Building Smarter with CI/CD in Flutter
Summary
CI/CD pipelines are essential for Flutter development, enabling automation and streamlining processes like builds, testing, and deployment for cross-platform apps. These pipelines ensure high-quality code, faster releases, and fewer integration issues. By incorporating tools like Jenkins, GitHub Actions, and Codemagic, Flutter developers can handle platform-specific challenges, optimize testing, and secure workflows.
Key insights:
Importance of CI/CD: Essential for managing Flutter’s complexities across platforms, ensuring consistent builds, testing, and deployments.
Cross-Platform Challenges: CI/CD addresses unique Flutter hurdles like platform-specific builds, dependencies, and configurations.
Automation Benefits: Automates repetitive tasks, improves code quality, and accelerates release cycles with fewer errors.
Testing Efficiency: Integrates unit, widget, and integration testing to catch bugs early, ensuring reliability across devices and platforms.
Tool Selection: Tools like Jenkins, GitHub Actions, Codemagic, and Bitrise offer tailored features for various team sizes and project needs.
Security Management: Protects sensitive credentials with secure storage solutions like GitHub Secrets, ensuring safe deployments.
Best Practices: Modular pipelines, optimized caching, secure secret management, and robust documentation ensure scalable and efficient CI/CD workflows.
Emerging Trends: AI-driven pipelines, serverless CI/CD, DevSecOps integration, and low-code solutions will redefine future Flutter development workflows.
Cost Efficiency: Free and cloud-based solutions like GitHub Actions provide budget-friendly CI/CD options for small teams.
Continuous Feedback: Real-time monitoring and feedback loops enhance collaboration and quick issue resolution during development.
Introduction
Flutter’s growing popularity as a cross-platform development framework has revolutionized how developers build applications for multiple platforms. However, with this flexibility comes the challenge of maintaining consistent quality across different platforms while ensuring rapid, reliable, and consistent delivery. This is where implementing a strong CI/CD (Continuous Integration/Continuous Delivery) pipeline becomes important for Flutter applications. It enables teams to automate builds, tests, and deployment processes while maintaining high code quality and faster time to market. This insight aims to cover must-have CI/CD pipelines for Flutter applications that can make software development easier for developers.
Continuous Integration / Continuous Deployment
1. Overview
Continuous Integration/Continuous Deployment (CI/CD) is a set of practices and tools designed to automate and streamline the software development lifecycle. CI involves automatically integrating code changes from multiple developers into a shared repository where automated builds and tests verify each integration. On the other hand, CD extends this automation to either prepare releases for deployment (Continuous Delivery) or automatically deploy to production (Continuous Deployment).
CI/CD also allows teams to have “Blue/Green” deployments, where two different environments are maintained simultaneously to streamline the release process. The “blue” environment hosts the current live/production version of the application whereas the “green” environment contains new versions that are being prepared for deployment. This setup allows for seamless transitions between the two by allowing teams to switch traffic from one environment to the other without disruption. If any issues arise with the new release, traffic can quickly be reverted to the stable “blue” environment which ensures continuity and reliability for users. This approach not only minimizes downtime but also reduces the risk associated with deploying new features or updates.
2. History of CI/CD
The emergence of CI/CD stems from the need for faster and more reliable software delivery processes. Traditional waterfall methods, with their linear approach, required lengthy planning phases and restricted changes during development, often delaying projects. Agile methodologies, introduced in the early 2000s, revolutionized software development by promoting iterative cycles and continuous feedback, paving the way for modern CI/CD practices that align with these principles
3. Key Benefits of CI/CD
Continuous Integration and Continuous Deployment (CI/CD) offer numerous benefits for software development. By automating processes, development cycles become faster as manual intervention is reduced which allows for quicker release schedules. Code quality is enhanced through automated testing and code analysis, which not only catch issues earlier in the development phase but also ensure that your codebase is readable. Regular integration further minimizes merge conflicts and integration problems, ensuring smoother collaboration among team members which is essential for product engineering.
