priorities. In the end, Stacked provides a simpler, more flexible solution for medium-to-smaller applications, whereas BloC is ideal for large-scale, test-driven applications. The following table contrasts BloC with Stacked's advantages and disadvantages:
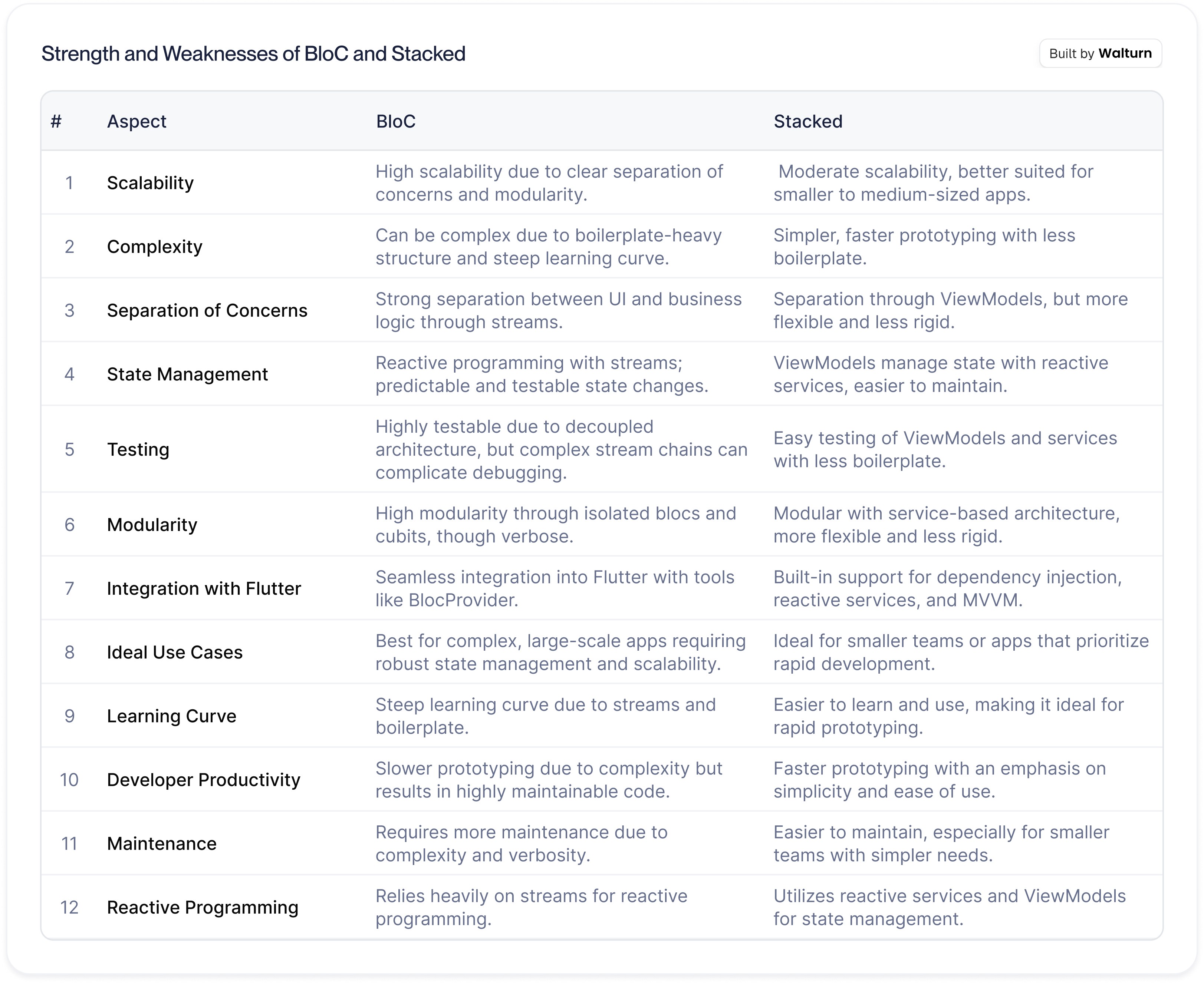
Conclusion
To sum up, BloC and Stacked both offer efficient state management options in Flutter, each with unique advantages and disadvantages. BloC is perfect for big, complicated applications that need a lot of modularity, scalability, and maintainability. It is ideal for projects requiring consistent state transitions and comprehensive testing capabilities due to its dependency on streams and distinct separation of concerns. However, it might be difficult for smaller teams and quick prototyping due to its verbose nature, steep learning curve, and debugging difficulty. On the other hand, Stacked places a higher priority on developer productivity, adaptability, and simplicity, which makes it a good fit for smaller teams or projects where quick prototyping and simple upkeep are essential. Though it might not provide BloC with the same level of granular control over state management in more complex scenarios, its ViewModel-driven architecture and low boilerplate minimize development overhead.
Authors
References
Alkhateeb, Bakri. “Understanding State Management: A Comprehensive Guide.” Medium, 21 Jan. 2024, medium.com/@bakrialkhateeb.dev/overview-of-state-management-in-software-development-54c489011b90#:~:text=State%20management%20refers%20to%20the,the%20current%20page%20being%20viewed.
“Architecture.” Bloc, bloclibrary.dev/architecture.
Bizzotto, Andrea. A Comparison of Popular Flutter App Architectures. codewithandrea.com/articles/comparison-flutter-app-architectures.
“Guide to App Architecture.” Android Developers, developer.android.com/topic/architecture.Sparkleo.
“5 Top Flutter State Management Tools: Finding the Right Fit for Your Project.” Medium, 29 Mar. 2024, medium.com/@sparkleo/5-top-flutter-state-management-tools-finding-the-right-fit-for-your-project-f1e7117c1433.
View Models | the Production Flutter Framework. stacked.filledstacks.com/docs/in-depth/viewmodels.