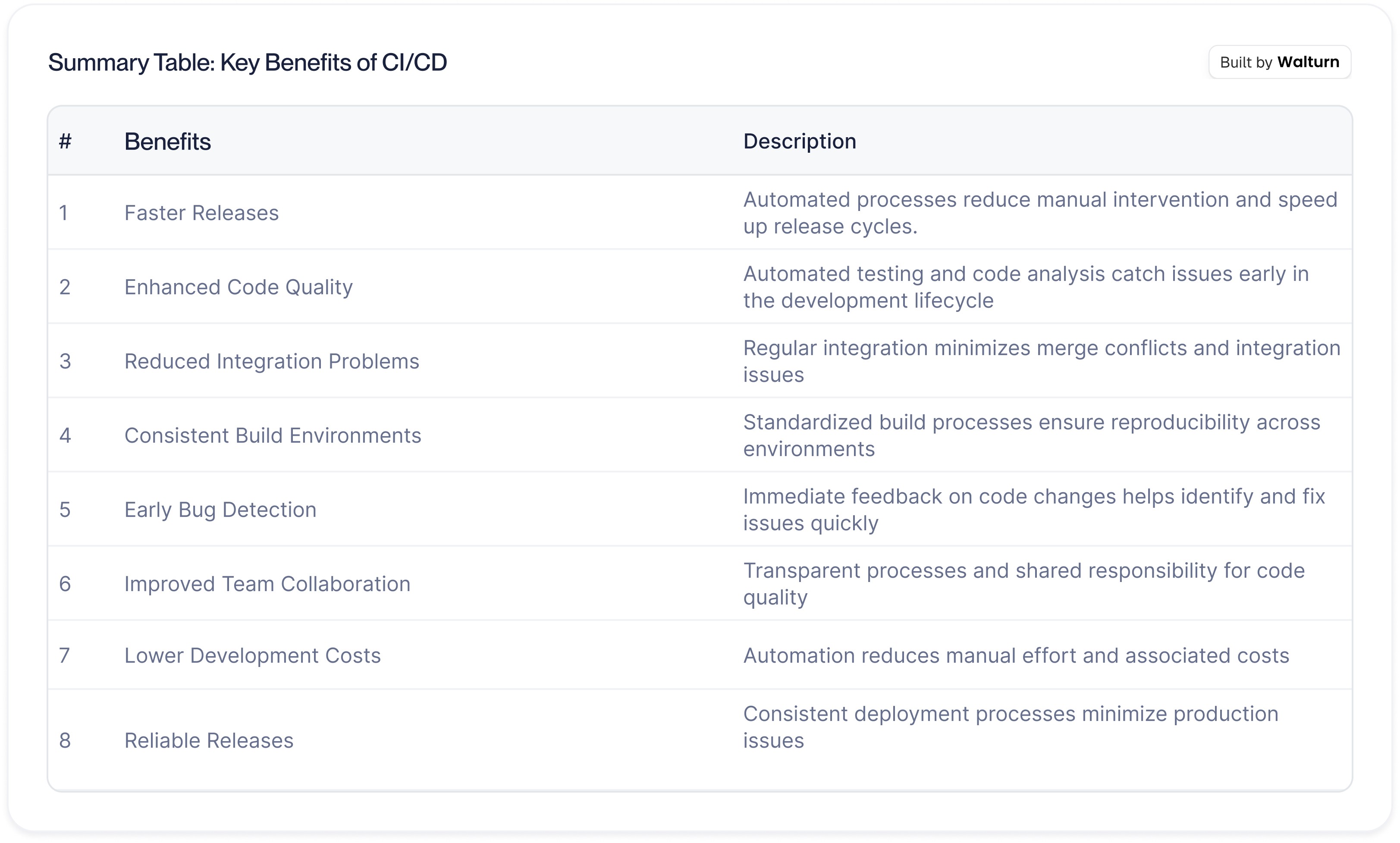
4. Why CI/CD is Essential for Flutter Projects
Flutter’s cross-platform capabilities are powerful but they also introduce complexities that make implementing CI/CD pipelines an essential part of the project lifecycle. By enabling the development of native applications for mobile, web, and desktop from a single codebase, Flutter simplifies development but presents unique challenges that are not typically encountered in single-platform projects. This section highlights the importance of leveraging CI/CD in Flutter apps to ensure smooth development and deployment processes.
Flutter applications often target multiple platforms (such as iOS and Android), each with distinct build configurations, dependencies, testing environments, and deployment requirements. Without automation, managing these platform-specific intricacies can become error-prone and time-consuming. CI/CD solves this by creating a standardized pipeline that automates builds for each platform to ensure consistent handling of configurations, dependencies, and assets. This consistency eliminates manual errors and ensures that applications behave uniformly across all target platforms.
The build process for Flutter is complex due to platform-specific requirements. Maintaining distinct release channels such as alpha, beta, and production adds to the challenge. CI/CD streamlines this by automating processes and managing release artifacts for each platform. With CI/CD, teams can automate workflows to produce platform-specific builds with predefined configurations, reducing human effort and accelerating release cycles.
Testing in Flutter projects is another area that can add to the complexity of projects. Developers need to conduct platform-specific UI tests, verify unique features and behaviors, and ensure compatibility across different screen sizes, device types, and operating systems. CI/CD addresses this by integrating automated testing into the pipeline, and running tests on real and virtual devices for every code change. This ensures that bugs are caught early and platform-specific quirks are identified before they impact users. This results in a higher-quality application with faster feedback for developers.
Dependency management also presents significant challenges in Flutter projects. Teams must handle updates to the Flutter SDK, coordinate third-party packages’ versions, and manage native dependencies for each platform. Inconsistencies in dependency handling can lead to build failures or runtime issues. CI/CD tools mitigate this by enforcing strict dependency checks, running builds in clean environments, providing early warnings for incompatibility, and automatically suggesting updates of third-party dependencies. This proactive approach ensures that the application remains stable and maintains compatibility with its ecosystem.
In summary, CI/CD enhances the development lifecycle of Flutter projects by introducing automation, consistency, and reliability. It allows developers to focus on building innovative features rather than spending time managing the intricacies of multi-platform development. By addressing the challenges of Flutter development head-on, CI/CD not only simplifies workflows but also ensures that the end product meets the highest standards of quality, performance, and user experience.
Key Components of a CI/CD Pipeline for Flutter Apps
A well-structured CI/CD pipeline for Flutter applications consists of several components that work together to ensure the reliable and efficient delivery of your applications. This section aims to explore each of these components in detail.
1. Source Control Management (SCM)
Source Control Management serves as the foundation of any CI/CD pipeline including those for Flutter projects. Git has emerged as the de facto standard for version control with platforms like GitHub, GitLab, and Bitbucket offering different advantages. GitHub excels in open-source collaboration, while GitLab provides integrated CI/CD capabilities, and Bitbucket often appeals to enterprise environments.
Branching strategies are also important for maintaining code stability and efficient collaboration. GitFlow uses a hierarchical structure with branches like main or master for production, develop for active development, and feature-specific or hotfix branches. This model suits teams managing complex release cycles. On the other hand, trunk-based development emphasizes simplicity with short-lived feature branches that frequently merge into the main branch to promote rapid iteration and reduce merge conflicts. This approach is particularly effective for Agile teams relying on continuous testing and integration.
2. Continuous Integration
Continuous Integration automates the process of integrating code changes from multiple contributors into a shared repository. CI ensures that every code commit triggers automated builds and validation checks which reduces the risk of integration issues. In Flutter projects, CI pipelines handle platform-specific builds including APKs for Android and IPAs for iOS to ensure compatibility across devices.
Furthermore, CI tools like Github Actions, Jenkins, and CircleCI excel in Flutter development. GitHub Actions integrates natively with repositories and offers a range of Flutter-specific actions while Jenkins allows for extensive customization with self-hosting options, and CircleCI’s scalable cloud-based architecture simplifies pipeline configuration and execution, especially for teams looking for rapid deployment cycles.
Beyond building, CI involves static code analysis with flutter analyze, linting, dependency audits, and automated formatting checks. These steps ensure code quality remains high throughout the development lifecycle.
3. Automated Testing
Testing is a cornerstone of reliable Flutter application development, safeguarding functionality across platforms. A well-structured testing pyramid, consisting of Unit tests, Widget tests, and Integration tests, ensures comprehensive coverage of your application.
Unit tests validate the logic of individual components. For example:
Widget tests verify the behavior of Flutter widgets in isolation such as UI responsiveness and component integration. For example:
Integration tests assess the application’s end-to-end functionality, incorporating real devices and emulators to detect platform-specific issues. Services like Firebase Test Lab enable automated execution of these tests across a wide array of device configurations.
To maximize effectiveness, teams should aim for high test coverage and integrate automated UI tests alongside regular performance profiling. Tools like Flutter Driver, Appium, and integration with CI platforms ensure continuous validation of application behavior.
4. Build Automation
Build automation handles the compilation and packaging of Flutter applications for multiple platforms. This component ensures consistent, error-free builds tailored to each platform’s requirements. Properly structured configuration files simplify build automation. For example, the following YAML snippet demonstrates typical build settings:
By automating repetitive build processes, developers can save time and reduce human error, enabling a streamlined delivery pipeline.
5. Continuous Delivery vs Continuous Deployment
Understanding the distinction between Continuous Delivery and Continuous Deployment is essential for defining your pipeline’s scope and automation level. Continuous Delivery ensures that code is always production-ready, automating the build, test, and pre-release processes while leaving deployment as a manual decision. This approach is ideal for teams needing to navigate app store reviews or require controlled releases with specific timelines.
On the other hand, Continuous Deployment eliminates manual intervention, pushing verified changes directly into production. This model suits internal apps or web platforms that benefit from immediate updates. However, it requires very strong testing strategies to minimize the risk of deploying bugs into production. For Flutter projects that target app stores, Continuous Delivery is often the optimal balance between automation and control.
Choosing the Right CI/CD Tools for Flutter
Selecting the right CI/CD tools for your Flutter development workflow is an important decision that directly affects your project’s efficiency, scalability, and deployment pipeline. This section of the article aims to explore some powerful CI/CD tools for Fluter including their unique strengths, configurations, and considerations to help you make an informed choice.
1. Jenkins
Jenkins is one of the most established CI/CD tools that offers unmatched flexibility and customizability. Its open-source nature gives development teams complete control over their build and deployment processes. With a massive library of plugins and integrations, Jenkins adapts to diverse project needs making it ideal for larger teams or organizations with complex workflows. Teams can tailor workflows to specific requirements, enjoy the benefits of self-hosting for complete infrastructure control, and leverage an extensive plugin ecosystem to extend functionality and integrate with several tools.
2. GitHub Actions
GitHub Actions has been gaining significant momentum in the Flutter community due to its seamless integration with GitHub repositories and straightforward configuration process. As a cloud-based solution, GitHub Actions eliminates the need for server management while still offering strong automating capabilities which makes it an attractive choice for teams looking for a more streamlined approach to CI/CD implementation.
3. CircleCI
CircleCI is another powerful cloud-based solution that offers fast, scalable, and reliable contained build environments. Flutter developers benefit from CircleCI’s ability to run cross-platform builds with ease to ensure consistent results across multiple pipelines.
4. Specialized Mobile CI/CD Platforms: Bitrise and Codemagic
While general-purpose CI/CD tools offer broad capabilities, specialized platforms like Bitrise and Codemagic cater specifically to mobile development, making them well-suited for Flutter projects.
Bitrise simplifies mobile app development workflows with pre-configured steps tailored for Flutter. Its caching significantly reduces build times while its user-friendly interface streamlines complex tasks like managing iOS certificates and Android signing configurations.
On the other hand, Codemagic is designed exclusively for Flutter and Dart projects. It automatically detects Flutter project configurations, making setup effortless. Features like automatic code signing, app store publishing, and detailed build analytics make Codemagic a standout choice. To read more about Codemagic, check out our insight on the platform here.
Specialized platforms offer several compelling advantages for development teams. They stand out for their ease of use, requiring minimal setup time by leveraging their pre-configured workflows that get teams up and running quickly. These platforms are specifically optimized for mobile development by handling platform-specific requirements without the need for complex configurations. Additionally, they come equipped with advanced features like code signing, artifact management, and direct publishing capabilities that streamline the entire development process.
When selecting the best CI/CD tool for your Flutter project, several key factors come into play. Team size and skill level significantly influence the choice. While large, experienced teams might leverage Jenkins’ extensive customizability, smaller teams often find the simplicity of Codemagic or GitHub Actions more appealing. Infrastructure requirements also play an important role as organizations requiring strict control over their infrastructure typically lean towards Jenkins or self-hosted CircleCI, while teams prioritizing ease of maintenance often choose cloud-based solutions like GitHub Actions, Bitrise, or Codemagic.
Likewise, cost considerations need to be considered in the decision-making process. GitHub Actions provides an attractive free tier that works well for open-source projects whereas premium tools like Bitrise and Codemagic may require additional budget allocation. Integration requirements shape the final choice - teams deeply integrated into the GitHub ecosystem naturally find GitHub Actions to be an ideal fit, while those needing complex, multi-tool integrations may find Jenkins or CircleCI more suitable.
By thoroughly assessing your team’s specific needs, project scale, and available budget, you can identify the CI/CD tool that will best enhance your Flutter development pipeline and drive your project’s success.
Challenges in Implementing CI/CD For Flutter Projects
While the benefits of implementing CI/CD pipelines for Flutter applications are numerous, the process of setting up and maintaining these pipelines is not without its challenges. Flutter, as a cross-platform framework, introduces unique complexities that developers and teams must address to ensure a seamless CI/CD workflow. Understanding these challenges and their solutions is essential to building a strong and reliable pipeline.
1. Platform-Specific Issues
Challenge: One of the most significant challenges in Flutter CI/CD is managing platform-specific build requirements. For instance, building iOS applications requires a macOS environment along with provisioning profiles, certificates, and the configuration of a signing process. Teams without access to macOS systems may find this process daunting especially when integrating these steps into an automated pipeline. On the Android side, developers must handle keystore management and configure specific build settings for release versions which can become cumbersome when targeting multiple release channels.
Solution: Leveraging specialized tools like Codemagic or Bitrise can simplify platform-specific build processes by offering pre-configured environments tailored to Flutter. These tools handle tasks such as macOS provisioning and Android Keystore management, reducing the complexity for developers.
2. Dependency Management
Challenge: Flutter projects often rely heavily on third-party libraries and plugins, which must be compatible with the Flutter SDK and native platforms like Android and iOS. Managing these dependencies within a CI/CD pipeline can become challenging when updates introduce breaking changes. Additionally, keeping track of native dependencies for platform-specific features further complicates the process. Without proper tools or strategies, these issues can lead to build failures or runtime errors.
Solution: Using tools like flutter pub outdated within the pipeline ensures that dependencies are up-to-date. Regular audits of dependency compatibility and proactive testing with new Flutter SDK releases can mitigate breaking changes.
3. Testing Complexity
Challenge: Testing is another area where issues arise. Flutter’s cross-platform capabilities mean that testing must be conducted across multiple device types, operating systems, screen sizes, and resolutions. Ensuring adequate test coverage across all these dimensions requires a well-structured testing strategy but running such extensive test suites within a CI/CD pipeline can be resource-intensive and time-consuming. Additionally, debugging failures in automated tests, especially for platform-specific features can slow down the development process.
Solution: Optimizing test execution by running tests in parallel or focusing on incremental builds can reduce pipeline execution time. Cloud-based testing solutions like Firebase Test Lab can streamline testing across devices without requiring extensive local infrastructure.
4. Resource Constraints
Challenge: Smaller teams or open-source contributors often face resource limitations when setting up CI/CD pipelines. While cloud-based CI/CD solutions offer scalability and flexibility, they may also introduce costs that are prohibitive for some teams. Moreover, maintaining on-premises CI/CD infrastructure can demand expertise and time that small teams might lack.
Solution: For small teams, free or lower-cost CI/CD solutions like GitHub Actions’ free tier or self-hosted Jenkins can be viable options. These tools allow teams to build efficiency pipelines without significant upfront investment.
5. Managing Environment Configurations
Challenge: Flutter apps often require different configurations for various environments such as development, staging, and production. Managing these environment-specific variables securely and consistently across multiple platforms within a CI/CD pipeline can become complex. Without proper practice, configuration mismatches can lead to unexpected runtime behavior.
Solution: Tools like GitHub Secrets, Bitrise Env Vars, or third-party solutions like Vault can help teams maintain consistency and security across all stages of the pipeline.
By proactively addressing these challenges, teams can unlock the full potential of CI/CD for Flutter projects, enabling the full potential of CI/CD for Flutter projects to enable faster delivery cycles, enhanced code quality, and reliable deployments across multiple platforms.
Best Practices for CI/CD in Flutter Development
Implementing a CI/CD pipeline for Flutter applications can transform the development and deployment process, but to truly maximize its potential, teams must follow best practices. These practices ensure that pipelines are efficient, secure, and capable of supporting the complexities of cross-platform Flutter development. This section of the article covers some best practices for setting up CI/CD pipelines.
1. Designing Modular and Maintainable Pipelines
A well-structured CI/CD pipeline is modular, making it easy to debug, maintain, and update. Teams should define distinct stages for source control, build, test, and deployment, ensuring that each phase of the pipeline is independent and can be modified without affecting others. For example, separating build processes for iOS and Android allows for parallel execution and reduces the overall pipeline runtime.
Additionally, using configurations as code (e.g., YAML files in Github Actions or Jenkinsfiles in Jenkins) promotes pipeline reproducibility and version control. This approach enables teams to easily replicate or roll back pipelines when needed.
2. Optimizing Build Performance
Flutter apps can have lengthy build processes especially when targeting multiple platforms. Implementing caching mechanisms is essential to speed up these processes. For example, caching Flutter SDKs, dependencies, and generated assets ensures that these resources do not need to be downloaded or rebuilt every time the pipeline runs.
Incremental builds can also help optimize your build performance. By only rebuilding parts of the project that are affected by recent code changes, pipelines can significantly reduce build time. Tools and CI/CD platforms that support incremental builds are particularly valuable in large projects.
3. Ensuring Code Quality Through Automation
Integrating static code analysis with tools like flutter analyze or dart analyze, along with spell-checking can help enforce coding standards and detect issues early. Automated linting ensures consistency across the codebase which makes it easier for teams to collaborate effectively.
In addition, automated testing should be a mandatory step in every CI/CD pipeline. Running unit, widget, and integrating tests ensure that new code does not introduce regressions or break existing functionality. High test coverage, enforced as a pipeline threshold, can serve as a safeguard against poorly tested code being merged into the main branch.
4. Securely Managing Secrets and Environment Variables
Secrets such as API keys, signing certificates, and environment-specific configurations must be handled with the utmost care in CI/CD pipelines. Using secure storage solutions like Github Secrets is essential. Access to these secrets should be tightly controlled and limited to specific pipeline stages that require them.
For flutter projects targeting multiple environments, defining a standardized approach for managing environment variables (e.g., using .env files for platform-specific configuration files) ensures consistency and prevents errors caused by mismatched settings.
5. Monitoring and Observability
CI/CD pipelines should include mechanisms to monitor their performance and detect issues in real-time. Metrics such as build time, test success rates, and failure trends provide valuable insights into the pipeline’s health and efficiency. By tracking these metrics, teams can identify bottlenecks or flaky tests and address them proactively.
6. Continuous Feedback Loops
CI/CD pipelines should facilitate continuous feedback to allow developers to quickly respond to issues. Feedback loops can be enhanced by sending pipeline results directly to communication channels like Slack to ensure stakeholders are promptly notified of failures or performance issues.
7. Documenting and Standardizing Processes
Documentation is an often overlooked best practice in CI/CD implementation. Clear, comprehensive documentation of the pipeline’s structure, configurations, and troubleshooting steps ensures that all team members can understand and contribute to the pipeline’s maintenance and improvement. Standardizing processes for tasks like branching, merging, and testing encourages team collaboration and reduces the likelihood of errors.
By following these best practices, teams can ensure that their CI/CD pipelines for Flutter are not only functional but also efficient, secure, and scalable.
The Future of CI/CD in Flutter Development
As the demand for Flutter applications continues to grow, CI/CD pipelines are poised to evolve by integrating emerging technologies and addressing new challenges in software development. The future of CI/CD in Flutter development will be shaped by trends that enhance automation, optimize workflows, and deepened integrations with other aspects of software engineering. These advancements will empower developers to build, test, and deploy applications with unprecedented efficiency and reliability.
1. Serverless CI/CD Pipelines
The rise of serverless computing is set to redefine CI/CD pipelines. Serverless CI/CD platforms eliminate the need for managing infrastructure or providing on-demand build environments that scale automatically with workload requirements. This model is particularly important for Flutter teams as it reduces the overhead of maintaining build servers while ensuring rapid and cost-effective processing for multi-platform builds.
Flutter developers can expect serverless platforms to offer pre-configured environments tailored for Flutter SDK versions, reducing setup time and eliminating inconsistencies. Additionally, serverless CI/CD allows teams to experiment with complex workflows without worrying about resource constraints, enabling more dynamic and strong pipelines.
2. AI-Driven CI/CD Optimization
Artificial intelligence and machine learning are increasingly finding applications in CI/CD pipelines, and this trend is expected to expand. AI tools can predict pipeline performance, detect patterns in build failures, and recommend improvements.
In the context of Flutter projects, AI could enhance development in several ways. First, it can analyze build logs to identify common causes of failures and suggest fixes. Additionally, AI can dynamically prioritize tests based on code changes, which helps reduce overall execution time. It can also optimize resource allocation for parallel builds, ensuring efficient use of cloud environments.
This AI-driven approach to CI/CD will not only improve efficiency but also reduce the cognitive load on development teams, enabling them to focus on building innovative features.
3. Deeper Integration with DevSecOps
As security becomes a top priority for software development, CI/CD pipelines will increasingly integrate with DevSecOps practices. Future pipelines for Flutter applications will seamlessly incorporate automated security checks.
These automated security measures could include scanning Flutter dependencies for vulnerabilities, verifying API security through automated tests, and enforcing compliance with security policies at every stage of the pipeline.
These integrations will help ensure that Flutter apps are not only functional and high-performing but also secure against emerging threats.
4. Low-Code and No-Code CI/CD Solutions
As CI/CD adoption grows across teams with varying levels of expertise, low-code, and no-code solutions are expected to gain popularity. These platforms will allow developers to set up pipelines using visual interfaces, reducing the need for manual scripting and configuration. For Flutter projects, such solutions will streamline pipeline creation, enabling teams to focus on application development rather than operational complexities.
Conclusion
In conclusion, the implementation of robust CI/CD pipelines has become a cornerstone of modern software development. Flutter’s ability to target multiple platforms from a single codebase offers numerous benefits but also introduces unique challenges in the build, test, and deployment processes. CI/CD pipelines bridge the gap between Flutter’s powerful cross-platform capabilities and the need for consistency, speed, and reliability in software delivery.
Authors
Streamline Your Flutter Development with Expert CI/CD Solutions
Take your Flutter projects to the next level with Walturn’s expertise in CI/CD implementation. We help you automate builds, testing, and deployments to ensure consistent quality across all platforms. From managing dependencies to optimizing release workflows, our tailored solutions enhance efficiency and reliability in your development process.
References
CircleCI. “Continuous Integration and Delivery.” CircleCI, circleci.com.
“Codemagic - CI/CD for Android, iOS, Flutter and React Native Projects.” Codemagic, codemagic.io/start.
Continuous Integration and Delivery (CI/CD) Platform | Bitrise. bitrise.io.
“GitHub Actions.” GitHub, 2024, github.com/features/actions.
“Jenkins.” Jenkins, www.jenkins.io.
Thatikonda, Vamsi Krishna. “Beyond the Buzz: A Journey Through CI/CD Principles and Best Practices.” European Journal of Theoretical and Applied Sciences, vol. 1, no. 5, Sept. 2023, pp. 334–40. https://doi.org/10.59324/ejtas.2023.1(5).24.
Walturn’s Best Practices for Backend Development - Walturn Insight. www.walturn.com/insights/walturn-s-best-practices-for-backend-development.